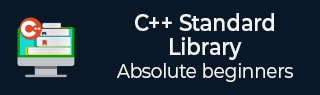
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ multimap::empty() 函数
C++ 的std::multimap::empty()函数用于检查 multimap 是否为空。如果 multimap 为空,则返回布尔值 true,否则返回 false。此函数有助于在执行进一步操作之前需要确定 multimap 是否包含任何元素的条件操作。此函数的时间复杂度为常数,即 O(1)。
语法
以下是 std::multimap::empty() 函数的语法。
bool empty() const noexcept;
参数
它不接受任何参数。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
返回值
如果 multimap 为空,则此函数返回 true,否则返回 false。
示例
让我们看下面的例子,我们将演示 empty() 函数的使用。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; if (a.empty()) { std::cout << "Multimap is empty." << std::endl; } else { std::cout << "Multimap is not empty." << std::endl; } return 0; }
输出
以上代码的输出如下:
Multimap is empty.
示例
考虑以下示例,我们将向 multimap 中添加元素并应用 empty() 函数。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; a.insert(std::make_pair(1, "Welcome")); if (a.empty()) { std::cout << "Multimap is empty." << std::endl; } else { std::cout << "Multimap is not empty." << std::endl; } return 0; }
输出
以下是以上代码的输出:
Multimap is not empty.
示例
在下面的示例中,我们将使用 empty() 函数在循环中。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; a.insert({1, "Hi"}); while (!a.empty()) { auto x = a.begin(); std::cout << "Removing the element: " << x->second << std::endl; a.erase(x); } if (a.empty()) { std::cout << "Multimap is empty now." << std::endl; } return 0; }
输出
如果我们运行以上代码,它将生成以下输出:
Removing the element: Hi Multimap is empty now.
multimap.htm
广告