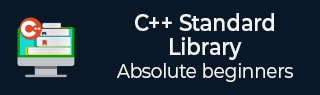
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ unordered_multimap::empty() 函数
C++ 的std::unordered_multimap::empty() 函数用于检查 unordered_multimap 是否为空。如果 unordered_multimap 为空,则返回 true;否则返回 false。此函数不会以任何方式修改或更改容器的内容。
语法
以下是 std::unordered_multimap::empty() 函数的语法。
bool empty() const noexcept;
参数
此函数不接受任何参数。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
返回值
此函数返回布尔值,即如果 unordered_multimap 为空(大小为零),则返回 true,否则返回 false。
示例 1
让我们看下面的例子,我们将演示 unordered_multimap::empty() 函数的使用。
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_multimap<char, int> umm; if (umm.empty()) cout << "Unordered multimap is empty." << endl; umm.emplace_hint(umm.begin(), 'a', 1); umm.emplace_hint(umm.end(), 'b', 2); if (!umm.empty()) cout << "Unordered multimap is not empty." << endl; return 0; }
输出
让我们编译并运行上述程序,这将产生以下结果:
Unordered multimap is empty. Unordered multimap is not empty.
示例 2
考虑下面的例子,我们将使用 empty() 函数来检查 unordered_multimap 是否包含任何元素。
#include <unordered_map> #include <iostream> #include <utility> using namespace std; int main() { unordered_multimap<int, int> numbers; cout << boolalpha; cout << "Initially, numbers.empty(): " << numbers.empty() << '\n'; numbers.emplace(55, 110); numbers.insert(make_pair(12345, 115)); cout << "After adding elements, numbers.empty(): " << numbers.empty() << '\n'; }
输出
如果我们运行上述代码,它将生成以下输出:
Initially, numbers.empty(): true After adding elements, numbers.empty(): false
示例 3
在下面的示例中,我们将使用 empty() 函数,如果容器不为空,则显示容器中的所有元素。
#include <unordered_map> #include <iostream> #include <utility> using namespace std; int main() { unordered_multimap<string, int> marks_of_students; marks_of_students.insert(make_pair("Aman", 100)); marks_of_students.insert(make_pair("Akash", 95)); marks_of_students.insert({{"Vivek", 98},{"Aman", 92},{"Akash", 97},{"Rahul", 96}}); for(auto & it: marks_of_students){ if(marks_of_students.empty()){ cout<<"marks of students are empty, I can't be able to fetch the data!"<<endl; } else{ cout<<"Marks of "<<it.first<<" is "<<it.second<<endl; } } cout<<endl; return 0; }
输出
以下是上述代码的输出:
Marks of Rahul is 96 Marks of Vivek is 98 Marks of Akash is 97 Marks of Akash is 95 Marks of Aman is 92 Marks of Aman is 100
广告