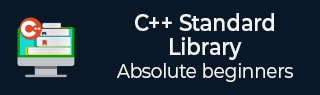
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ unordered_multimap::equal_range() 函数
C++ 的 std::unordered_multimap::equal_range() 函数用于返回一对迭代器,它们表示键与指定键等效的元素范围。
众所周知,equal_range() 函数返回一对迭代器,其中第一个迭代器指向范围内与指定键等效的第一个元素,第二个迭代器指向该范围的最后一个元素之后的位置。如果 unordered_multimap 中没有与指定键等效的元素,则返回对中的两个迭代器(下界和上界)都将等于容器末尾之后的位置,即 unordered_multimap.end()。
语法
以下是 std::unordered_multimap::equal() 函数的语法。
std::pair<iterator, iterator> equal_range(const key_type& key);
参数
- k − 表示要比较或搜索的键值。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
返回值
此函数返回一对迭代器。
示例 1
在下面的示例中,我们演示了 unordered_multimap::equal_range() 函数的用法。
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_multimap<char, int> um = { {'a', 1}, {'b', 2}, {'c', 3}, {'e', 4}, {'a', 5}, {'d', 6}, {'e', 5} }; auto ret = um.equal_range('e'); cout << "Lower bound is " << ret.first->first << " = "<< ret.first->second << endl; cout << "Upper bound is " << ret.second->first << " = " << ret.second->second << endl; return 0; }
输出
让我们编译并运行上面的程序,这将产生以下结果:
Lower bound is e = 5 Upper bound is c = 3
示例 2
考虑下面的示例,我们将访问与范围内键关联的值。
#include <iostream> #include <unordered_map> using namespace std; int main() { unordered_multimap<int, string> um = { {1, "one"}, {2, "two"}, {4, "four"}, {3, "three"}, {4, "four"}, {5, "five"}, {3, "three"}, }; auto range = um.equal_range(3); // Check if the key was found if (range.first != um.end()) { cout << "Key 3 found in the multimap!" << endl; // Access the values associated with keys in the range for (auto it = range.first; it != range.second; ++it) { cout << "The value associated with key " << it->first << " is: " << it->second << endl; } } else { cout << "Key 3 not found in the multimap!" << endl; } return 0; }
输出
如果我们运行上面的代码,它将生成以下输出:
Key 3 found in the multimap! The value associated with key 3 is: three The value associated with key 3 is: third
示例 3
让我们看一下下面的示例,我们将获取重复键的值以及指定键的上界和下界。
#include <iostream> #include <string> #include <unordered_map> using namespace std; typedef unordered_multimap<string,string> stringmultimap; int main () { stringmultimap umm = { {"orange","FL"}, {"strawberry","LA"}, {"strawberry","OK"}, {"pumpkin","NH"} }; cout << "Entries with strawberry: "<<endl; auto range = umm.equal_range("strawberry"); for (auto it = range.first; it != range.second; ++it){ cout<< it->first << " is: " << it->second<<endl; } cout << "Lower bound is " << range.first->first << " = "<< range.first->second << endl; cout << "Upper bound is " << range.second->first << " = " << range.second->second << endl; return 0; }
输出
以下是上述代码的输出:
Entries with strawberry: strawberry is: OK strawberry is: LA Lower bound is strawberry = OK Upper bound is pumpkin = NH
示例 4
以下示例将使用 multimap 并迭代指向范围的键值对。
#include <iostream> #include <unordered_map> using namespace std; int main() { unordered_multimap<int, int> umm; umm.insert({ 1, 2 }); umm.insert({ 1, 2 }); umm.insert({ 2, 3 }); umm.insert({ 3, 4 }); umm.insert({ 2, 6 }); auto range = umm.equal_range(1); cout << "Elements with Key 1: "; for (auto it = range.first; it != range.second; it++) { cout << it->second << " "; } cout << endl; range = umm.equal_range(2); cout << "Elements with Key 2: "; for (auto it = range.first; it != range.second; it++) { cout << it->second << " "; } return 0; }
输出
上述代码的输出如下:
Elements with Key 1: 2 2 Elements with Key 2: 6 3
广告