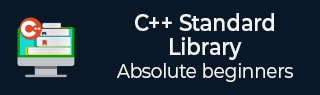
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ unordered_multimap::max_bucket_count() 函数
C++ 的std::unordered_multimap::max_bucket_count() 函数用于返回 unordered_multimap 容器根据其当前内存分配可以容纳的最大桶数。unordered_multimap 中的每个桶都是一个容器,它保存具有相同哈希值的元素。
桶是容器内部哈希表中的一个槽,元素根据其键的哈希值分配到该槽。
语法
以下是 std::unordered_multimap::max_bucket_count() 函数的语法。
size_type max_bucket_count() const;
参数
此函数不接受任何参数。
返回值
此函数返回一个无符号整数,表示最大桶数。
示例 1
让我们看下面的例子,我们将对空 multimap 使用 max_bucket_count() 函数并观察输出。
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_multimap<char, int> um; cout << "max_bucket_count of unordered_multimap = " << um.max_bucket_count() << endl; return 0; }
输出
让我们编译并运行以上程序,这将产生以下结果:
max_bucket_count of unordered_multimap = 576460752303423487
示例 2
考虑另一种情况,我们将使用 max_bucket_count 获取当前 multimap 中的最大桶数。
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_multimap<char, int> umm={{'A', 1}, {'B', 2}, {'A', 3}, {'B', 4}, {'C', 5}}; cout << "max_bucket_count of unordered_multimap = " << umm.max_bucket_count() << endl; return 0; }
输出
如果我们运行以上代码,它将生成以下输出:
max_bucket_count of unordered_multimap = 576460752303423487
示例 3
在下面的示例中,我们创建了一个存储负键值的 unordered_multimap,然后使用max_bucket_count获取最大桶数,如下所示:
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_multimap<int, char> umm={{-1, 'a'}, {-2, 'b'}, {-3, 'c'}, {-2, 'd'}, {-3, 'e'}}; cout << "max_bucket_count of unordered_multimap = " << umm.max_bucket_count() << endl; return 0; }
输出
以下是以上代码的输出:
max_bucket_count of unordered_multimap = 576460752303423487
示例 4
在下面的示例中,我们将获取在将元素插入空 multimap 之前和之后的最大桶数。
#include <iostream> #include <unordered_map> using namespace std; int main() { unordered_multimap<char, int> ummap; cout << "Size is : " << ummap.size() << endl; cout << "Max bucket count is : " << ummap.max_bucket_count() << endl; // insert elements ummap.insert({ 'a', 10 }); ummap.insert({ 'b', 12 }); ummap.insert({ 'c', 13 }); ummap.insert({ 'b', 12 }); ummap.insert({ 'd', 15 }); ummap.insert({ 'e', 20 }); cout << "Size is : " << ummap.size() << endl; cout << "Max bucket count is : " << ummap.max_bucket_count() << endl; return 0; }
输出
以上代码的输出如下:
Size is : 0 Max bucket count is : 576460752303423487 Size is : 6 Max bucket count is : 576460752303423487
广告