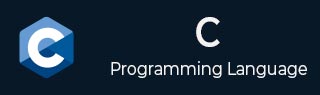
- C编程教程
- C语言 - 首页
- C语言基础
- C语言 - 概述
- C语言 - 特性
- C语言 - 历史
- C语言 - 环境搭建
- C语言 - 程序结构
- C语言 - Hello World
- C语言 - 编译过程
- C语言 - 注释
- C语言 - 词法单元
- C语言 - 关键字
- C语言 - 标识符
- C语言 - 用户输入
- C语言 - 基本语法
- C语言 - 数据类型
- C语言 - 变量
- C语言 - 整数提升
- C语言 - 类型转换
- C语言 - 类型强制转换
- C语言 - 布尔值
- C语言中的常量和字面量
- C语言 - 常量
- C语言 - 字面量
- C语言 - 转义序列
- C语言 - 格式说明符
- C语言中的运算符
- C语言 - 运算符
- C语言 - 算术运算符
- C语言 - 关系运算符
- C语言 - 逻辑运算符
- C语言 - 位运算符
- C语言 - 赋值运算符
- C语言 - 一元运算符
- C语言 - 自增和自减运算符
- C语言 - 三元运算符
- C语言 - sizeof 运算符
- C语言 - 运算符优先级
- C语言 - 其他运算符
- C语言中的决策
- C语言 - 决策
- C语言 - if 语句
- C语言 - if...else 语句
- C语言 - 嵌套 if 语句
- C语言 - switch 语句
- C语言 - 嵌套 switch 语句
- C语言中的循环
- C语言 - 循环
- C语言 - while 循环
- C语言 - for 循环
- C语言 - do...while 循环
- C语言 - 嵌套循环
- C语言 - 死循环
- C语言 - break 语句
- C语言 - continue 语句
- C语言 - goto 语句
- C语言中的函数
- C语言 - 函数
- C语言 - 主函数
- C语言 - 按值调用函数
- C语言 - 按引用调用函数
- C语言 - 嵌套函数
- C语言 - 变参函数
- C语言 - 用户自定义函数
- C语言 - 回调函数
- C语言 - 返回语句
- C语言 - 递归
- C语言中的作用域规则
- C语言 - 作用域规则
- C语言 - 静态变量
- C语言 - 全局变量
- C语言中的数组
- C语言 - 数组
- C语言 - 数组的特性
- C语言 - 多维数组
- C语言 - 将数组传递给函数
- C语言 - 从函数返回数组
- C语言 - 变长数组
- C语言中的指针
- C语言 - 指针
- C语言 - 指针和数组
- C语言 - 指针的应用
- C语言 - 指针运算
- C语言 - 指针数组
- C语言 - 指向指针的指针
- C语言 - 将指针传递给函数
- C语言 - 从函数返回指针
- C语言 - 函数指针
- C语言 - 指向数组的指针
- C语言 - 指向结构体的指针
- C语言 - 指针链
- C语言 - 指针与数组的区别
- C语言 - 字符指针和函数
- C语言 - 空指针
- C语言 - void 指针
- C语言 - 悬空指针
- C语言 - 解引用指针
- C语言 - 近指针、远指针和巨大指针
- C语言 - 指针数组的初始化
- C语言 - 指针与多维数组的比较
- C语言中的字符串
- C语言 - 字符串
- C语言 - 字符串数组
- C语言 - 特殊字符
- C语言中的结构体和联合体
- C语言 - 结构体
- C语言 - 结构体和函数
- C语言 - 结构体数组
- C语言 - 自引用结构体
- C语言 - 查找表
- C语言 - 点(.)运算符
- C语言 - 枚举(enum)
- C语言 - 结构体填充和打包
- C语言 - 嵌套结构体
- C语言 - 匿名结构体和联合体
- C语言 - 联合体
- C语言 - 位域
- C语言 - typedef
- C语言中的文件处理
- C语言 - 输入与输出
- C语言 - 文件I/O(文件处理)
- C语言预处理器
- C语言 - 预处理器
- C语言 - 编译指示
- C语言 - 预处理器运算符
- C语言 - 宏
- C语言 - 头文件
- C语言中的内存管理
- C语言 - 内存管理
- C语言 - 内存地址
- C语言 - 存储类别
- 其他主题
- C语言 - 错误处理
- C语言 - 可变参数
- C语言 - 命令执行
- C语言 - 数学函数
- C语言 - static关键字
- C语言 - 随机数生成
- C语言 - 命令行参数
- C编程资源
- C语言 - 问答
- C语言 - 快速指南
- C语言 - 速查表
- C语言 - 有用资源
- C语言 - 讨论
C语言中的命令执行
C语言中的命令执行
C语言中的命令执行用于使用C程序执行系统命令。系统命令通过使用system()函数执行,该函数是stdlib.h头文件的库函数。
使用system()函数,可以在C程序中执行Windows/Linux终端命令。
语法
以下是执行系统命令的语法:
system(char *command);
命令执行示例
以下代码显示了在C语言中使用system()函数执行ls命令。
#include <stdio.h> #include<stdlib.h> #include<string.h> int main() { char cmd[10]; strcpy(cmd,"dir C:\\users\\user\\*.c"); system(cmd); return 0; }
输出
运行代码并检查其输出:
C:\Users\user>dir *.c Volume in drive C has no label. Volume Serial Number is 7EE4-E492 Directory of C:\Users\user 04/01/2024 01:30 PM 104 add.c 04/02/2024 01:37 PM 159 add1.c 04/02/2024 01:37 PM 259 array.c 04/02/2024 01:37 PM 149 main.c 04/02/2024 01:37 PM 180 recursion.c 04/02/2024 01:37 PM 241 struct.c 04/02/2024 01:37 PM 172 voidptr.c 7 File(s) 1,264 bytes 0 Dir(s) 139,073,761,280 bytes
C语言中的exec函数族
"unistd.h"头文件中引入了exec函数族。这些函数用于执行文件,一旦调用,它们就会用新的进程映像替换当前进程映像。
以下是C语言中exec函数族中的函数:
- execl()函数
- execlp()函数
- execle()函数
- execv()函数
- execvp()函数
- execve()函数
1. execl()函数
execl()函数的第一个参数是可执行文件作为其第一个参数。接下来的参数在执行时将提供给文件。最后一个参数必须为NULL。
int execl(const char *pathname, const char *arg, ..., NULL)
示例
请看下面的例子:
#include <unistd.h> int main(void) { char *file = "/usr/bin/echo"; char *arg1 = "Hello world!"; execl(file, file, arg1, NULL); return 0; }
Linux中的echo命令通过C代码调用。
输出
保存、编译和执行上述程序:
$ gcc hello.c -o hello $ ./hello Hello world!
2. execlp()函数
execlp()函数类似于execl()函数。它使用PATH环境变量来定位文件。因此,无需提供可执行文件的路径。
int execlp(const char *file, const char *arg, ..., NULL)
示例
请看下面的例子:
#include <unistd.h> int main(void) { char *file = "echo"; char *arg1 = "Hello world!"; execlp(file, file, arg1, NULL); return 0; }
输出
这里,echo已经位于PATH环境变量中。保存、编译并从终端运行。
$ gcc hello.c -o hello $ ./hello Hello world!
3. execle()函数
在execle()函数中,我们可以将环境变量传递给函数,它将使用这些变量。其原型如下:
int execle(const char *pathname, const char *arg, ..., NULL, char *const envp[])
示例
请看下面的例子:
#include <unistd.h> int main(void) { char *file = "/usr/bin/bash"; char *arg1 = "-c"; char *arg2 = "echo $ENV1 $ENV2!"; char *const env[] = {"ENV1 = Hello", "ENV2 = World", NULL}; execle(file, file, arg1, arg2, NULL, env); return 0; }
输出
保存、编译并从终端运行:
$ gcc hello.c -o hello $ ./hello Hello world!
4. execv()函数
execv()函数接收一个参数向量,这些参数将提供给可执行文件。此外,向量的最后一个元素必须为NULL
int execv(const char *pathname, char *const argv[])
示例
请看下面的例子:
#include <unistd.h> int main(void) { char *file = "/usr/bin/echo"; char *const args[] = {"/usr/bin/echo", "Hello world!", NULL}; execv(file, args); return 0; }
输出
保存、编译和执行上述程序:
$ gcc hello.c -o hello $ ./hello Hello world!
5. execvp()函数
execvp()函数具有以下语法:
int execvp(const char *file, char *const argv[])
示例
请看下面的例子:
#include <unistd.h> int main(void) { char *file = "echo"; char *const args[] = {"/usr/bin/echo", "Hello world!", NULL}; execvp(file, args); return 0; }
输出
保存、编译和执行上述程序:
$ gcc hello.c -o hello $ ./hello Hello world!
6. execve()函数
除了环境变量之外,我们还可以将其他参数作为NULL终止的向量传递给execve()函数:
int execve(const char *pathname, char *const argv[], char *const envp[])
示例
请看下面的例子:
#include <unistd.h> int main(void) { char *file = "/usr/bin/bash"; char *const args[] = {"/usr/bin/bash", "-c", "echo Hello $ENV!", NULL}; char *const env[] = {"ENV=World", NULL}; execve(file, args, env); return 0; }
输出
保存、编译和执行上述程序:
$ gcc hello.c -o hello $ ./hello Hello world!
广告