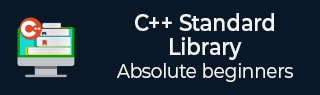
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ unordered_multimap::get_allocator() 函数
C++ 的std::unordered_multimap::get_allocator() 函数用于获取与 unordered_multimap 关联的分配器的副本。分配器负责为存储在 unordered_multimap 中的元素分配和释放内存。
此函数不会修改 unordered_multimap 并且不会抛出任何异常。
语法
以下是 std::unordered_multimap::get_allocator() 函数的语法。
allocator_type get_allocator() const;
参数
此函数不接受任何参数。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
返回值
此函数返回与 unordered_multimap 关联的分配器。
示例 1
在以下示例中,让我们看看 unordered_multimap::get_allocator() 函数的使用方法。
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_multimap<char, int> umm; pair<const char, int> *p; p = umm.get_allocator().allocate(5); cout << "Allocated size = " << sizeof(*p) * 5 << endl; return 0; }
输出
如果我们运行以上代码,它将生成以下输出:
Allocated size = 40
示例 2
让我们看看以下示例,我们将创建一个 unordered_multimap 对象和一个分配器类型对象,并将分配器与 Pair<char, int> 进行比较。
#include <iostream> #include <unordered_map> using namespace std; int main() { unordered_multimap<char, int> umm; unordered_multimap<char, int>::allocator_type u = umm.get_allocator(); cout << "Is allocator Pair<char, int> : "<< boolalpha << (u == allocator<pair<char, int> >()); return 0; }
输出
以下是以上代码的输出:
Is allocator Pair<char, int> : true
示例 3
考虑另一种情况,我们将使用 get_allocator() 函数。
#include <unordered_map> #include <iostream> using namespace std; typedef unordered_multimap<char, int> Mymap; typedef allocator<pair<const char, int> > Myalloc; int main() { Mymap obj; Mymap::allocator_type al = obj.get_allocator(); cout << "al == std::allocator() is "<< std::boolalpha << (al == Myalloc()) << std::endl; return (0); }
输出
以上代码的输出如下:
al == std::allocator() is true
广告