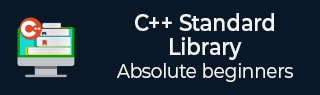
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ unordered_map::emplace_hint() 函数
C++ 函数 unordered_map::emplace_hint() 使用提示或位置在 unordered_map 中插入一个新元素。该位置仅作为提示;它不决定插入操作要执行的位置,并且通过插入新元素将容器大小扩展一个。此函数类似于 emplace() 函数,并且仅当键不存在时才进行插入,表示键应该是唯一的。
如果多次放置相同的键,则映射仅存储第一个元素,因为映射是不存储多个相同值键的容器。
语法
以下是 unordered_map::emplace_hint() 函数的语法。
unordered_map.emplace_hint(position, key, element);
参数
position - 插入元素的位置提示。容器可以使用此值来优化操作。
key - 它指定要插入到 unordered_multimap 中的键。
element - 它指定要插入到 unordered_multimap 中的元素/值。
返回值
返回指向新插入元素的迭代器。如果由于元素已存在而插入失败,则返回指向现有元素的迭代器。
示例 1
考虑以下示例,我们将在其中演示 emplace_hint() 函数的用法。
#include <iostream> #include <unordered_map> using namespace std; int main(void){ unordered_map<char, int> um ={ {'b', 2}, {'c', 3}, {'d', 4}, }; um.emplace_hint(um.end(), 'e', 5); um.emplace_hint(um.begin(), 'a', 1); cout << "Unordered map contains following elements" << endl; for (auto it = um.cbegin(); it != um.cend(); ++it) cout << it->first << " = " << it->second << endl; return 0; }
输出
以上代码的输出如下:
Unordered map contains following elements a = 1 e = 5 d = 4 b = 2 c = 3
示例 2
在以下示例中,我们将使用 emplace_hint() 函数并将键/值对添加到容器的起始位置。
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_map<string, int> um = {{"Aman", 490},{"Vivek", 485},{"Akash", 500},{"Sonam", 450}}; cout << "Unordered map contains following elements before" << endl; for (auto it = um.begin(); it != um.end(); ++it) cout << it->first << " = " << it->second << endl; cout<<"after use of the emplace_hint() function \n"; um.emplace_hint(um.begin(), "Sarika", 440); um.emplace_hint(um.begin(), "Satya", 460); cout << "Unordered map contains following elements" << endl; for (auto it = um.begin(); it != um.end(); ++it) cout << it->first << " = " << it->second << endl; return 0; }
输出
以下是以上代码的输出:
Unordered map contains following elements before Sonam = 450 Akash = 500 Vivek = 485 Aman = 490 after use of the emplace_hint() function Unordered map contains following elements Sarika = 440 Satya = 460 Sonam = 450 Akash = 500 Vivek = 485 Aman = 490
示例 3
让我们看一下以下示例,我们将在其中创建一个 unordered_map 并使用 emplace_hint() 函数以随机顺序存储键值对。
#include <iostream> #include <unordered_map> using namespace std; int main() { unordered_map<int, string> Umap; Umap.emplace_hint(Umap.begin(), 1, "January"); Umap.emplace_hint(Umap.begin(), 2, "February"); Umap.emplace_hint(Umap.begin(), 3, "March"); cout << "The unordered_map is : \n"; cout << "KEY\tELEMENT"<<endl; for (auto itr = Umap.begin(); itr != Umap.end(); itr++) cout << itr->first << "\t"<< itr->second << endl; return 0; }
输出
让我们编译并运行以上程序,这将产生以下结果:
The unordered_map is : KEY ELEMENT 3 March 2 February 1 January
广告