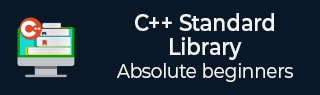
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ Forward_list::cend() 函数
C++ 的std::forward_list::cend()函数用于检索指向forward_list容器中最后一个元素之后元素的常量迭代器。
这个forward_list的元素充当占位符;尝试访问它会导致未定义的行为。在C++中,迭代器是一个可以迭代STL(标准模板库)容器中的元素并提供对单个元素访问的对象。迭代器的主要优点是为所有容器类型提供了一个公共接口。
语法
以下是C++ std::forward_list::cend()函数的语法:
const_iterator cend() const;
参数
- 它不接受任何参数。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
返回值
此函数返回指向最后一个元素之后元素的常量迭代器。
示例 1
如果forward_list是int类型,则cend()函数返回指向容器中最后一个元素之后元素的常量迭代器。
在下面的程序中,我们使用C++ std::forward_list::cend()函数来检索指向当前forward_list {1, 2, 3, 4, 5}的最后一个元素之后元素的常量迭代器。
#include<iostream> #include<forward_list> using namespace std; int main() { //create a forward_list forward_list<int> num_list = {1, 2, 3, 4, 5}; cout<<"The num_list contents are: "<<endl; for(int n : num_list){ cout<<n<<endl; } //using the cend() function auto it = num_list.cend(); cout<<"The constant iterator following the last element is: "<<*it; return 0; }
输出
让我们编译并运行上面的程序,这将产生以下结果:
The num_list contents are: 1 2 3 4 5 Segmentation fault
示例 2
使用for循环以及cend()函数来检索容器中所有最后一个元素之后元素的常量迭代器。
以下是C++ std::forward_list::cend()函数的另一个示例。在这里,我们创建一个名为char_list的forward_list(类型为char),其内容为{'a', 'b', 'c', 'd', 'e'}。然后,我们使用for循环以及cend()函数遍历容器,并检索此forward_list中所有最后一个元素之后元素的常量迭代器。
#include<iostream> #include<forward_list> using namespace std; int main() { //create a forward_list forward_list<char> char_list = {'a', 'b', 'c', 'd', 'e'}; int size = distance(char_list.begin(), char_list.end()); cout<<"The size of the char_list forward_list is: "<<size<<endl; //using cend() function cout<<"The constant iterator of all the elements are: "<<endl; for(auto it = char_list.begin(); it != char_list.cend(); it++){ cout<<*it<<endl; } }
输出
以下是上述程序的输出:
The size of the char_list forward_list is: 5 The constant iterator of all the elements are: a b c d e
示例 3
除了int类型和char类型的forward_list元素外,我们还可以检索string类型forward_list元素的迭代器。
在这个程序中,我们创建一个名为languages的forward_list(类型为string),其内容为{"HTML", "CSS", "JavaScript", "C++", "Java"}。然后,我们使用for循环以及cend()函数遍历容器,并检索此forward_list中所有最后一个元素之后元素的常量迭代器。
#include<iostream> #include<forward_list> using namespace std; int main() { //create a forward_list forward_list<string> languages = {"HTML", "CSS", "JavaScript", "C++", "Java"}; int size = distance(languages.begin(), languages.end()); cout<<"The size of the vowels forward_list is: "<<size<<endl; //using cend() function cout<<"The constant iterators of an elements are: "<<endl; for(auto it = languages.begin(); it != languages.cend(); it++){ cout<<*it<<endl; } }
输出
这将生成以下输出:
The size of the vowels forward_list is: 5 The constant iterators of an elements are: HTML CSS JavaScript C++ Java