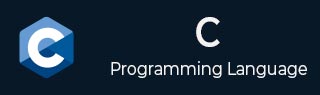
- C编程教程
- C语言 - 首页
- C语言基础
- C语言 - 概述
- C语言 - 特性
- C语言 - 历史
- C语言 - 环境搭建
- C语言 - 程序结构
- C语言 - Hello World
- C语言 - 编译过程
- C语言 - 注释
- C语言 - 词法单元
- C语言 - 关键字
- C语言 - 标识符
- C语言 - 用户输入
- C语言 - 基本语法
- C语言 - 数据类型
- C语言 - 变量
- C语言 - 整数提升
- C语言 - 类型转换
- C语言 - 类型强制转换
- C语言 - 布尔值
- C语言中的常量和字面量
- C语言 - 常量
- C语言 - 字面量
- C语言 - 转义序列
- C语言 - 格式说明符
- C语言中的运算符
- C语言 - 运算符
- C语言 - 算术运算符
- C语言 - 关系运算符
- C语言 - 逻辑运算符
- C语言 - 位运算符
- C语言 - 赋值运算符
- C语言 - 一元运算符
- C语言 - 自增和自减运算符
- C语言 - 三元运算符
- C语言 - sizeof 运算符
- C语言 - 运算符优先级
- C语言 - 其他运算符
- C语言中的决策语句
- C语言 - 决策语句
- C语言 - if 语句
- C语言 - if...else 语句
- C语言 - 嵌套 if 语句
- C语言 - switch 语句
- C语言 - 嵌套 switch 语句
- C语言中的循环
- C语言 - 循环
- C语言 - while 循环
- C语言 - for 循环
- C语言 - do...while 循环
- C语言 - 嵌套循环
- C语言 - 死循环
- C语言 - break 语句
- C语言 - continue 语句
- C语言 - goto 语句
- C语言中的函数
- C语言 - 函数
- C语言 - 主函数
- C语言 - 按值传递函数
- C语言 - 按引用传递函数
- C语言 - 嵌套函数
- C语言 - 可变参数函数
- C语言 - 用户自定义函数
- C语言 - 回调函数
- C语言 - 返回语句
- C语言 - 递归
- C语言中的作用域规则
- C语言 - 作用域规则
- C语言 - 静态变量
- C语言 - 全局变量
- C语言中的数组
- C语言 - 数组
- C语言 - 数组的特性
- C语言 - 多维数组
- C语言 - 将数组传递给函数
- C语言 - 从函数返回数组
- C语言 - 变长数组
- C语言中的指针
- C语言 - 指针
- C语言 - 指针和数组
- C语言 - 指针的应用
- C语言 - 指针运算
- C语言 - 指针数组
- C语言 - 指向指针的指针
- C语言 - 将指针传递给函数
- C语言 - 从函数返回指针
- C语言 - 函数指针
- C语言 - 指向数组的指针
- C语言 - 指向结构体的指针
- C语言 - 指针链
- C语言 - 指针与数组的区别
- C语言 - 字符指针和函数
- C语言 - 空指针
- C语言 - void 指针
- C语言 - 野指针
- C语言 - 解引用指针
- C语言 - 近指针、远指针和巨指针
- C语言 - 指针数组的初始化
- C语言 - 指针与多维数组的区别
- C语言中的字符串
- C语言 - 字符串
- C语言 - 字符串数组
- C语言 - 特殊字符
- C语言中的结构体和联合体
- C语言 - 结构体
- C语言 - 结构体和函数
- C语言 - 结构体数组
- C语言 - 自引用结构体
- C语言 - 查找表
- C语言 - 点(.)运算符
- C语言 - 枚举(enum)
- C语言 - 结构体填充和打包
- C语言 - 嵌套结构体
- C语言 - 匿名结构体和联合体
- C语言 - 联合体
- C语言 - 位域
- C语言 - typedef
- C语言中的文件处理
- C语言 - 输入输出
- C语言 - 文件I/O (文件处理)
- C语言预处理器
- C语言 - 预处理器
- C语言 - 编译指示
- C语言 - 预处理器运算符
- C语言 - 宏
- C语言 - 头文件
- C语言中的内存管理
- C语言 - 内存管理
- C语言 - 内存地址
- C语言 - 存储类
- 其他主题
- C语言 - 错误处理
- C语言 - 可变参数
- C语言 - 命令执行
- C语言 - 数学函数
- C语言 - static 关键字
- C语言 - 随机数生成
- C语言 - 命令行参数
- C编程资源
- C语言 - 问答
- C语言 - 快速指南
- C语言 - 速查表
- C语言 - 有用资源
- C语言 - 讨论
C语言速查表
这份C语言速查表快速概述了C语言的概念,从基础到高级。这份速查表对学生、开发者和正在准备面试的人非常有用。通读这份速查表,学习C编程语言的所有基本和高级概念。
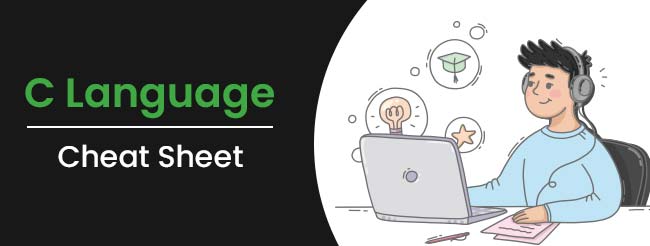
C程序的基本结构
C程序的基本结构让你了解在C语言中编写程序所需的基本语句。以下是C程序的基本结构:
// Preprocessor directive/header file inclusion section #include <stdio.h> // Global declaration section // the main() function int main() { // Variable declarations section int x, y; // other code statements section // Return o return 0; } // Other user-defined function definition section
#include <stdio.h>
#include 是一个预处理器指令,它包含C程序中的头文件。stdio.h 是一个头文件,其中定义了所有与输入输出相关的函数。
main() 函数
main() 函数是C程序的入口点,程序的执行从main() 函数开始。
以下是main() 函数的语法:
int main() { return 0; }
注释
C语言中有两种类型的注释。单行注释和多行注释。编译器会忽略注释。
单行注释
使用 // 来编写单行注释。
// This is a single-line comment
多行注释
在文本之前和之后使用 /* 和 */ 来在C语言中编写多行注释。
/* This is line 1 This is line 2 .. */
打印(printf() 函数)
printf() 函数是一个库函数,用于在控制台输出上打印格式化的文本。每当你想打印任何内容时,都使用 printf()。
示例
printf("Hello world");
用户输入(scanf() 函数)
scanf() 函数用于从用户处获取各种类型的输入。
以下是 scanf() 函数的语法:
scanf("format_specifier", &variable_name);
格式说明符
以下是printf() 和scanf() 函数中使用的C格式说明符列表,用于打印/输入特定类型的数值。
格式说明符 | 类型 |
---|---|
%c | 字符 |
%d | 带符号整数 |
%e 或 %E | 浮点数的科学计数法 |
%f | 浮点值 |
%g 或 %G | 类似于 %e 或 %E |
%hi | 带符号整数(短整型) |
%hu | 无符号整数(短整型) |
%i | 无符号整数 |
%l 或 %ld 或 %li | 长整型 |
%lf | 双精度浮点数 |
%Lf | 长双精度浮点数 |
%lu | 无符号整型或无符号长整型 |
%lli 或 %lld | 长长整型 |
%llu | 无符号长长整型 |
%o | 八进制表示 |
%p | 指针 |
%s | 字符串 |
%u | 无符号整型 |
%x 或 %X | 十六进制表示 |
示例
#include <stdio.h> int main(){ int age = 18; float percent = 67.75; printf("Age: %d \nPercent: %f", age, percent); return 0; }
输出
Age: 18 Percent: 67.750000
数据类型
数据类型指定存储在变量中的数据的类型和大小。数据类型分为3类:
- 基本数据类型
- 派生数据类型
- 用户自定义数据类型
基本数据类型
基本数据类型是C语言中的内置数据类型,它们也用于创建派生数据类型。
数据类型 | 名称 | 描述 |
---|---|---|
int | 整数 | 表示整数值 |
char | 字符 | 表示单个字符 |
float | 浮点数 | 表示浮点值 |
派生数据类型
派生数据类型是从基本数据类型派生出来的。派生数据类型包括:
- 数组
- 指针
用户自定义数据类型
用户自定义数据类型是由程序员创建的,用于处理不同类型的数据,并根据需求而定。用户自定义数据类型包括:
- 结构体
- 联合体
- 枚举
基本的输入输出
对于C语言中的基本输入和输出,我们使用printf() 和scanf() 函数。
printf() 函数用于在控制台上打印格式化的文本。
printf("Hello world");
scanf() 函数用于从用户处获取输入。
scanf("%d", &x); // Integer input scanf("%f", &y); // float input scanf("%c", &z); // Character Input scanf("%s", name); // String input
基本输入输出示例
#include <stdio.h> int main() { int num; printf("Input any integer number: "); scanf("%d", &num); printf("The input is: %d\n", num); return 0; }
输出
Input any integer number: The input is: 0
标识符
C标识符是用户为变量、常量、函数等定义的名称。定义标识符的规则如下:
- 关键字不能用作标识符。
- 标识符中只允许使用字母、下划线符号 (_) 和数字。
- 标识符必须以字母或下划线开头。
- 同一个标识符不能用作两个实体的名称。
- 标识符应该有意义且具有描述性。
有效标识符示例
age, _name, person1, roll_no
关键字
C关键字是C编译器中的保留字,它们用于特定目的,不能用作标识符。
以下是C语言中的关键字:
auto | double | int | struct |
break | else | long | switch |
case | enum | register | typedef |
char | extern | return | union |
continue | for | signed | void |
do | if | static | while |
default | goto | sizeof | volatile |
const | float | short | unsigned |
变量
C变量是赋予存储区域的名称,我们的程序可以使用它来访问和操作数据。
声明变量的语法
data_type variable_name;
转义序列
转义序列是特殊字符后跟转义符(反斜杠 \)。转义序列具有特殊含义,用于打印那些无法正常打印的字符。
以下是C语言中的转义序列列表:
转义序列 | 含义 |
---|---|
\\ | \字符 |
\' | '字符 |
\" | "字符 |
\? | ?字符 |
\a | 警告或铃声 |
\b | 退格 |
\f | 换页 |
\n | 换行 |
\r | 回车 |
\t | 水平制表符 |
\v | 垂直制表符 |
\ooo | 一位到三位八进制数 |
\xhh ... | 一位或多位十六进制数 |
运算符
运算符是用于执行特定数学或逻辑运算的特殊符号。
以下是C语言中使用的运算符:
运算符 | 符号 | 描述 |
---|---|---|
赋值运算符 | =, +=, -=, <<= | 执行赋值操作,即为变量赋值。 |
算术运算符 | +, -, *, /, % | 执行算术运算。 |
关系运算符 | <, >, <=, >=, ==, != | 对两个操作数进行比较。 |
逻辑运算符 | &&, ||, ! | 执行逻辑运算,例如逻辑与、或和非。 |
位运算符 | &, ^, |, <<, >>, ~ | 执行位运算。 |
三元运算符 | ? : | 执行条件操作以进行决策。 |
其他运算符 | ,sizeof,&,*,⇒,。 | 用于执行各种其他操作。 |
运算符示例
result = num1 + num2; if(result >=10){ printf("Greater than 10."); }
条件语句
C语言提供以下条件语句:
- if语句
- if-else语句
- if-else-if语句
- 嵌套if-else语句
- switch语句
- 三元运算符
if语句
一个if语句由一个布尔表达式和一个或多个语句组成。
if语句的语法如下:
if(boolean_expression) { /* statement(s) will execute if the boolean expression is true */ }
if-else语句
一个if-else语句可以后跟一个可选的else语句,当布尔表达式为假时执行。
if语句的语法如下:
if (Boolean expr){ Expression; . . . } else{ Expression; . . . }
if-else-if语句
if-else-if语句也称为阶梯式if-else。当条件不为真时,它用于检查多个条件。
if-else-if语句的语法:
if(condition1){ } else if(condition2){ } … else{ }
嵌套if语句
通过使用嵌套if语句,你可以在另一个if或else-if语句内使用一个if或else-if语句。
嵌套if语句的语法:
if (expr1){ if (expr2){ block to be executed when expr1 and expr2 are true } else{ block to be executed when expr1 is true but expr2 is false } }
switch语句
一个switch语句允许测试变量与值的列表是否相等。
switch语句的语法如下:
switch (Expression){ // if expr equals Value1 case Value1: Statement1; Statement2; break; // if expr equals Value2 case Value2: Statement1; Statement2; break; . . // if expr is other than the specific values above default: Statement1; Statement2; }
三元运算符
三元运算符(?:) 也称为条件运算符。它可以用作if-else语句的替代。
三元运算符的语法如下:
(condition) ? true_block: false_block;
循环
C语言循环用于分别执行一个或多个语句块指定的次数,或直到达到某个条件。以下是C语言中的循环语句:
- while循环
- do...while循环
- for循环
while循环
while循环是一种入口控制循环,其中在执行循环体之前检查条件。
while循环的语法如下:
while(test_condition){ // Statement(s); }
do…while循环
do…while循环是一种出口控制循环,其中在检查条件之前执行循环体。
do…while循环的语法如下:
do{ // Statement(s); }while(test_condition);
for循环
for循环也是一种入口控制循环,其中元素(初始化、测试条件和增量)放在一起,在for关键字的括号内形成for循环。
for循环的语法如下:
for(initialization ; test condition ; increment){ // Statement (s); }
跳转语句
跳转语句用于将程序的流程从一个地方转移到另一个地方。以下是C语言中的跳转语句:
- goto语句
- break语句
- continue语句
goto语句
goto语句将程序的控制转移到特定的标签。你需要定义一个后跟冒号(:)的标签。goto语句可以向上或向下转移程序的流程。
goto语句的语法如下:
标签名
//Statement(s) if(test_condition){ goto label_name; }
break语句
break语句可以与循环和switch语句一起使用。break语句终止循环执行并将程序的控制转移到循环体之外。
break语句的语法如下:
while(condition1){ . . . . . . if(condition2) break; . . . . . . }
continue语句
continue语句用于跳过当前迭代中循环内其余语句的执行,并将其转移到下一个循环迭代。
continue语句的语法如下:
while (expr){ . . . . . . if (condition) continue; . . . }
用户自定义函数
用户自定义函数由用户定义,用于执行特定任务以实现代码可重用性和模块化。
用户自定义函数示例
#include <stdio.h> // Function declaration int add(int, int); // Function definition int add(int a, int b) { return (a + b); } int main() { int num1 = 10, num2 = 10; int res_add; // Calling the function res_add = add(num1, num2); // Printing the results printf("Addition is : %d\n", res_add); return 0; }
输出
Addition is : 20
数组
数组是相似数据类型的多个数据项的集合,它们存储在连续的内存位置。数据项可以是基本数据类型(int、float、char),也可以是用户定义的类型,例如结构体或指针可以存储在数组中。
C语言数组可以分为两种类型:
- 一维数组 - 一维数组是相同数据类型的单个数据项列表。
- 多维数组 - 多维数组,例如二维数组,是数组的数组。
数组语法
以下是不同类型数组声明的语法:
type array_name [size1]; // One-dimensional array type array_name [size1][size2]; // Two-dimensional arrays type array_name [size1][size2][size3]; // Three-dimensional arrays
一维数组示例
#include <stdio.h> int main(){ int numbers[5] = {10, 20, 30, 40, 50}; int i; // loop counter // Printing array elements printf("The array elements are : "); for (i = 0; i < 5; i++) { printf("%d ", numbers[i]); } return 0; }
输出
The array elements are : 10 20 30 40 50
二维数组示例
#include <stdio.h> int main () { /* an array with 5 rows and 2 columns*/ int a[5][2] = { {0,0}, {1,2}, {2,4}, {3,6},{4,8}}; int i, j; /* output each array element's value */ for ( i = 0; i < 5; i++ ) { for ( j = 0; j < 2; j++ ) { printf("a[%d][%d] = %d\n", i,j, a[i][j] ); } } return 0; }
输出
a[0][0] = 0 a[0][1] = 0 a[1][0] = 1 a[1][1] = 2 a[2][0] = 2 a[2][1] = 4 a[3][0] = 3 a[3][1] = 6 a[4][0] = 4 a[4][1] = 8
字符串
C语言字符串是一系列字符,即char数据类型的数组,以“空字符”(用'\0'表示)结尾。要使用scanf()和printf()函数读取和打印字符串,你必须使用“%s”格式说明符。
字符串声明
char string_name[size];
读取字符串
scanf("%s", string_name);
打印字符串
printf("%s", string_name);
C语言字符串示例
#include <stdio.h> int main() { char name[20]; printf("Enter a name: "); scanf("%s", name); printf("You entered: %s", name); return 0; }
字符串函数
C标准库string.h提供各种函数来处理字符串。以下是C语言字符串函数的列表:
序号 | 函数 | 描述 |
---|---|---|
1 | char *strcat | 将src指向的字符串附加到dest指向的字符串的末尾。 |
2 | char *strncat | 将src指向的字符串附加到dest指向的字符串的末尾,最多n个字符长。 |
3 | char *strchr( | 在参数str指向的字符串中搜索字符c(无符号字符)的第一次出现。 |
4 | int strcmp | 将str1指向的字符串与str2指向的字符串进行比较。 |
5 | int strncmp | 最多比较str1和str2的前n个字节。 |
6 | int strcoll | 比较字符串str1和str2。结果取决于位置的LC_COLLATE设置。 |
7 | char *strcpy | 将src指向的字符串复制到dest。 |
8 | char *strncpy | 最多将src指向的字符串中的n个字符复制到dest。 |
9 | size_t strcspn | 计算str1的初始段的长度,该段完全由str2中不存在的字符组成。 |
10 | char *strerror | 在一个内部数组中搜索错误号errnum,并返回指向错误消息字符串的指针。 |
11 | size_t strlen | 计算字符串str的长度,直到但不包括终止空字符。 |
12 | char *strpbrk | 在字符串str1中查找与str2中指定的任何字符匹配的第一个字符。 |
13 | char *strrchr | 在参数str指向的字符串中搜索字符c(无符号字符)的最后一次出现。 |
14 | size_t strspn | 计算str1的初始段的长度,该段完全由str2中的字符组成。 |
15 | char *strstr | 查找出现在字符串haystack中的整个字符串needle(不包括终止空字符)的第一次出现。 |
16 | char *strtok | 将字符串str分解成一系列由delim分隔的标记。 |
17 | size_t strxfrm | 将字符串src的前n个字符转换为当前区域设置,并将它们放在字符串dest中。 |
结构体
C语言结构体是不同数据类型的集合。结构体被认为是用户定义的数据类型,你可以在其中组合不同数据类型的数据项。
结构体声明语法
struct struct_name { type1 item1; type2 item2; . . }structure_variable;
结构体示例
#include <stdio.h> struct book{ char title[10]; char author[20]; double price; int pages; }; int main(){ struct book book1 = {"Learn C", "Dennis Ritchie", 675.50, 325}; printf("Title: %s \n", book1.title); printf("Author: %s \n", book1.author); printf("Price: %lf\n", book1.price); printf("Pages: %d \n", book1.pages); printf("Size of book struct: %d", sizeof(struct book)); return 0; }
输出
Title: Learn C Author: Dennis Ritchie Price: 675.500000 Pages: 325 Size of book struct: 48
联合体
C语言联合体是一种用户定义的数据类型,允许在同一个内存位置存储不同数据类型的数据项集合。
联合体声明语法
union [union tag]{ member definition; member definition; ... member definition; } [one or more union variables];
联合体示例
#include <stdio.h> union Data{ int i; float f; }; int main(){ union Data data; data.i = 10; data.f = 220.5; printf("data.i: %d \n", data.i); printf("data.f: %f \n", data.f); return 0; }
输出
data.i: 1130135552 data.f: 220.500000
枚举 (enums)
C语言枚举 (enum)是一种枚举数据类型,它由一组整型常量组成。
枚举声明语法
enum myenum {val1, val2, val3, val4};
枚举示例
#include <stdio.h> enum status_codes { OKAY = 1, CANCEL = 0, ALERT = 2 }; int main() { // Printing values printf("OKAY = %d\n", OKAY); printf("CANCEL = %d\n", CANCEL); printf("ALERT = %d\n", ALERT); return 0; }
输出
OKAY = 1 CANCEL = 0 ALERT = 2
指针
C语言指针是派生数据类型,用于存储另一个变量的地址,也可以用于访问和操作存储在该位置的变量数据。
指针声明语法
data_type *pointer_name;
指针初始化语法
如果你声明了一个指针,以下是使用另一个变量的地址初始化指针的语法:
pointer_name = &variable_name;
指针示例
#include <stdio.h> int main() { int x = 10; // Pointer declaration and initialization int * ptr = & x; // Printing the current value printf("Value of x = %d\n", * ptr); // Changing the value * ptr = 20; // Printing the updated value printf("Value of x = %d\n", * ptr); return 0; }
输出
Value of x = 10 Value of x = 20
指针类型
C语言中有各种类型的指针。它们是:
动态内存分配
变量的内存是在编译时声明的。C语言提供一些动态内存分配函数,允许在运行时为变量分配内存。
动态内存分配函数:
- malloc()
- calloc()
- realloc()
- free()
malloc()函数
malloc()函数分配请求的内存(指定大小的块数),并返回指向它的指针。
malloc() 函数的语法如下:
malloc (size_t size); calloc() Function
calloc() 函数
calloc() 函数分配请求的内存(指定大小的块数),并返回 void 指针。calloc() 函数将分配的内存设置为零。
calloc() 函数的语法如下:
void *calloc(size_t nitems, size_t size)
realloc() 函数
realloc() 函数尝试重新调整由指针指向的内存块的大小,该指针之前已通过调用 malloc() 或 calloc() 函数分配。
realloc() 函数的语法如下:
void *calloc(size_t nitems, size_t size)
free() 函数
free() 函数释放之前通过调用 calloc()、malloc() 或 realloc() 分配的内存。
realloc() 函数的语法如下:
void *calloc(size_t nitems, size_t size)
文件处理
文件处理指的是对文件执行各种操作,例如创建、写入、读取、删除、移动、重命名文件等。C 语言提供了各种文件处理函数。
文件操作
以下是可以使用 C 语言文件处理函数对文件执行的操作:
- 创建新文件
- 打开现有文件
- 向文件写入数据
- 向文件追加数据
- 从文件读取数据
- 重命名文件
- 删除文件
- 关闭文件
文件处理函数
以下是 C 语言中文件处理函数的列表:
函数 | 描述 |
---|---|
fopen() | 创建并以各种模式打开文件。 |
fclose() | 关闭文件。 |
fputc(),fputs(),fprintf() | 向文件写入数据。 |
fgetc(),fgets(),fscanf() | 从文件读取数据。 |
fwrite(),fread() | 向二进制文件写入和读取数据。 |
rename() | 重命名文件。 |
remove() | 删除文件。 |
文件处理示例
这是一个 C 语言文件处理的示例:
#include <stdio.h> #include <stdlib.h> int main() { FILE *file; char file_name[] = "my_file.txt"; char write_data[100] = "Tutorials Point"; char read_data[100]; // Opening file in write mode file = fopen("file_name.txt", "w"); if (file == NULL) { printf("Error\n"); return 1; } // Writing to the file fprintf(file, "%s", write_data); // Closing the file fclose(file); // Again, opening the file in read mode file = fopen("file_name.txt", "r"); if (file == NULL) { printf("Error.\n"); return 1; } // Reading data from the file if (fgets(read_data, 100, file) != NULL) { // Printing it on the screen printf("File's data:\n%s\n", read_data); } fclose(file); return 0; }
输出
File's data: Tutorials Point
预处理器指令
预处理器指令是预编译的一部分,以井号 (#) 字符开头。这些指令指示编译器在开始编译过程之前展开 include 并扩展代码。
以下是预处理器指令的列表:
指令 | 描述 |
---|---|
#define | 替换预处理器宏。 |
#include | 插入来自另一个文件的特定头文件。 |
#undef | 取消定义预处理器宏。 |
#ifdef | 如果定义了此宏,则返回 true。 |
#ifndef | 如果未定义此宏,则返回 true。 |
#if | 测试编译时条件是否为 true。 |
#else | #if 的替代方案。 |
#elif | #else 和 #if 合并成一条语句。 |
#endif | 结束预处理器条件。 |
#error | 在 stderr 上打印错误消息。 |
#pragma | 使用标准化方法向编译器发出特殊命令。 |
预处理器指令示例
这是一个 #define 的示例,它是 C 语言中的一种预处理器指令:
#define MAX_ARRAY_LENGTH 20
标准库
以下是常用库(C 头文件)的列表:
头文件 | 用途 |
---|---|
stdio.h | 提供标准输入和输出函数。 |
string.h | 提供各种字符串操作函数。 |
math.h | 提供数学运算函数。 |
stdlib.h | 提供各种用于内存分配、类型转换等的实用函数。 |
time.h | 提供与日期时间相关的函数。 |