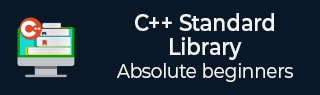
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ multimap::equal_range() 函数
C++ 的std::multimap::equal_range() 函数用于返回一对迭代器,表示具有特定键的元素范围。它允许检索与给定键匹配的所有条目。第一个迭代器指向具有该键的第一个元素,第二个迭代器指向具有该键的最后一个元素的下一位置。此函数的时间复杂度是对数的,即 O(log n)。
语法
以下是 std::multimap::equal_range() 函数的语法。
pair<const_iterator,const_iterator> equal_range (const key_type& k) const; pair<iterator,iterator>equal_range (const key_type& k);
参数
- k - 表示要搜索的键。
返回值
此函数返回一对迭代器。
示例
让我们看下面的例子,我们将演示 equal_range() 函数的基本用法。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{1, "A"}, {2, "B"}, {3, "C"}, {3, "D"}}; auto x = a.equal_range(3); std::cout << "Elements with key 3:" << std::endl; for (auto y = x.first; y != x.second; ++y) { std::cout << y->first << ": " << y->second << std::endl; } return 0; }
输出
以上代码的输出如下:
Elements with key 3: 3: C 3: D
示例
考虑下面的例子,我们将修改范围内的元素。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a; a.insert({1, "A"}); a.insert({1, "B"}); a.insert({2, "Tutorix"}); auto x = a.equal_range(1); for (auto y = x.first; y != x.second; ++y) { y->second = "TutorialsPoint"; } for (const auto& pair : a) { std::cout << pair.first << ": " << pair.second << std::endl; } return 0; }
输出
以下是以上代码的输出:
1: TutorialsPoint 1: TutorialsPoint 2: Tutorix
示例
在下面的例子中,我们将处理不存在的键。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{1, "apple"}, {2, "banana"}, {2, "blueberry"}}; auto x = a.equal_range(3); if (x.first == x.second) { std::cout << "No element found with provided key." << std::endl; } else { for (auto y = x.first; y != x.second; ++y) { std::cout << y->first << ": " << y->second << std::endl; } } return 0; }
输出
如果我们运行以上代码,它将生成以下输出:
No element found with provided key.
示例
以下示例中,我们将删除范围内的元素。
#include <iostream> #include <map> int main() { std::multimap<int, std::string> a = {{1, "Hi"}, {1, "Hello"}, {2, "Vanakam"}}; auto x = a.equal_range(1); a.erase(x.first, x.second); std::cout << "Elements after erasing :" << std::endl; for (const auto& pair : a) { std::cout << pair.first << ": " << pair.second << std::endl; } return 0; }
输出
让我们编译并运行以上程序,这将产生以下结果:
Elements after erasing : 2: Vanakam
multimap.htm
广告