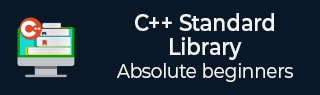
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ unordered_multimap::find() 函数
C++ 的std::unordered_multimap::find()函数用于查找与键k关联的元素。如果操作成功,则方法返回指向该元素的迭代器;否则,返回指向multimap::end()的迭代器。
语法
以下是std::unordered_multimap::find()函数的语法。
iterator find (const key_type& k); const_iterator find (const key_type& k) const;
参数
- k − 表示要搜索的键。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
返回值
如果找到指定的键,则返回指向该元素的迭代器;否则,返回multimap::end()迭代器。
示例 1
在下面的示例中,我们演示了unordered_multimap::find()函数的使用。
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_multimap<char, int> umm = { {'a', 1}, {'b', 2}, {'c', 3}, {'d', 4}, {'c', 3}, {'d', 6}, {'e', 5} }; auto it = umm.find('d'); cout << "Iterator points to " << it->first << " = " << it->second << endl; return 0; }
输出
让我们编译并运行上面的程序,这将产生以下结果:
Iterator points to d = 6
示例 2
考虑下面的示例,我们将查找值为偶数的键值对。
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_multimap<char, int> um = { {'a', 1}, {'b', 2}, {'c', 3}, {'d', 4}, {'e', 5}, {'f', 6}, }; for(auto it = um.begin(); it!=um.end(); ++it){ if(it->second % 2 == 0){ it = um.find(it->first); cout<<it->first<<" = "<<it->second<<endl; } } return 0; }
输出
如果我们运行上面的代码,它将生成以下输出:
f = 6 d = 4 b = 2
示例 3
让我们看看下面的示例,我们将创建一个接受输入字符串的输入字符串,如果输入键在multimap中可用,则返回其键值,否则返回“未找到”。
#include <iostream> #include <string> #include <unordered_map> using namespace std; int main () { unordered_multimap<string,double> umm = { {"John",55.4}, {"Vaibhaw",65.1}, {"Sunny",50.9}, {"John",60.4} }; string input; cout << "who? "; getline (cin,input); auto got = umm.find (input); if ( got == umm.end() ) cout << "not found"; else cout << got->first << " is " << got->second; return 0; }
输出
以下是输入在multimap中可用时的输出。
who? John John is 60.4
输出
以下是输入不可用时的输出:
who? Aman not found
示例 4
下面的示例演示了如何使用find()函数搜索指定键的元素。
#include <iostream> #include <string> #include <unordered_map> using namespace std; int main () { unordered_multimap<string,int> umm = { {"John",1}, {"Vaibhaw",2}, {"Sunny",3}, {"John",4} }; if (auto it = umm.find("John"); it != umm.end()) cout << "Found " << it->first << " " << it->second << '\n'; else cout << "Not found\n"; return 0; }
输出
以上代码的输出如下:
Found John 4
广告