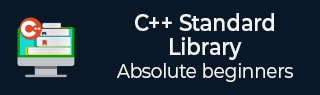
- C 标准库
- C 标准库
- C++ 标准库
- C++ 库 - 首页
- C++ 库 - <fstream>
- C++ 库 - <iomanip>
- C++ 库 - <ios>
- C++ 库 - <iosfwd>
- C++ 库 - <iostream>
- C++ 库 - <istream>
- C++ 库 - <ostream>
- C++ 库 - <sstream>
- C++ 库 - <streambuf>
- C++ 库 - <atomic>
- C++ 库 - <complex>
- C++ 库 - <exception>
- C++ 库 - <functional>
- C++ 库 - <limits>
- C++ 库 - <locale>
- C++ 库 - <memory>
- C++ 库 - <new>
- C++ 库 - <numeric>
- C++ 库 - <regex>
- C++ 库 - <stdexcept>
- C++ 库 - <string>
- C++ 库 - <thread>
- C++ 库 - <tuple>
- C++ 库 - <typeinfo>
- C++ 库 - <utility>
- C++ 库 - <valarray>
- C++ STL 库
- C++ 库 - <array>
- C++ 库 - <bitset>
- C++ 库 - <deque>
- C++ 库 - <forward_list>
- C++ 库 - <list>
- C++ 库 - <map>
- C++ 库 - <multimap>
- C++ 库 - <queue>
- C++ 库 - <priority_queue>
- C++ 库 - <set>
- C++ 库 - <stack>
- C++ 库 - <unordered_map>
- C++ 库 - <unordered_set>
- C++ 库 - <vector>
- C++ 库 - <algorithm>
- C++ 库 - <iterator>
- C++ 高级库
- C++ 库 - <any>
- C++ 库 - <barrier>
- C++ 库 - <bit>
- C++ 库 - <chrono>
- C++ 库 - <cinttypes>
- C++ 库 - <clocale>
- C++ 库 - <condition_variable>
- C++ 库 - <coroutine>
- C++ 库 - <cstdlib>
- C++ 库 - <cstring>
- C++ 库 - <cuchar>
- C++ 库 - <charconv>
- C++ 库 - <cfenv>
- C++ 库 - <cmath>
- C++ 库 - <ccomplex>
- C++ 库 - <expected>
- C++ 库 - <format>
- C++ 库 - <future>
- C++ 库 - <flat_set>
- C++ 库 - <flat_map>
- C++ 库 - <filesystem>
- C++ 库 - <generator>
- C++ 库 - <initializer_list>
- C++ 库 - <latch>
- C++ 库 - <memory_resource>
- C++ 库 - <mutex>
- C++ 库 - <mdspan>
- C++ 库 - <optional>
- C++ 库 - <print>
- C++ 库 - <ratio>
- C++ 库 - <scoped_allocator>
- C++ 库 - <semaphore>
- C++ 库 - <source_location>
- C++ 库 - <span>
- C++ 库 - <spanstream>
- C++ 库 - <stacktrace>
- C++ 库 - <stop_token>
- C++ 库 - <syncstream>
- C++ 库 - <system_error>
- C++ 库 - <string_view>
- C++ 库 - <stdatomic>
- C++ 库 - <variant>
- C++ STL 库速查表
- C++ STL - 速查表
C++ unordered_multimap::swap() 函数
C++ 的std::unordered_multimap::swap()函数用于交换第一个unordered_multimap与另一个unordered_multimap的内容。只有当unordered_multimap的元素类型相同时,才会进行交换。此函数不依赖于大小。
语法
以下是std::unordered_multimap::swap()函数的语法。
void swap(unordered_multimap<Key,T,Hash,Pred,Alloc>& first, unordered_multimap<Key,T,Hash,Pred,Alloc>& second);
参数
- first − 第一个unordered_multimap对象。
- second − 第二个相同类型的unordered_multimap对象。
返回值
此函数不返回任何值。
示例 1
让我们来看下面的例子,我们将使用swap()函数在两个multimap之间进行交换。
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_multimap<char, int> umm1 = { {'a', 1}, {'b', 2}, {'c', 3}, {'b', 4}, {'c', 5}, }; unordered_multimap<char, int> umm2 = { {'A', 1}, {'B', 2}, {'C', 3}, {'D', 4} }; cout << "umm1 contains following elements before swapping: " << endl; for (auto it = umm1.begin(); it != umm1.end(); ++it) cout << it->first << " = " << it->second << endl; cout << "umm2 contains following elements before swapping: " << endl; for (auto it = umm2.begin(); it != umm2.end(); ++it) cout << it->first << " = " << it->second << endl; swap(umm1, umm2); cout << "umm1 contains following elements after swapping: " << endl; for (auto it = umm1.begin(); it != umm1.end(); ++it) cout << it->first << " = " << it->second << endl; cout << "umm2 contains following elements after swapping: " << endl; for (auto it = umm2.begin(); it != umm2.end(); ++it) cout << it->first << " = " << it->second << endl; return 0; }
输出
如果我们运行上面的代码,它将生成以下输出:
umm1 contains following elements before swapping: c = 5 c = 3 b = 4 b = 2 a = 1 umm2 contains following elements before swapping: D = 4 C = 3 B = 2 A = 1 umm1 contains following elements after swapping: D = 4 C = 3 B = 2 A = 1 umm2 contains following elements after swapping: c = 5 c = 3 b = 4 b = 2 a = 1
示例 2
考虑另一种情况,我们将创建两个multimap,一个包含元素,另一个为空,并应用swap()函数将元素交换到空multimap中。
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_multimap<char, int> umm1 = { {'a', 1}, {'b', 2}, {'c', 3}, {'b', 4}, {'c', 5}, }; unordered_multimap<char, int> umm2; swap(umm1, umm2); cout << "umm2 unordered multimap contains following elements" << endl; for (auto it = umm2.begin(); it != umm2.end(); ++it) cout << it->first << " = " << it->second << endl; return 0; }
输出
以下是上述代码的输出:
umm2 unordered multimap contains following elements c = 5 c = 3 b = 4 b = 2 a = 1
示例 3
在下面的例子中,我们将使用两个multimap,并使用swap()函数相互交换两个multimap的元素。
#include <iostream> #include <unordered_map> using namespace std; int main(void) { unordered_multimap<string, int> John = { {"CHE", 85}, {"PHY", 88}, {"MAT", 99}, {"CMS", 86}, {"ENG", 95} }; unordered_multimap<string, int> Bob = { {"PHY", 90}, {"CHE", 82}, {"MAT", 98}, {"CMS", 88} }; cout<<"Swapping the marks of first unordered multimap with second unordered_multimap"<<endl; swap(John, Bob); cout <<"John contains following marks after swapping: " << endl; for (auto & it: John) cout << it.first << " = " << it.second << endl; cout <<"Bob contains following marks after swapping: " << endl; for (auto & it: Bob) cout << it.first << " = " << it.second << endl; return 0; }
输出
上述代码的输出如下:
Swapping the marks of first unordered multimap with second unordered_multimap john contains following marks after swapping: CMS = 88 MAT = 98 CHE = 82 PHY = 90 Bob contains following marks after swapping: ENG = 95 CMS = 86 MAT = 99 PHY = 88 CHE = 85
广告