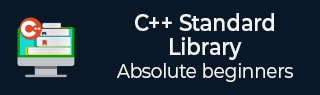
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ forward_list::clear() 函数
C++ 的std::forward_list::clear()函数用于擦除 forward_list 中的所有元素。
它通过从 forward_list 中删除所有元素来销毁 forward_list,并将 forward_list 的大小设置为零。此函数的返回类型为 void,这意味着它不返回值。
语法
以下是 C++ std::forward_list::clear() 函数的语法:
void clear();
参数
- 它不接受任何参数。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
返回值
此函数不返回值。
示例 1
如果 forward_list 是 int 类型,则 clear() 函数会擦除 forward_list 中的所有元素。
在以下程序中,我们使用 C++ std::forward_list::clear() 函数来擦除当前 forward_list {10, 20, 30, 40, 50} 中的所有元素,并且此函数调用后 forward_list 的大小将为零。
#include<iostream> #include<forward_list> using namespace std; int main(){ //create a forward_list forward_list<int> num_list = {10, 20, 30, 40, 50}; cout<<"The forward_list contents before the clear operation: "<<endl; for(int n : num_list) { cout<<n<<endl; } cout<<"The size of the forward_list before clear operation: "; int size = distance(num_list.begin(), num_list.end()); cout<<size<<endl; //using the clear() function num_list.clear(); int new_size = distance(num_list.begin(), num_list.end()); cout<<"The size of forward_list after clear operation: "<<new_size; }
输出
以下是上述程序的输出:
The forward_list contents before the clear operation: 10 20 30 40 50 The size of the forward_list before clear operation: 5 The size of forward_list after clear operation: 0
示例 2
如果 forward_list 是 char 类型,则此函数会擦除 forward_list 中的所有元素。
以下是 C++ std::forward_list::clear() 函数的另一个示例。在这里,我们创建一个名为 char_list 的 forward_list(类型为 char),其内容为 {'A', 'B', 'C', 'D', 'E'}。然后,使用 clear() 函数,我们尝试擦除此 forward_list 中的所有元素。
#include<iostream> #include<forward_list> using namespace std; int main(){ //create a forward_list forward_list<int> char_list = {'A', 'B', 'C', 'D', 'E'}; cout<<"The forward_list contents before the clear operation: "<<endl; for(char c : char_list) { cout<<c<<endl; } cout<<"The size of the forward_list before clear operation: "; int size = distance(char_list.begin(), char_list.end()); cout<<size<<endl; //using the clear() function char_list.clear(); int new_size = distance(char_list.begin(), char_list.end()); cout<<"The size of forward_list after clear operation: "<<new_size; }
输出
这将生成以下输出:
The forward_list contents before the clear operation: A B C D E The size of the forward_list before clear operation: 5 The size of forward_list after clear operation: 0
示例 3
除了 int 类型和 char 类型 forward_list 元素之外,我们还可以擦除 string 类型 forward_list 元素。
在此示例中,我们创建一个名为 languages 的 forward_list(类型为 string),其内容为 {"Java", "HTML", "CSS", "JavaScript"}。使用 clear() 函数,我们尝试擦除此 forward_list 中的所有元素。
#include<iostream> #include<forward_list> using namespace std; int main(){ //create a forward_list forward_list<string> languages = {"Java", "HTML", "CSS", "JavaScript"}; cout<<"The forward_list contents before the clear operation: "<<endl; for(string l : languages) { cout<<l<<endl; } cout<<"The size of the forward_list before clear operation: "; int size = distance(languages.begin(), languages.end()); cout<<size<<endl; //using the clear() function languages.clear(); int new_size = distance(languages.begin(), languages.end()); if(new_size == 0) { cout<<"The forward_list has no elements and size is: "<<new_size; }else{ cout<<"The forward_list is non_empty and size is: "<<new_size; } }
输出
上述程序产生以下输出:
The forward_list contents before the clear operation: Java HTML CSS JavaScript The size of the forward_list before clear operation: 4 The forward_list has no elements and size is: 0