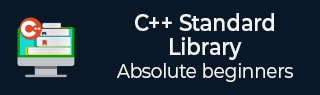
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ unordered_multimap::swap() 函数
C++ 的std::unordered_multimap::swap()函数用于交换第一个unordered_multimap与另一个unordered_multimap的内容。当另一个容器与第一个容器类型相同时,交换操作才会进行。
此函数交换元素或键/值对,而无需实际对单个元素执行任何复制或移动操作,从而无论大小都能实现常数时间执行。
语法
以下是std::unordered_multimap::swap()函数的语法。
void swap(unordered_multimap& n);
参数
- n - 表示另一个unordered_multimap对象。
返回值
此函数不返回任何值。
示例 1
让我们看下面的例子,我们将演示unordered_multimap::swap()函数的使用。
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_multimap<char, int> umm1 = { {'a', 1}, {'a', 10}, {'a', 100}, {'a', 1000}, {'a', 10000} }; unordered_multimap<char, int> umm2; umm1.swap(umm2); cout << "second unordered_multimap contains following elements after swap " << endl; for (auto it = umm2.begin(); it != umm2.end(); ++it) cout << it->first << " = " << it->second << endl; return 0; }
输出
如果我们运行以上代码,它将生成以下输出:
second unordered_multimap contains following elements after swap a = 10000 a = 1000 a = 100 a = 10 a = 1
示例 2
考虑下面的例子,我们将使用swap()函数将一个容器的元素交换到另一个容器,反之亦然。
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_multimap<char, int> umm1 = { {'a', 1}, {'b', 2}, {'c', 3}, {'b', 20}, {'c', 30}, }; unordered_multimap<char, int> umm2 = { {'e', 5}, {'f', 6}, {'g', 7}, {'h', 8} }; cout<<"umm1 contains following element before swap: "<<endl; for(auto & it:umm1){ cout<<it.first<< " = "<<it.second<<endl; } cout<<"umm2 contains following element before swap: "<<endl; for(auto & it:umm2){ cout<<it.first<< " = "<<it.second<<endl; } umm1.swap(umm2); cout << "umm1 contains following elements after swap: " << endl; for (auto it = umm1.begin(); it != umm1.end(); ++it) cout << it->first << " = " << it->second << endl; cout << "umm2 contains following elements after swap: " << endl; for (auto it = umm2.begin(); it != umm2.end(); ++it) cout << it->first << " = " << it->second << endl; return 0; }
输出
以下是以上代码的输出:
umm1 contains following element before swap: c = 30 c = 3 b = 20 b = 2 a = 1 umm2 contains following element before swap: h = 8 g = 7 f = 6 e = 5 umm1 contains following elements after swap: h = 8 g = 7 f = 6 e = 5 umm2 contains following elements after swap: c = 30 c = 3 b = 20 b = 2 a = 1
示例 3
在下面的例子中,我们将使用swap()函数仅将一个unordered_multimap中唯一的偶数键及其值交换到另一个unordered_multimap。
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_multimap<int, int> umm1, umm2, temp; umm1 = {{1, 10}, {2, 20}, {1, 100}, {2, 200}, {3, 300}, {4, 40}}; cout<<"umm1 contains following element before swap: "<<endl; for(auto& it:umm1){ cout<<it.first<<" = "<<it.second<<endl; } for(auto & it:umm1){ if(it.first%2 == 0){ temp.insert({it.first, it.second}); } } umm2.swap(temp); cout<<"umm2 contains following element before swap: "<<endl; for (auto it = umm2.begin(); it != umm2.end(); ++it) cout << it->first << " = " << it->second << endl; return 0; }
输出
以上代码的输出如下:
umm1 contains following element before swap: 4 = 40 3 = 300 2 = 200 2 = 20 1 = 100 1 = 10 umm2 contains following element before swap: 2 = 20 2 = 200 4 = 40
广告