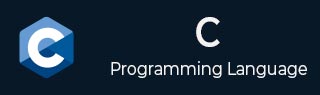
- C编程教程
- C - 首页
- C语言基础
- C - 概述
- C - 特性
- C - 历史
- C - 环境搭建
- C - 程序结构
- C - Hello World
- C - 编译过程
- C - 注释
- C - 词法单元
- C - 关键字
- C - 标识符
- C - 用户输入
- C - 基本语法
- C - 数据类型
- C - 变量
- C - 整数提升
- C - 类型转换
- C - 类型强制转换
- C - 布尔值
- C语言中的常量和字面量
- C - 常量
- C - 字面量
- C - 转义序列
- C - 格式说明符
- C语言中的运算符
- C - 运算符
- C - 算术运算符
- C - 关系运算符
- C - 逻辑运算符
- C - 位运算符
- C - 赋值运算符
- C - 一元运算符
- C - 自增和自减运算符
- C - 三元运算符
- C - sizeof 运算符
- C - 运算符优先级
- C - 其他运算符
- C语言中的决策控制
- C - 决策控制
- C - if 语句
- C - if...else 语句
- C - 嵌套 if 语句
- C - switch 语句
- C - 嵌套 switch 语句
- C语言中的循环
- C - 循环
- C - while 循环
- C - for 循环
- C - do...while 循环
- C - 嵌套循环
- C - 无限循环
- C - break 语句
- C - continue 语句
- C - goto 语句
- C语言中的函数
- C - 函数
- C - 主函数
- C - 按值传递函数调用
- C - 按引用传递函数调用
- C - 嵌套函数
- C - 可变参数函数
- C - 用户自定义函数
- C - 回调函数
- C - 返回语句
- C - 递归
- C语言中的作用域规则
- C - 作用域规则
- C - 静态变量
- C - 全局变量
- C语言中的数组
- C - 数组
- C - 数组的特性
- C - 多维数组
- C - 将数组传递给函数
- C - 从函数返回数组
- C - 变长数组
- C语言中的指针
- C - 指针
- C - 指针和数组
- C - 指针的应用
- C - 指针运算
- C - 指针数组
- C - 指针到指针
- C - 将指针传递给函数
- C - 从函数返回指针
- C - 函数指针
- C - 指向数组的指针
- C - 指向结构体的指针
- C - 指针链
- C - 指针与数组
- C - 字符指针和函数
- C - 空指针
- C - void 指针
- C - 悬空指针
- C - 解引用指针
- C - 近、远和巨型指针
- C - 指针数组的初始化
- C - 指针与多维数组
- C语言中的字符串
- C - 字符串
- C - 字符串数组
- C - 特殊字符
- C语言中的结构体和联合体
- C - 结构体
- C - 结构体和函数
- C - 结构体数组
- C - 自引用结构体
- C - 查找表
- C - 点(.)运算符
- C - 枚举(或 enum)
- C - 结构体填充和打包
- C - 嵌套结构体
- C - 匿名结构体和联合体
- C - 联合体
- C - 位域
- C - Typedef
- C语言中的文件处理
- C - 输入和输出
- C - 文件I/O(文件处理)
- C预处理器
- C - 预处理器
- C - 指令
- C - 预处理器运算符
- C - 宏
- C - 头文件
- C语言中的内存管理
- C - 内存管理
- C - 内存地址
- C - 存储类
- 其他主题
- C - 错误处理
- C - 可变参数
- C - 命令执行
- C - 数学函数
- C - static 关键字
- C - 随机数生成
- C - 命令行参数
- C编程资源
- C - 问答
- C - 快速指南
- C - 速查表
- C - 有用资源
- C - 讨论
C语言中的结构体数组
在 C 编程中,struct 关键字用于定义派生数据类型。定义后,您可以声明结构体变量的数组,就像声明int、float或char类型的数组一样。结构体数组有很多用例,例如存储类似于数据库表的记录,其中每行都有不同的数据类型。
通常,结构体类型在代码开头定义,以便可以在任何函数内部使用其类型。您可以声明结构体数组,然后在其中填充数据,或者在声明时初始化它。
初始化结构体数组
让我们定义一个名为book的结构体类型,如下所示:
struct book{ char title[10]; double price; int pages; };
在程序中,您可以声明一个数组并在花括号内给出每个元素的值来初始化它。结构体数组中的每个元素本身都是一个结构体值。因此,我们有如下所示的嵌套花括号:
struct book b[3] = { {"Learn C", 650.50, 325}, {"C Pointers", 175, 225}, {"C Pearls", 250, 250} };
编译器如何为该数组分配内存?由于我们有一个包含三个元素的数组,这些元素的结构体大小为 32 字节,因此该数组占用“32 x 3”字节。每块 32 字节将容纳一个“title”、“price”和“pages”元素。
学 | 习 | 资 | 源 | 网 | 站 | 675.50 | 325 | ||||
站 | 编 | 程 | 教 | 网 | 程 | 习 | 源 | 资 | 175 | 225 | |
站 | 编 | 习 | 资 | 源 | 学 | 资 | 250 | 250 |
声明结构体数组
您还可以声明一个空的结构体数组。之后,您可以使用 scanf() 语句读取其中的数据,或者为每个元素赋值,如下所示:
struct book b[3]; strcpy(b[0].title, "Learn C"); b[0].price = 650.50; b[0].pages=325; strcpy(b[1].title, "C Pointers"); b[1].price = 175; b[1].pages=225; strcpy(b[2].title, "C Pearls"); b[2].price = 250;250 b[2].pages=325;
读取结构体数组
我们还可以接受用户的输入来填充数组。
示例 1
在以下代码中,for循环用于接受数组中每个结构体元素的“title”、“price”和“pages”元素的输入。
#include <stdio.h> struct book{ char title[10]; double price; int pages; }; int main(){ struct book b[3]; strcpy(b[0].title, "Learn C"); b[0].price = 650.50; b[0].pages = 325; strcpy(b[1].title, "C Pointers"); b[1].price = 175; b[1].pages = 225; strcpy(b[2].title, "C Pearls"); b[2].price = 250; b[2].pages = 325; printf("\nList of Books:\n"); for (int i = 0; i < 3; i++){ printf("Title: %s \tPrice: %7.2lf \tPages: %d\n", b[i].title, b[i].price, b[i].pages); } return 0; }
输出
运行此代码时,将产生以下输出:
List of Books: Title: Learn C Price: 650.50 Pages: 325 Title: C Pointers Price: 175.00 Pages: 225 Title: C Pearls Price: 250.00 Pages: 325
示例 2
在此示例中,定义了一个名为student的结构体类型。其元素为“name”;物理、化学和数学成绩;以及“percentage”。
声明了一个包含三个结构体 student 类型的数组,并使用for循环填充前四个元素。在循环本身内部,计算每个下标的“percent”元素。
最后,打印一个包含学生姓名、分数和百分比的数组,以显示成绩单。
#include <stdio.h> struct student{ char name[10]; int physics, chemistry, maths; double percent; }; int main(){ struct student s[3]; strcpy(s[0].name, "Ravi"); s[0].physics = 50; s[0].chemistry = 60; s[0].maths = 70; strcpy(s[1].name, "Kiran"); s[1].physics = 55; s[1].chemistry = 66; s[1].maths = 77; strcpy(s[2].name, "Anil"); s[2].physics = 45; s[2].chemistry = 55; s[2].maths = 65; for (int i = 0; i < 3; i++){ s[i].percent = (double)(s[i].physics + s[i].maths + s[i].chemistry)/3; } printf("\nName\tPhysics\tChemistry\t\Maths\tPercent\n"); for(int i = 0; i < 3; i++){ printf("%s \t\t%d \t\t%d \t\t%d \t\t%5.2lf\n", s[i].name, s[i].physics, s[i].chemistry, s[i].maths, s[i].percent); } return 0; }
输出
运行此代码时,将产生以下输出:
Name Physics Chemistry Maths Percent Ravi 50 60 70 60.00 Kiran 55 66 77 66.00 Anil 45 55 65 55.00
排序结构体数组
让我们再举一个结构体数组的例子。在这里,我们将通过实现冒泡排序技术,使“book”结构体类型的数组按价格升序排序。
注意:可以使用赋值运算符将一个结构体变量的元素直接赋值给另一个结构体变量。
示例
请看下面的示例:
#include <stdio.h> struct book{ char title[15]; double price; int pages; }; int main(){ struct book b[3] = { {"Learn C", 650.50, 325}, {"C Pointers", 175, 225}, {"C Pearls", 250, 250} }; int i, j; struct book temp; for(i = 0; i < 2; i++){ for(j = i; j < 3; j++){ if (b[i].price > b[j].price){ temp = b[i]; b[i] = b[j]; b[j] = temp; } } } printf("\nList of Books in Ascending Order of Price:\n"); for (i = 0; i < 3; i++){ printf("Title: %s \tPrice: %7.2lf \tPages: %d\n", b[i].title, b[i].price, b[i].pages); } return 0; }
输出
运行此代码时,将产生以下输出:
List of Books in Ascending Order of Price: Title: C Pointers Price: 175.00 Pages: 225 Title: C Pearls Price: 250.00 Pages: 250 Title: Learn C Price: 650.50 Pages: 325
声明指向结构体数组的指针
我们还可以声明指向结构体数组的指针。C 使用间接运算符 (→) 来访问结构体变量的内部元素。
示例
以下示例显示了如何声明指向结构体数组的指针:
#include <stdio.h> struct book { char title[15]; double price; int pages; }; int main(){ struct book b[3] = { {"Learn C", 650.50, 325}, {"C Pointers", 175, 225}, {"C Pearls", 250, 250} }; struct book *ptr = b; for(int i = 0; i < 3; i++){ printf("Title: %s \tPrice: %7.2lf \tPages: %d\n", ptr -> title, ptr -> price, ptr -> pages); ptr++; } return 0; }
输出
运行此代码时,将产生以下输出:
Title: Learn C Price: 650.50 Pages: 325 Title: C Pointers Price: 175.00 Pages: 225 Title: C Pearls Price: 250.00 Pages: 250