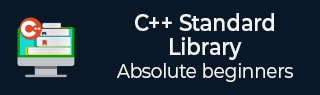
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ unordered_multimap::bucket() 函数
C++ 的std::unordered_multimap::bucket()函数用于返回包含键为k的元素所在的桶号。
桶是容器哈希表中的一个内存空间,元素根据其键的哈希值分配到该内存空间。桶的大小范围是0到bucket_count-1。
语法
以下是std::unordered_multimap::bucket()函数的语法。
size_type bucket(const Key& keyval) const;
参数
- k - 表示要查找其桶的键。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
返回值
此函数返回无符号整型,表示对应于键的桶的序号。
示例 1
在以下示例中,让我们看看unordered_multimap::bucket()函数的使用方法。
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_multimap<char, int> umm = { {'a', 1}, {'b', 2}, {'c', 3}, {'d', 4}, {'e', 5} }; cout << "Unordered multimap contains following elements" << endl; for (auto it = umm.begin(); it != umm.end(); ++it) { cout << "Element " << "[" << it->first << " : "<< it->second << "] " << "is in " << umm.bucket(it->first) << " bucket." << endl; } return 0; }
输出
让我们编译并运行上述程序,这将产生以下结果:
Unordered multi-map contains following elements Element [e : 5] is in 1 bucket. Element [d : 4] is in 0 bucket. Element [c : 3] is in 4 bucket. Element [b : 2] is in 3 bucket. Element [a : 1] is in 2 bucket.
示例 2
让我们看下面的例子,我们将创建一个存储字符串值的unordered_multimap,并应用bucket()函数来统计彼此分配的桶的数量。
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_multimap<string, string> umm = { {"Aman", "Ranchi"}, {"Vivek", "Kanpur"}, {"Akash", "Daltonganj"}, {"Aman", "Ranchi"}, {"Vivek", "Kanpur"}, }; for (auto it = umm.begin(); it != umm.end(); ++it) { cout << "Element " << "[" << it->first << " : " << it->second << "] " << "is in " << umm.bucket(it->first) << " bucket." << endl; } return 0; }
输出
如果我们运行上面的代码,它将生成以下输出:
Element [Akash : Daltonganj] is in 4 bucket. Element [Vivek : Kanpur] is in 4 bucket. Element [Vivek : Kanpur] is in 4 bucket. Element [Aman : Ranchi] is in 3 bucket. Element [Aman : Ranchi] is in 3 bucket.
示例 3
考虑以下示例,我们将显示从unordered_multimap指向容器第一个元素的迭代器的桶数。
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_multimap<string, string> umm = { {"Aman", "Ranchi"}, {"Vivek", "Kanpur"}, {"Akash", "Daltonganj"}, {"Aman", "Ranchi"}, {"Vivek", "Kanpur"}, }; // prints the bucket number of the beginning element auto it = umm.begin(); // stores the bucket number of the key k int number = umm.bucket(it->first); cout << "The bucket number of key " << it->first << " is " << number; return 0; }
输出
以下是上述代码的输出:
The bucket number of key Akash is 4
示例 4
以下是一个示例,我们将显示当前unordered_multimap中指定键的桶号。
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_multimap<char, int> umm = { {'A', 1}, {'B', 2}, {'C', 3}, {'A', 1}, {'D', 4}, {'A', 1}, {'E', 5}, {'D', 4}, }; cout<<umm.bucket('A')<<endl; cout<<umm.bucket('D')<<endl; return 0; }
输出
以上代码的输出如下:
10 2
广告