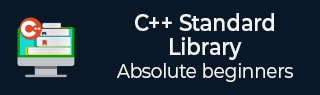
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ unordered_multimap::rehash() 函数
C++ 的std::unordered_multimap::rehash()函数用于将容器中的桶数设置为n或更多。重新哈希是哈希表的重建;根据其新的哈希值,所有元素都将重新排列到新的桶集合中。当容器的负载因子即将超过其max_load_factor时,容器会自动执行重新哈希。
如果n大于容器中当前的桶数,则会发生重新哈希;同样,如果n小于容器中当前的桶数,则该函数可能对桶计数没有影响,并且可能不会强制重新哈希。
语法
以下是std::unordered_multimap::rehash()函数的语法。
void rehash(size_type n);
参数
- n − 表示请求的桶数。
返回值
此函数不返回任何值。
示例1
在下面的示例中,让我们看看unordered_multimap::rehash()函数的用法。
#include <iostream> #include <unordered_map> using namespace std; int main (void) { unordered_multimap<char, int> umMap; cout << "Initial bucket_count: " << umMap.bucket_count() << endl; umMap.rehash(20); cout << "Current bucket_count: " << umMap.bucket_count() << endl; return 0; }
输出
如果我们运行上面的代码,它将生成以下输出:
Initial bucket_count: 1 Current bucket_count: 23
示例2
考虑下面的示例,我们将计算使用rehash()函数之前和之后的大小和桶数。
#include <iostream> #include <unordered_map> using namespace std; int main (void) { unordered_multimap<int, int> umMap={{3, 30}, {2, 20}, {3, 30}, {2, 20}, {1, 10}}; cout << "Size of container before use of rehash: "<<umMap.size() << endl; cout << "Initial bucket_count: " << umMap.bucket_count() << endl; umMap.rehash(20); cout << "Size of container after use of rehash: "<<umMap.size() << endl; cout << "Current bucket_count: " << umMap.bucket_count() << endl; return 0; }
输出
以下是上述代码的输出:
Size of container before use of rehash: 5 Initial bucket_count: 5 Size of container after use of rehash: 5 Current bucket_count: 23
示例3
让我们看看下面的示例,我们将使用rehash()函数来设置容器中的桶数。
#include <iostream> #include <unordered_map> using namespace std; int main() { unordered_multimap<int, int> umMap; umMap = { {1, 100}, {2, 20}, {3, 30}, {2, 20}, {1, 10} }; cout << "Size of container : " << umMap.size() << endl; cout << "Initial bucket count : " << umMap.bucket_count() << endl; umMap.rehash(10); cout << "Size of container : " << umMap.size() << endl; cout << "current bucket count is : " << umMap.bucket_count() << endl; for(unsigned int i = 0; i < umMap.bucket_count(); i++){ cout<<"The bucket #"<<i <<endl; } return 0; }
输出
上述代码的输出如下:
Size of container : 5 Initial bucket count : 5 Size of container : 5 current bucket count is : 11 The bucket #0 The bucket #1 The bucket #2 The bucket #3 The bucket #4 The bucket #5 The bucket #6 The bucket #7 The bucket #8 The bucket #9 The bucket #10
广告