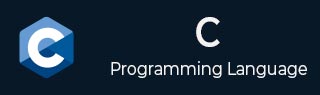
- C编程教程
- C - 首页
- C语言基础
- C - 概述
- C - 特性
- C - 历史
- C - 环境搭建
- C - 程序结构
- C - Hello World
- C - 编译过程
- C - 注释
- C - 词法单元
- C - 关键字
- C - 标识符
- C - 用户输入
- C - 基本语法
- C - 数据类型
- C - 变量
- C - 整数提升
- C - 类型转换
- C - 类型强制转换
- C - 布尔值
- C语言中的常量和字面量
- C - 常量
- C - 字面量
- C - 转义序列
- C - 格式说明符
- C语言中的运算符
- C - 运算符
- C - 算术运算符
- C - 关系运算符
- C - 逻辑运算符
- C - 位运算符
- C - 赋值运算符
- C - 一元运算符
- C - 自增和自减运算符
- C - 三元运算符
- C - sizeof 运算符
- C - 运算符优先级
- C - 其他运算符
- C语言中的决策语句
- C - 决策语句
- C - if 语句
- C - if...else 语句
- C - 嵌套 if 语句
- C - switch 语句
- C - 嵌套 switch 语句
- C语言中的循环
- C - 循环
- C - while 循环
- C - for 循环
- C - do...while 循环
- C - 嵌套循环
- C - 无限循环
- C - break 语句
- C - continue 语句
- C - goto 语句
- C语言中的函数
- C - 函数
- C - 主函数
- C - 按值传递函数调用
- C - 按引用传递函数调用
- C - 嵌套函数
- C - 可变参数函数
- C - 用户定义函数
- C - 回调函数
- C - 返回语句
- C - 递归
- C语言中的作用域规则
- C - 作用域规则
- C - 静态变量
- C - 全局变量
- C语言中的数组
- C - 数组
- C - 数组的特性
- C - 多维数组
- C - 将数组传递给函数
- C - 从函数返回数组
- C - 变长数组
- C语言中的指针
- C - 指针
- C - 指针和数组
- C - 指针的应用
- C - 指针运算
- C - 指针数组
- C - 指向指针的指针
- C - 将指针传递给函数
- C - 从函数返回指针
- C - 函数指针
- C - 指向数组的指针
- C - 指向结构体的指针
- C - 指针链
- C - 指针与数组
- C - 字符指针和函数
- C - 空指针
- C - void 指针
- C - 悬空指针
- C - 解引用指针
- C - 近指针、远指针和巨指针
- C - 指针数组的初始化
- C - 指针与多维数组
- C语言中的字符串
- C - 字符串
- C - 字符串数组
- C - 特殊字符
- C语言中的结构体和联合体
- C - 结构体
- C - 结构体和函数
- C - 结构体数组
- C - 自引用结构体
- C - 查找表
- C - 点(.)运算符
- C - 枚举(或枚举类型)
- C - 结构体填充和打包
- C - 嵌套结构体
- C - 匿名结构体和联合体
- C - 联合体
- C - 位域
- C - Typedef
- C语言中的文件处理
- C - 输入输出
- C - 文件I/O(文件处理)
- C预处理器
- C - 预处理器
- C - 指令
- C - 预处理器运算符
- C - 宏
- C - 头文件
- C语言中的内存管理
- C - 内存管理
- C - 内存地址
- C - 存储类
- 其他主题
- C - 错误处理
- C - 可变参数
- C - 命令执行
- C - 数学函数
- C - static 关键字
- C - 随机数生成
- C - 命令行参数
- C编程资源
- C - 问答
- C - 快速指南
- C - 速查表
- C - 有用资源
- C - 讨论
C语言中的三元运算符
C语言中的三元运算符(?:)是一种条件运算符。“三元”表示该运算符有三个操作数。三元运算符通常用于以更紧凑的方式编写多个条件(if-else)语句。
C语言中三元运算符的语法
三元运算符使用以下语法:
exp1 ? exp2 : exp3
它使用三个**操作数**:
- **exp1** - 求值为真或假的布尔表达式
- **exp2** - 当 exp1 为真时,由 ? 运算符返回
- **exp3** - 当 exp1 为假时,由 ? 运算符返回
示例 1:C语言中的三元运算符
以下C程序使用三元运算符检查变量的值是偶数还是奇数。
#include <stdio.h> int main(){ int a = 10; (a % 2 == 0) ? printf("%d is Even \n", a) : printf("%d is Odd \n", a); return 0; }
输出
运行此代码时,将产生以下输出:
10 is Even
将“a”的值更改为 15 并再次运行代码。现在您将获得以下**输出**:
15 is Odd
示例 2
条件运算符是 if-else 结构的紧凑表示形式。我们可以通过以下代码重写检查奇偶数的逻辑:
#include <stdio.h> int main(){ int a = 10; if (a % 2 == 0){ printf("%d is Even\n", a); } else{ printf("%d is Odd\n", a); } return 0; }
输出
运行代码并检查其输出:
10 is Even
示例 3
以下程序比较两个变量“a”和“b”,并将较大的值赋给变量“c”。
#include <stdio.h> int main(){ int a = 100, b = 20, c; c = (a >= b) ? a : b; printf ("a: %d b: %d c: %d\n", a, b, c); return 0; }
输出
运行此代码时,将产生以下输出:
a: 100 b: 20 c: 100
示例 4
使用 if-else 结构的相应代码如下:
#include <stdio.h> int main(){ int a = 100, b = 20, c; if (a >= b){ c = a; } else { c = b; } printf ("a: %d b: %d c: %d\n", a, b, c); return 0; }
输出
运行代码并检查其输出:
a: 100 b: 20 c: 100
示例 5
如果需要在三元运算符的真操作数和/或假操作数中放置多个语句,则必须用逗号分隔它们,如下所示:
#include <stdio.h> int main(){ int a = 100, b = 20, c; c = (a >= b) ? printf ("a is larger "), c = a : printf("b is larger "), c = b; printf ("a: %d b: %d c: %d\n", a, b, c); return 0; }
输出
在此代码中,较大的数字被赋值给“c”,并打印相应的提示信息。
a is larger a: 100 b: 20 c: 20
示例 6
使用 if-else 语句的相应程序如下:
#include <stdio.h> int main(){ int a = 100, b = 20, c; if(a >= b){ printf("a is larger \n"); c = a; } else{ printf("b is larger \n"); c = b; } printf ("a: %d b: %d c: %d\n", a, b, c); return 0; }
输出
运行代码并检查其输出:
a is larger a: 100 b: 20 c: 100
嵌套三元运算符
就像我们可以使用嵌套 if-else 语句一样,我们可以在真操作数和假操作数中使用三元运算符。
exp1 ? (exp2 ? expr3 : expr4) : (exp5 ? expr6: expr7)
首先,C 检查 expr1 是否为真。如果是,则检查 expr2。如果为真,则结果为 expr3;如果为假,则结果为 expr4。
如果 expr1 为假,则它可能会检查 expr5 是否为真,并返回 expr6 或 expr7。
示例 1
让我们开发一个C程序来确定一个数字是否能被 2 和 3 整除,或者被 2 整除但不能被 3 整除,或者被 3 整除但不能被 2 整除,或者既不能被 2 也不能被 3 整除。我们将为此目的使用嵌套条件运算符,如下面的代码所示:
#include <stdio.h> int main(){ int a = 15; printf("a: %d\n", a); (a % 2 == 0) ? ( (a%3 == 0)? printf("divisible by 2 and 3") : printf("divisible by 2 but not 3")) : ( (a%3 == 0)? printf("divisible by 3 but not 2") : printf("not divisible by 2, not divisible by 3") ); return 0; }
输出
检查不同的值:
a: 15 divisible by 3 but not 2 a: 16 divisible by 2 but not 3 a: 17 not divisible by 2, not divisible by 3 a: 18 divisible by 2 and 3
示例 2
在此程序中,我们使用嵌套 if-else 语句实现了相同的功能,而不是使用条件运算符:
#include <stdio.h> int main(){ int a = 15; printf("a: %d\n", a); if(a % 2 == 0){ if (a % 3 == 0){ printf("divisible by 2 and 3"); } else { printf("divisible by 2 but not 3"); } } else{ if(a % 3 == 0){ printf("divisible by 3 but not 2"); } else { printf("not divisible by 2, not divisible by 3"); } } return 0; }
输出
运行此代码时,将产生以下输出:
a: 15 divisible by 3 but not 2