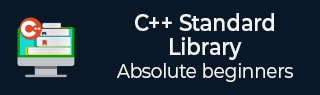
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ unordered_map::operator!= 函数
C++ 的std::unordered_map::operator!=函数用于检查两个unordered_map是否相等。如果两个unordered_map不相等,则返回true,否则返回false。
如果我们比较数据类型不同的unordered_map,则unordered_map::operator!=函数将显示错误。它仅适用于具有相同数据类型的unordered_map。
语法
以下是std::unordered_map::operator!=函数的语法。
bool operator!=(const unordered_map<Key,T,Hash,Pred,Alloc>& first, const unordered_map<Key,T,Hash,Pred,Alloc>& second);
参数
- first − 第一个unordered_map对象。
- second − 第二个unordered_map对象。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
返回值
如果两个unordered_map不相等,则此函数返回true,否则返回false。
示例 1
在下面的示例中,让我们看看operator!=函数的用法。
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_map<char, int> um1; unordered_map<char, int> um2; um1.emplace('a', 1); if (um1 != um2) cout << "Both unordered_maps are not equal" << endl; um1 = um2; if (!(um1 != um2)) cout << "Both unordered_maps are equal" << endl; return 0; }
输出
让我们编译并运行上面的程序,这将产生以下结果:
Both unordered_maps are not equal Both unordered_maps are equal
示例 2
考虑下面的示例,我们将比较两个类型相同但存储不同元素的unordered_map。
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_map<int, int> um1 = {{1, 5}, {2, 6}, {3, 7}, {4, 8}}; unordered_map<int, int> um2 = {{2, 4}, {3, 1}, {4, 2}, {5, 3}}; if (um1 != um2) cout << "Both unordered_maps are not equal" << endl; if (!(um1 != um2)) cout << "Both unordered_maps are equal" << endl; return 0; }
输出
如果我们运行上面的代码,它将生成以下输出:
Both unordered_maps are not equal
示例 3
让我们看看下面的示例,我们将比较两个unordered_map并显示元素。
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_map<string, string> um1 = {{"aman", "kumar"}, {"akash", "gupta"}, {"vivek", "verma"}, {"Rahul", "yadav"}}; unordered_map<string, string> um2 = {{"Vivek", "Verma"}, {"Alok", "Gupta"}, {"Sakshi", "Sharma"}, {"Akash", "Kumar"}}; if (um1 != um2) { cout<<"elements of um1"<<endl; for(auto & it: um1){ cout<<it.first<<" : "<<it.second<<endl; } cout<<endl; cout<<"elements of um2"<<endl; for(auto & it: um2){ cout<<it.first<<" : "<<it.second<<endl; } } if (!(um1 != um2)){ cout << " elements of both unordered_maps are equal" << endl; for(auto& it: um1){ cout<<it.first<<" : "<<it.second<<endl; } } return 0; }
输出
以下是上述代码的输出:
elements of um1 vivek : verma Rahul : yadav akash : gupta aman : kumar elements of um2 Akash : Kumar Sakshi : Sharma Alok : Gupta Vivek : Verma
示例 4
以下是一个示例,我们将使用unordered_map::operator!=函数并显示布尔值。
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_map<string, string> um1 = {{"aman", "kumar"}, {"akash", "gupta"}, {"vivek", "verma"}, {"Rahul", "yadav"}}; unordered_map<string, string> um2 = {{"Vivek", "Verma"}, {"Alok", "Gupta"}, {"Sakshi", "Sharma"}, {"Akash", "Kumar"}}; if(um1!=um2) cout<<(um1!=um2)<<endl; else cout<<(!(um1!=um2))<<endl; return 0; }
输出
上述代码的输出如下:
1
广告