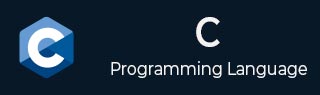
- C 编程教程
- C - 首页
- C 语言基础
- C - 概述
- C - 特性
- C - 历史
- C - 环境设置
- C - 程序结构
- C - Hello World
- C - 编译过程
- C - 注释
- C - 词法单元
- C - 关键字
- C - 标识符
- C - 用户输入
- C - 基本语法
- C - 数据类型
- C - 变量
- C - 整数提升
- C - 类型转换
- C - 类型强制转换
- C - 布尔类型
- C 语言中的常量和字面量
- C - 常量
- C - 字面量
- C - 转义序列
- C - 格式说明符
- C 语言中的运算符
- C - 运算符
- C - 算术运算符
- C - 关系运算符
- C - 逻辑运算符
- C - 位运算符
- C - 赋值运算符
- C - 一元运算符
- C - 自增和自减运算符
- C - 三元运算符
- C - sizeof 运算符
- C - 运算符优先级
- C - 其他运算符
- C 语言中的决策
- C - 决策
- C - if 语句
- C - if...else 语句
- C - 嵌套 if 语句
- C - switch 语句
- C - 嵌套 switch 语句
- C 语言中的循环
- C - 循环
- C - while 循环
- C - for 循环
- C - do...while 循环
- C - 嵌套循环
- C - 无限循环
- C - break 语句
- C - continue 语句
- C - goto 语句
- C 语言中的函数
- C - 函数
- C - 主函数
- C - 按值调用函数
- C - 按引用调用函数
- C - 嵌套函数
- C - 可变参数函数
- C - 用户自定义函数
- C - 回调函数
- C - 返回语句
- C - 递归
- C 语言中的作用域规则
- C - 作用域规则
- C - 静态变量
- C - 全局变量
- C 语言中的数组
- C - 数组
- C - 数组的特性
- C - 多维数组
- C - 将数组传递给函数
- C - 从函数返回数组
- C - 可变长数组
- C 语言中的指针
- C - 指针
- C - 指针和数组
- C - 指针的应用
- C - 指针运算
- C - 指针数组
- C - 指针到指针
- C - 将指针传递给函数
- C - 从函数返回指针
- C - 函数指针
- C - 指向数组的指针
- C - 指向结构体的指针
- C - 指针链
- C - 指针与数组
- C - 字符指针和函数
- C - 空指针
- C - void 指针
- C - 野指针
- C - 解引用指针
- C - 近、远和巨型指针
- C - 指针数组的初始化
- C - 指针与多维数组
- C 语言中的字符串
- C - 字符串
- C - 字符串数组
- C - 特殊字符
- C 结构体和联合体
- C - 结构体
- C - 结构体和函数
- C - 结构体数组
- C - 自引用结构体
- C - 查找表
- C - 点 (.) 运算符
- C - 枚举 (或 enum)
- C - 结构体填充和打包
- C - 嵌套结构体
- C - 匿名结构体和联合体
- C - 联合体
- C - 位域
- C - Typedef
- C 语言中的文件处理
- C - 输入和输出
- C - 文件 I/O (文件处理)
- C 预处理器
- C - 预处理器
- C - 编译指示
- C - 预处理器运算符
- C - 宏
- C - 头文件
- C 语言中的内存管理
- C - 内存管理
- C - 内存地址
- C - 存储类
- 其他主题
- C - 错误处理
- C - 可变参数
- C - 命令执行
- C - 数学函数
- C - static 关键字
- C - 随机数生成
- C - 命令行参数
- C 编程资源
- C - 问答
- C - 快速指南
- C - 速查表
- C - 有用资源
- C - 讨论
C - if-else 语句
if-else 语句是 C 语言中经常使用的决策语句之一。if-else 语句在条件不满足时提供了一条备选路径。
else 关键字帮助您提供当 if 语句中的布尔表达式结果为假时要采取的备选操作方案。else 关键字的使用是可选的;您可以选择是否使用它。
if-else 语句的语法
以下是 if-else 子句的语法:
if (Boolean expr){ Expression; . . . } else{ Expression; . . . }
C 编译器 会评估条件,如果条件为真,则执行 if 语句后面的语句或语句块。
如果编程逻辑需要计算机在条件为假时执行其他指令,则将其作为 else 子句的一部分。
if 语句后面可以跟一个可选的 else 语句,当布尔表达式为假时执行该语句。
if-else 语句的流程图
以下流程图表示 if-else 子句在 C 语言中的工作方式:
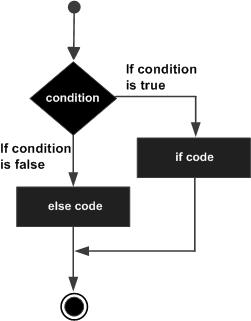
请注意,如果 if 和 else 子句中有多个语句,则需要花括号。例如,在以下代码中,我们不需要花括号。
if (marks<50) printf("Result: Fail\n"); else printf("Result: Pass\n");
但是,当 if 或 else 部分有多个语句时,您需要告诉编译器将它们视为复合语句。
C if-else 语句示例
示例:使用 if-else 语句计算税款
在下面给出的代码中,计算了员工收入的税款。如果收入低于 10000,则税率为 10%。对于收入超过 10000 的部分,超出部分的税率为 15%。
#include <stdio.h> int main() { int income = 5000; float tax; printf("Income: %d\n", income); if (income<10000){ tax = (float)(income * 10 / 100); printf("tax: %f \n", tax); } else { tax= (float)(1000 + (income-10000) * 15 / 100); printf("tax: %f", tax); } }
输出
运行代码并检查其输出:
Income: 5000 tax: 500.000000
将 income 变量设置为 15000,然后再次运行程序。
Income: 15000 tax: 1750.000000
示例:使用 if-else 语句检查数字
以下程序检查 char 变量是否存储的是数字字符还是非数字字符。
#include <stdio.h> int main() { char ch='7'; if (ch>=48 && ch<=57){ printf("The character is a digit."); } else{ printf("The character is not a digit."); } return 0; }
输出
运行代码并检查其输出:
The character is a digit.
将任何其他字符(例如 "*")赋给 "ch" 并查看结果。
The character is not a digit.
示例:没有花括号的 if-else 语句
考虑以下代码。它旨在在金额大于 100 时计算 10% 的折扣,否则不打折。
#include <stdio.h> int main() { int amount = 50; float discount; printf("Amount: %d\n", amount); if (amount >= 100) discount = amount * 10 / 100; printf("Discount: %f \n", discount); else printf("Discount not applicable\n"); return 0; }
输出
程序在编译期间显示以下错误:
error: 'else' without a previous 'if'
编译器将执行 if 子句后的第一个语句,并假设由于下一个语句不是 else(无论如何它是可选的),因此后续的 printf() 语句是无条件的。但是,下一个 else 与任何 if 语句都没有关联,因此出现错误。
示例:没有花括号的 if-else 语句
也考虑以下代码:
#include <stdio.h> int main() { int amount = 50; float discount, nett; printf("Amount: %d\n", amount); if (amount<100) printf("Discount not applicable\n"); else printf("Discount applicable"); discount = amount*10/100; nett = amount - discount; printf("Discount: %f Net payable: %f", discount, nett); return 0; }
输出
该代码没有给出任何编译错误,但给出了不正确的输出:
Amount: 50 Discount not applicable Discount: 5.000000 Net payable: 45.000000
它产生了不正确的输出,因为编译器假设 else 子句中只有一个语句,其余语句是无条件的。
以上两个代码示例强调了一个事实,即当 if 或 else 部分有多个语句时,必须将它们放在花括号中。
为了安全起见,即使对于单个语句,最好也使用花括号。实际上,它提高了代码的可读性。
上面问题的正确解决方案如下所示:
if (amount >= 100){ discount = amount * 10 / 100; printf("Discount: %f \n", discount); } else { printf("Discount not applicable\n"); }
C 语言中的 else-if 语句
C 语言也允许您在程序中使用 else-if。让我们看看您可能需要在什么情况下使用 else-if 子句。
假设您遇到如下情况。如果某个条件为真,则运行其后的指定代码块。如果不是,则改为运行下一个代码块。但是,如果以上条件都不满足,并且所有其他情况都失败,则最终运行另一个代码块。在这种情况下,您将使用 else-if 子句。
else-if 语句的语法
以下是 else-if 子句的语法:
if (condition){ // if the condition is true, // then run this code } else if(another_condition){ // if the above condition was false // and this condition is true, // then run the code in this block } else{ // if both the above conditions are false, // then run this code }
else-if 语句的示例
请查看以下示例:
#include <stdio.h> int main(void) { int age = 15; if (age < 18) { printf("You need to be over 18 years old to continue\n"); }else if (age < 21) { printf("You need to be over 21\n"); } else { printf("You are over 18 and older than 21 so you can continue \n"); } }
输出
运行代码并检查其输出:
You need to be over 18 years old to continue
现在,为变量 "age" 提供不同的值,然后再次运行代码。如果提供的值小于 18,您将获得不同的输出。