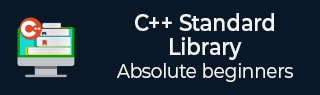
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ multimap::crend() 函数
C++ 的 std::multimap::crend() 函数用于返回一个指向 multimap 中第一个元素之前元素的常量反向迭代器,标志着它反向顺序的结尾。此迭代器不能用于修改它指向的元素。它用于反向迭代 multimap 而无需更改元素,从而确保只读访问。此函数的时间复杂度是常数,即 O(1)。
语法
以下是 std::multimap::crend() 函数的语法。
const_reverse_iterator crend() const noexcept;
参数
它不接受任何参数。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
返回值
此函数返回一个常量反向迭代器。
示例
在下面的示例中,我们将演示 crend() 函数的用法。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{2, "B"}, {1, "C"}, {3, "A"}}; for (auto x = a.crbegin(); x != a.crend(); ++x) { std::cout << x->first << ": " << x->second << std::endl; } return 0; }
输出
以下是上述代码的输出:
3: A 2: B 1: C
示例
考虑以下示例,我们将检查 multimap 是否为空。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; if (a.crbegin() == a.crend()) { std::cout << "Multimap is empty." << std::endl; } else { std::cout << "Multimap is not empty." << std::endl; } return 0; }
输出
上述代码的输出如下:
Multimap is empty.
示例
在下面的示例中,我们将查找 multimap 中最大的键。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{4, "A"}, {3, "B"}, {1, "C"}}; auto x = a.crbegin(); if (x != a.crend()) { std::cout << "" << x->first << std::endl; } return 0; }
输出
如果我们运行上述代码,它将生成以下输出:
4
示例
以下示例将计算 multimap 中值为“B”的元素个数。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{3, "A"}, {1, "B"}, {1, "B"}, {1, "B"}}; int x = 0; for (auto y = a.crbegin(); y != a.crend(); ++y) { if (y->second == "B") { ++x; } } std::cout << " " << x << std::endl; return 0; }
输出
让我们编译并运行上述程序,这将产生以下结果:
3
multimap.htm
广告