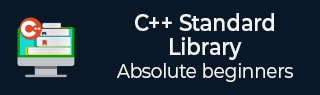
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ multimap::count() 函数
C++ 的 std::multimap::count() 函数用于返回与指定键匹配的元素数量。与每个键都唯一的 std::map 不同,std::multimap 允许具有相同键的多个元素。它操作的是一个排序集合,其中每个键可以与多个值关联。使用此函数,我们可以确定某个键在 multimap 中出现的次数。此函数的时间复杂度是对数级的,即 O(log n)。
语法
以下是 std::multimap::count() 函数的语法。
size_type count (const key_type& k) const;
参数
- k − 表示要搜索的键。
返回值
此函数返回与键关联的值的数量。
示例
在下面的示例中,我们将演示 count() 函数的基本用法。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{1, "Sail"}, {2, "Audi"}, {1, "Beat"}, {1, "Cruze"}}; int x = 1; int y = a.count(x); std::cout << "Occurrences of key " << x << ": " << y << std::endl; return 0; }
输出
以上代码的输出如下:
Occurrences of key 1: 3
示例
考虑下面的示例,我们将获取不存在的键的计数。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{1, "Hi"}, {2, "Hello"},{3, "Namaste"}}; int x = 5; int y = a.count(x); std::cout << "Occurrences of key " << x << ": " << y << std::endl; return 0; }
输出
以上代码的输出如下:
Occurrences of key 5: 0
示例
让我们看下面的示例,我们将对空的 multimap 应用 count 并观察输出。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> x; int a = 2; int b = x.count(a); std::cout << "Occurrences of key " << a << ": " << b << std::endl; return 0; }
输出
如果我们运行以上代码,它将生成以下输出:
Occurrences of key 2: 0
示例
下面的示例中,我们将 count() 函数用于循环中。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{1, "Hi"}, {1, "Hello"}, {3, "Welcome"}}; for (int x = 1; x <= 2; ++x) { int y = a.count(x); std::cout << "Occurrences of key " << x << " : " << y << std::endl; } return 0; }
输出
让我们编译并运行上述程序,这将产生以下结果:
Occurrences of key 1 : 2 Occurrences of key 2 : 0
multimap.htm
广告