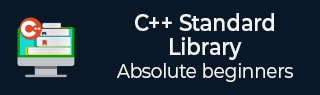
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ List::clear() 函数
C++ 的std::list::clear()函数用于从列表(或容器)中删除所有元素。
当调用此函数时,size() 函数会将列表大小返回为零。此函数的返回类型为 void,这意味着它不返回值。
语法
以下是 C++ std::list::clear() 函数的语法:
void clear();
参数
- 它不接受任何参数。
返回值
此函数不返回任何值。
示例 1
在下面的程序中,我们使用 C++ std::list::clear() 函数来清空当前列表 {10, 20, 30, 40, 50}。
#include<iostream> #include<list> using namespace std; int main(void) { //create an integer list list<int> lst = {10, 20, 30, 40, 50}; cout<<"The list elements are: "; for(int l: lst) { cout<<l<<" "; } cout<<"\nInitial size of list: "<<lst.size(); //use clear() method lst.clear(); cout<<"\nAfter perform the clear() function the list size is: "<<lst.size(); }
输出
以上程序输出如下:
The list elements are: 10 20 30 40 50 Initial size of list: 5 After perform the clear() function the list size is: 0
示例 2
以下是 C++ std::list::clear() 函数的另一个示例,在这里,我们创建一个值为 {'+', '@', '#', '$','%'} 的列表(类型为 char),并使用此函数尝试清空此列表。
#include<iostream> #include<list> using namespace std; int main(void) { //create char type list list<char> lst = {'+', '@', '#', '$','%'}; cout<<"The list elements are: "; for(char l: lst) { cout<<l<<" "; } cout<<"\nInitial size of list: "<<lst.size(); //use clear() method lst.clear(); cout<<"\nAfter perform the clear() function the list size is: "<<lst.size(); }
输出
以下是以上程序的输出:
The list elements are: + @ # $ % Initial size of list: 5 After perform the clear() function the list size is: 0
示例 3
如果当前列表是字符串类型。
在此程序中,我们创建一个值为 {'Java', 'HTML', 'CSS', 'Angular'} 的列表(类型为字符串)。然后,使用 C++ std::list::clear() 函数尝试清空此列表。
#include<iostream> #include<list> using namespace std; int main(void) { list<string> lst = {"Java", "HTML", "CSS", "Angular"}; cout<<"The list elements are: "; for(string l: lst) { cout<<l<<" "; } cout<<"\nInitial size of list: "<<lst.size(); //use clear() method lst.clear(); cout<<"\nAfter perform the clear() function the list size is: "<<lst.size(); }
输出
执行以上程序后,将输出以下内容:
The list elements are: Java HTML CSS Angular Initial size of list: 4 After perform the clear() function the list size is: 0
广告