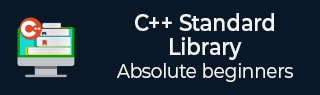
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ multimap::emplace() 函数
C++ 的 std::multimap::emplace() 函数用于将新的键值对插入容器中。与 insert() 函数不同,insert() 函数复制或移动元素,而 emplace() 函数通过消除不必要的复制来就地构造元素。它直接将参数转发到元素的构造函数。由于 multimap 允许具有相同键的多个元素,因此 emplace() 在插入元素时特别有用,无需创建和管理临时对。
语法
以下是 std::multimap::emplace() 函数的语法。
iterator emplace (Args&&... args);
参数
- args − 转发到元素构造函数的参数。
返回值
此函数返回指向新插入元素的迭代器。
示例
让我们看下面的例子,我们将演示 emplace() 函数的使用。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; a.emplace(1, "TP"); a.emplace(2, "TutorialsPoint"); for (const auto& x : a) { std::cout << x.first << ": " << x.second << std::endl; } return 0; }
输出
以上代码的输出如下:
1: TP 2: TutorialsPoint
示例
考虑另一种情况,我们将使用不同的键插入多个元素。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; a.emplace(1, "Hi"); a.emplace(2, "Namaste"); a.emplace(1, "Hello"); for (const auto& x : a) { std::cout << x.first << ": " << x.second << std::endl; } return 0; }
输出
如果我们运行以上代码,它将生成以下输出:
1: Hi 1: Hello 2: Namaste
示例
在下面的示例中,我们将使用移动语义将新元素插入到 multimap 中。
#include <iostream> #include <map> #include <utility> int main() { std::multimap<int, std::string> a; a.emplace(std::make_pair(1, "Hi")); a.emplace(2, std::move(std::string("Hello"))); for(const auto& pair : a) { std::cout << pair.first << ": " << pair.second << std::endl; } return 0; }
输出
以下是以上代码的输出:
1: Hi 2: Hello
multimap.htm
广告