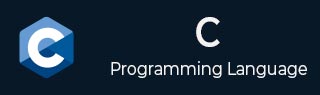
- C语言编程教程
- C语言 - 首页
- C语言基础
- C语言 - 概述
- C语言 - 特性
- C语言 - 历史
- C语言 - 环境搭建
- C语言 - 程序结构
- C语言 - Hello World
- C语言 - 编译过程
- C语言 - 注释
- C语言 - 词法单元
- C语言 - 关键字
- C语言 - 标识符
- C语言 - 用户输入
- C语言 - 基本语法
- C语言 - 数据类型
- C语言 - 变量
- C语言 - 整数提升
- C语言 - 类型转换
- C语言 - 类型强制转换
- C语言 - 布尔值
- C语言中的常量和字面量
- C语言 - 常量
- C语言 - 字面量
- C语言 - 转义序列
- C语言 - 格式说明符
- C语言中的运算符
- C语言 - 运算符
- C语言 - 算术运算符
- C语言 - 关系运算符
- C语言 - 逻辑运算符
- C语言 - 位运算符
- C语言 - 赋值运算符
- C语言 - 一元运算符
- C语言 - 自增和自减运算符
- C语言 - 三元运算符
- C语言 - sizeof 运算符
- C语言 - 运算符优先级
- C语言 - 其他运算符
- C语言中的决策
- C语言 - 决策
- C语言 - if 语句
- C语言 - if...else 语句
- C语言 - 嵌套 if 语句
- C语言 - switch 语句
- C语言 - 嵌套 switch 语句
- C语言中的循环
- C语言 - 循环
- C语言 - while 循环
- C语言 - for 循环
- C语言 - do...while 循环
- C语言 - 嵌套循环
- C语言 - 无限循环
- C语言 - break 语句
- C语言 - continue 语句
- C语言 - goto 语句
- C语言中的函数
- C语言 - 函数
- C语言 - 主函数
- C语言 - 按值调用函数
- C语言 - 按引用调用函数
- C语言 - 嵌套函数
- C语言 - 可变参数函数
- C语言 - 用户自定义函数
- C语言 - 回调函数
- C语言 - return 语句
- C语言 - 递归
- C语言中的作用域规则
- C语言 - 作用域规则
- C语言 - 静态变量
- C语言 - 全局变量
- C语言中的数组
- C语言 - 数组
- C语言 - 数组的特性
- C语言 - 多维数组
- C语言 - 将数组传递给函数
- C语言 - 从函数返回数组
- C语言 - 可变长度数组
- C语言中的指针
- C语言 - 指针
- C语言 - 指针和数组
- C语言 - 指针的应用
- C语言 - 指针运算
- C语言 - 指针数组
- C语言 - 指向指针的指针
- C语言 - 将指针传递给函数
- C语言 - 从函数返回指针
- C语言 - 函数指针
- C语言 - 指向数组的指针
- C语言 - 指向结构体的指针
- C语言 - 指针链
- C语言 - 指针与数组的区别
- C语言 - 字符指针和函数
- C语言 - NULL 指针
- C语言 - void 指针
- C语言 - 野指针
- C语言 - 解引用指针
- C语言 - 近、远和巨型指针
- C语言 - 指针数组的初始化
- C语言 - 指针与多维数组的区别
- C语言中的字符串
- C语言 - 字符串
- C语言 - 字符串数组
- C语言 - 特殊字符
- C语言结构体和联合体
- C语言 - 结构体
- C语言 - 结构体和函数
- C语言 - 结构体数组
- C语言 - 自引用结构体
- C语言 - 查找表
- C语言 - 点 (.) 运算符
- C语言 - 枚举 (enum)
- C语言 - 结构体填充和打包
- C语言 - 嵌套结构体
- C语言 - 匿名结构体和联合体
- C语言 - 联合体
- C语言 - 位域
- C语言 - Typedef
- C语言中的文件处理
- C语言 - 输入与输出
- C语言 - 文件 I/O (文件处理)
- C语言预处理器
- C语言 - 预处理器
- C语言 - 预处理指令
- C语言 - 预处理器运算符
- C语言 - 宏
- C语言 - 头文件
- C语言中的内存管理
- C语言 - 内存管理
- C语言 - 内存地址
- C语言 - 存储类
- 其他主题
- C语言 - 错误处理
- C语言 - 可变参数
- C语言 - 命令执行
- C语言 - 数学函数
- C语言 - static 关键字
- C语言 - 随机数生成
- C语言 - 命令行参数
- C语言编程资源
- C语言 - 问答
- C语言 - 快速指南
- C语言 - 速查表
- C语言 - 有用资源
- C语言 - 讨论
C语言 - 数组的特性
数组是C语言中非常重要的数据结构。在C程序中使用数组可以更容易地处理大量数据。由于数组的许多特性,数组比单个变量具有许多优点。数组的大多数重要特性都源于其组成——数组是相同数据类型值的集合,并且位于连续的内存块中。
数组的特性在不同的编程语言中可能会有所不同。在C语言中,数组的主要特性如下:
- 相同数据类型的集合
- 连续内存分配
- 固定大小
- 长度取决于类型
- 索引
- 指针关系
- 下界和上界
- 多维数组
- 复杂数据结构的实现
让我们详细讨论每个特性。
相同数据类型的集合
数组的所有元素都必须是相同的数据类型。这确保了对数据的访问和操作的一致性。
如果数组声明如下:
int arr[] = {50, 67.55, "hello", 21};
编译器会发出警告:
initialization of 'int' from 'char *' makes integer from pointer without a cast [-Wint-conversion]|
连续内存分配
数组的所有元素都存储在连续的内存位置,这意味着它们占据彼此相邻的内存块。这允许高效的随机访问和内存管理。
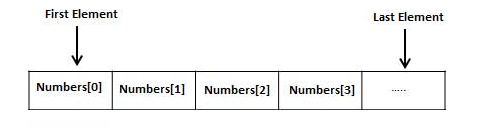
固定大小
数组的大小在声明时是固定的,并且在程序执行期间不能更改。这意味着您需要预先知道所需的最大元素数量。在C语言中,数组的大小不能用变量来定义。
//这是允许的
#define SIZE = 10 int arr[SIZE];
//这也是允许的
const SIZE = 10; int arr[SIZE];
//这是不允许的
int SIZE = 10; int arr[SIZE];
//大小必须是整数。这将导致错误
float num[10.5] = {50, 55, 67, 73, 45, 21, 39, 70, 49, 51};
长度取决于类型
由于数组可以存储所有相同类型的元素,因此它占用的总内存取决于数据类型。
示例
#include<stdio.h> int main() { int num[10] = {50, 55, 67, 73, 45, 21, 39, 70, 49, 51}; int size = sizeof(num) / sizeof(int); printf("element at lower bound num[0]: %d \n", num[0]); printf("at upper bound: %d byte \n", num[size-1]); printf("length of int array: %ld \n", sizeof(num)); double nm[10] = {50, 55, 67, 73, 45, 21, 39, 70, 49, 51}; size = sizeof(nm) / sizeof(double); printf("element at lower bound nm[0]: %f \n", nm[0]); printf("element at upper bound: %f \n", nm[size-1]); printf("byte length of double array: %ld \n", sizeof(nm)); return 0; }
输出
element at lower bound num[0]: 50 at upper bound: 51 byte length of int array: 40 element at lower bound nm[0]: 50.000000 element at upper bound: 51.000000 byte length of double array: 80
索引
数组中的每个元素都有一个唯一的索引,从0开始。可以使用方括号中包含的索引访问单个元素。通常,使用for循环遍历数组的长度,并将循环变量用作索引。
示例
#include <stdio.h> int main() { int a[] = {1,2,3,4,5}; int i; for (i=0; i<4; i++){ printf("a[%d]: %d \n", i, a[i]); } return 0; }
指针关系
数组的名称等效于指向其第一个元素的常量指针。这允许您在某些情况下互换使用数组名称和指针。
示例
#include <stdio.h> int main() { int num[10] = {50, 55, 67, 73, 45, 21, 39, 70, 49, 51}; printf("num[0]: %d Address of 0th element: %d\n", num[0], &num[0]); printf("Address of array: %d", num); return 0; }
输出
num[0]: 50 Address of 0th element: 6422000 Address of array: 6422000
下界和上界
数组中的每个元素都由一个从0开始的索引标识。数组的下界是其第一个元素的索引,始终为0。数组的最后一个元素的索引为数组大小减1。
示例
#include <stdio.h> int main() { int num[10] = {50, 55, 67, 73, 45, 21, 39, 70, 49, 51}; int size = sizeof(num) / sizeof(int); printf("element at lower bound num[0]: %d at upper bound: %d Size of array: %d", num[0], num[size-1], size); return 0; }
输出
element at lower bound num[0]: 50 at upper bound: 51 Size of array: 10
多维数组
如果数组声明时方括号中只有一个大小值,则称为一维数组。在一维数组中,每个元素都由其索引或下标标识。在C语言中,您可以声明多个索引来模拟二维、三维或多维数组。
示例
例如,以下是二维数组的示例:
int a[3][3] = { {1, 2, 3}, {11, 22, 33}, {111, 222, 333}};
您可以将一维数组视为列表,将二维数组视为表格或矩阵。理论上,数组的维数没有限制,但在实践中,二维数组用于电子表格、数据库等的設計。
复杂数据结构的实现
我们可以在结构数据类型的构造中使用数组来实现堆栈、链表和树等数据结构。
示例
typedef struct stack { int top; int arr[10]; } Stack;
因此,数组是程序员武器库中的重要工具,因为它可以用于不同的应用程序。C语言中的数组概念被许多后续的编程语言所实现,例如C++、C#、Java等。