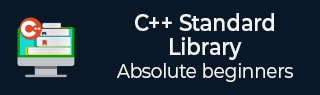
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ forward_list::emplace_after() 函数
C++ 的std::forward_list::emplace_after()函数用于在forward_list容器中指定位置之后插入(或附加)一个新元素。
它返回一个指向新插入元素的迭代器,并将forward_list的大小增加一。在C++中,迭代器是一个可以遍历STL(标准模板库)容器中的元素并访问单个元素的对象。
我们可以使用emplace_after()函数以及for循环在forward_list容器中指定位置之后动态插入元素。
语法
以下是C++ std::forward_list::emplace_after()函数的语法:
iterator emplace_after (val, pos);
参数
- val - 要插入到forward_list中的新元素的值。
- position - forward_list中新元素要插入到的位置。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
返回值
此函数返回一个指向新插入元素的迭代器。
示例1
在开头位置之后插入一个元素。
在下面的程序中,我们使用C++ std::forward_list::emplace_after()函数在当前forward_list {10, 30, 40, 50}的开头位置之后插入一个新的指定元素20。
#include<iostream> #include<forward_list> using namespace std; int main() { //create a forward_list forward_list<int> num_list = {10, 30, 40, 50}; cout<<"The num_list contents before the emplace_after operation: "<<endl; for(int n : num_list){ cout<<n<<endl; } //value of element and pos int val = 20; auto pos = num_list.begin(); //using the emplace_after() function num_list.emplace_after(pos, val); cout<<"The num_list contents after the emplace_after operation: "<<endl; for(int n : num_list){ cout<<n<<endl; } }
输出
以下是上述程序的输出:
The num_list contents before the emplace_after operation: 10 30 40 50 The num_list contents after the emplace_after operation: 10 20 30 40 50
示例2
如果forward_list是字符类型,则此函数会在指定位置之后插入新的指定元素。
以下是C++ std::forward_list::emplace_after()函数的另一个示例。在这里,我们创建一个名为char_list的forward_list(字符类型),其内容为{'B', 'C', 'D', 'E', 'F'}。然后,使用emplace_after()函数,我们尝试在此forward_list的指定位置之后插入一个新的指定元素'A'。
#include<iostream> #include<forward_list> using namespace std; int main() { //create a forward_list forward_list<char> char_list = {'B', 'C', 'D', 'E', 'F'}; cout<<"The char_list contents before the emplace_after operation: "<<endl; for(char c : char_list){ cout<<c<<endl; } //value of element and pos char val = 'A'; auto pos = char_list.before_begin(); //using the emplace_after() function char_list.emplace_after(pos, val); cout<<"The char_list contents after the emplace_after operation: "<<endl; for(char c : char_list){ cout<<c<<endl; } }
输出
这将生成以下输出:
The char_list contents before the emplace_after operation: B C D E F The char_list contents after the emplace_after operation: A B C D E F
示例3
除了int类型和char类型元素外,我们还可以将字符串类型元素插入到指定位置之后。
在这个例子中,我们创建一个名为names的forward_list(字符串类型),其内容为{"Aman", "Abhishek", "Ganesh", "Mohit"}。然后,使用emplace_after()函数,我们尝试在当前forward_list的开头位置之后插入一个新的指定元素"Raju"。
#include<iostream> #include<forward_list> using namespace std; int main() { //create a forward_list forward_list<string> names = {"Aman", "Abhishek", "Ganesh", "Mohit"}; cout<<"The names forward_list contents before the emplace_after operation: "<<endl; for(string s: names){ cout<<s<<endl; } //value of element and pos string val = "Raju"; auto pos = names.begin(); //using the emplace_after() function names.emplace_after(pos, val); cout<<"The names forward_list contents after the emplace_after operation: "<<endl; for(string s : names){ cout<<s<<endl; } }
输出
上述程序产生以下输出:
The names forward_list contents before the emplace_after operation: Aman Abhishek Ganesh Mohit The names forward_list contents after the emplace_after operation: Aman Raju Abhishek Ganesh Mohit
示例4
使用emplace_after()函数以及for循环在当前forward_list的指定位置之后动态插入元素。
#include<iostream> #include<forward_list> using namespace std; int main() { //create a forward_list forward_list<int> num_list = {1}; cout<<"The size of the num_list before the emplace_after operation: "<<endl; int size = distance(num_list.begin(), num_list.end()); cout<<size<<endl; //pos auto pos = num_list.begin(); for(int i = 10; i>1; i--){ num_list.emplace_after(pos, i); } cout<<"The num_list contents after the emplace_after operation: "<<endl; for(int n : num_list){ cout<<n<<endl; } }
输出
让我们编译并运行上述程序,这将产生以下结果:
The size of the num_list before the emplace_after operation: 1 The num_list contents after the emplace_after operation: 1 2 3 4 5 6 7 8 9 10