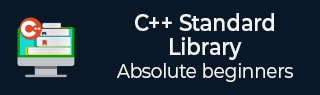
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ unordered_map::at() 函数
C++ 的unordered_map::at()函数用于检索给定项目键等效于key的映射值的引用,或者我们可以说它返回具有元素作为键“k”的值的引用。
如果项目不存在,则抛出类型为“out of range”的异常。使用此函数,我们可以访问映射值并修改它们。
语法
以下是 std::unordered_map::at() 函数的语法。
name_of_Unordered_map.at(k)
参数
k − 访问其映射值的键值。
返回值
如果对象具有常量限定符,则方法返回对映射值的常量引用;否则返回对非常量值的引用。
示例 1
让我们考虑以下示例,我们将演示 std::unordered_map::at() 函数的使用。
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_map<char, int> um = { {'a', 1}, {'b', 2}, {'c', 3}, {'d', 4}, {'e', 5} }; cout << "Value of key um['a'] = " << um.at('a') << endl; try { um.at('z'); } catch(const out_of_range &e) { cerr << "Exception at " << e.what() << endl; } return 0; }
输出
让我们编译并运行上述程序,这将产生以下结果:
Value of key um['a'] = 1 Exception at _Map_base::at
示例 2
在下面的示例中,我们将返回映射中不存在的键,并观察输出。
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_map<char, int> um = { {'a', 1}, {'b', 2}, {'c', 3}, {'d', 4}, {'e', 5} }; cout << "Value of key um['z'] = " << um.at('z') << endl; return 0; }
输出
如果我们运行上述代码,它将生成以下输出:
terminate called after throwing an instance of 'std::out_of_range' what(): _Map_base::atAborted
示例 3
考虑以下示例,我们创建一个无序映射来存储学生的成绩,并使用 at() 函数更新成绩。
#include <iostream> #include <unordered_map> using namespace std; int main() { unordered_map<string,int> stu_marks = { {"Aman", 95}, {"Vivek", 90}, {"Sarika", 85}, {"Ammu", 100} }; stu_marks.at("Aman") = 100; stu_marks.at("Vivek") += 95; stu_marks.at("Sarika") += 92; for (auto& i: stu_marks) { cout<<i.first<<": "<<i.second<<endl; } return 0; }
输出
以下是上述代码的输出:
Ammu: 100 Sarika: 177 Vivek: 185 Aman: 100
示例 4
以下示例声明一个无序映射,该映射具有整数类型键和字符类型值,并观察输出。
#include <iostream> #include <unordered_map> using namespace std; int main() { unordered_map<int,char> ASCII = { {65, 'a'}, {66, 'b'}, {67, 'c'}, {68, 'd'} }; cout << "Value of key ASCII 66 = " << ASCII.at(66) << endl; return 0; }
输出
上述代码的输出如下:
Value of key ASCII 66 = b
广告