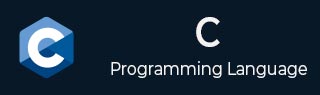
- C编程教程
- C - 首页
- C语言基础
- C - 概述
- C - 特性
- C - 历史
- C - 环境搭建
- C - 程序结构
- C - Hello World
- C - 编译过程
- C - 注释
- C - 词法单元
- C - 关键字
- C - 标识符
- C - 用户输入
- C - 基本语法
- C - 数据类型
- C - 变量
- C - 整数提升
- C - 类型转换
- C - 类型强制转换
- C - 布尔值
- C语言中的常量和字面量
- C - 常量
- C - 字面量
- C - 转义序列
- C - 格式说明符
- C语言中的运算符
- C - 运算符
- C - 算术运算符
- C - 关系运算符
- C - 逻辑运算符
- C - 位运算符
- C - 赋值运算符
- C - 一元运算符
- C - 自增和自减运算符
- C - 三元运算符
- C - sizeof 运算符
- C - 运算符优先级
- C - 其他运算符
- C语言中的决策
- C - 决策
- C - if 语句
- C - if...else 语句
- C - 嵌套 if 语句
- C - switch 语句
- C - 嵌套 switch 语句
- C语言中的循环
- C - 循环
- C - while 循环
- C - for 循环
- C - do...while 循环
- C - 嵌套循环
- C - 无限循环
- C - break 语句
- C - continue 语句
- C - goto 语句
- C语言中的函数
- C - 函数
- C - 主函数
- C - 按值调用函数
- C - 按引用调用函数
- C - 嵌套函数
- C - 可变参数函数
- C - 用户定义函数
- C - 回调函数
- C - 返回语句
- C - 递归
- C语言中的作用域规则
- C - 作用域规则
- C - 静态变量
- C - 全局变量
- C语言中的数组
- C - 数组
- C - 数组的特性
- C - 多维数组
- C - 将数组传递给函数
- C - 从函数返回数组
- C - 变长数组
- C语言中的指针
- C - 指针
- C - 指针和数组
- C - 指针的应用
- C - 指针运算
- C - 指针数组
- C - 指针到指针
- C - 将指针传递给函数
- C - 从函数返回指针
- C - 函数指针
- C - 指向数组的指针
- C - 指向结构体的指针
- C - 指针链
- C - 指针与数组
- C - 字符指针和函数
- C - 空指针
- C - void 指针
- C - 野指针
- C - 解引用指针
- C - 近指针、远指针和巨指针
- C - 指针数组的初始化
- C - 指针与多维数组
- C语言中的字符串
- C - 字符串
- C - 字符串数组
- C - 特殊字符
- C语言中的结构体和联合体
- C - 结构体
- C - 结构体和函数
- C - 结构体数组
- C - 自引用结构体
- C - 查找表
- C - 点(.)运算符
- C - 枚举(或 enum)
- C - 结构体填充和打包
- C - 嵌套结构体
- C - 匿名结构体和联合体
- C - 联合体
- C - 位域
- C - Typedef
- C语言中的文件处理
- C - 输入和输出
- C - 文件I/O(文件处理)
- C预处理器
- C - 预处理器
- C - 编译指示
- C - 预处理器运算符
- C - 宏
- C - 头文件
- C语言中的内存管理
- C - 内存管理
- C - 内存地址
- C - 存储类
- 其他主题
- C - 错误处理
- C - 可变参数
- C - 命令执行
- C - 数学函数
- C - static 关键字
- C - 随机数生成
- C - 命令行参数
- C编程资源
- C - 问答
- C - 快速指南
- C - 速查表
- C - 有用资源
- C - 讨论
C语言中的数学函数
C 数学函数
C语言提供各种函数来对数字执行数学运算,例如查找三角函数值、计算对数和指数、舍入数字等。要在C程序中使用这些**数学函数**,您需要包含math.h 头文件。
我们将数学函数分为以下几类:
三角函数
math.h 库定义了函数sin()、cos() 和 tan() - 返回相应的三角函数值,即给定角度的正弦、余弦和正切。
这些函数返回给定双精度类型(表示以弧度表示的角度)的相应比率。所有函数都返回双精度值。
double sin(double x) double cos(double x) double tan(double x)
对于所有上述函数,参数“x”都是以弧度表示的角度。
示例
以下示例演示了如何在C中使用三角函数:
#include <stdio.h> #include <math.h> #define PI 3.14159265 int main() { double x, sn, cs, tn, val; x = 45.0; val = PI / 180; sn = sin(x*val); cs = cos(x*val); tn = tan(x*val); printf("sin(%f) : %f\n", x, sn); printf("cos(%f) : %f\n", x, cs); printf("tan(%f) : %f\n", x, tn); return(0); }
输出
运行此代码时,将产生以下输出:
sin(45.000000) : 0.707107 cos(45.000000) : 0.707107 tan(45.000000) : 1.000000
反三角函数
math.h 库还包括反三角函数,也称为反正弦函数或反三角函数。它们是基本三角函数的反函数。例如,asin(x) 等价于 $\mathrm{sin^{-1}(x)}$。其他反函数有 acos()、atan() 和 atan2()。
以下asin() 函数 返回“x”在区间 [-pi/2, +pi/2] 弧度内的反正弦值:
double asin(double x)
以下acos() 函数 返回“x”在区间 [0, pi] 弧度内的主值反余弦值:
double acos(double x)
以下atan() 函数 返回“x”在区间 [-pi/2, +pi/2] 弧度内的主值反正切值。
double atan(double x)
示例 1
以下示例演示了如何在C程序中使用反三角函数:
#include <stdio.h> #include <math.h> #define PI 3.14159265 int main() { double x, asn, acs, atn, val; x = 0.9; val = 180/PI; asn = asin(x); acs = acos(x); atn = atan(x); printf("asin(%f) : %f in radians\n", x, asn); printf("acos(%f) : %f in radians\n", x, acs); printf("atan(%f) : %f in radians\n", x, atn); asn = (asn * 180) / PI; acs = (acs * 180) / PI; atn = (atn * 180) / PI; printf("asin(%f) : %f in degrees\n", x, asn); printf("acos(%f) : %f in degrees\n", x, acs); printf("atan(%f) : %f in degrees\n", x, atn); return(0); }
输出
运行此代码时,将产生以下输出:
asin(0.900000) : 1.119770 in radians acos(0.900000) : 0.451027 in radians atan(0.900000) : 0.732815 in radians asin(0.900000) : 64.158067 in degrees acos(0.900000) : 25.841933 in degrees atan(0.900000) : 41.987213 in degrees
The atan2() function returns the arc tangent in radians of "y/x" based on the signs of both values to determine the correct quadrant.
double atan2(double y, double x)
此函数返回“y / x”在区间 [-pi, +pi] 弧度内的主值反正切值。
示例 2
请查看以下示例:
#include <stdio.h> #include <math.h> #define PI 3.14159265 int main() { double x, y, ret, val; x = -7.0; y = 7.0; val = 180.0 / PI; ret = atan2 (y,x) * val; printf("The arc tangent of x = %lf, y = %lf ", x, y); printf("is %lf degrees\n", ret); return(0); }
输出
运行代码并检查其输出:
The arc tangent of x = -7.000000, y = 7.000000 is 135.000000 degrees
双曲函数
在数学中,双曲函数类似于三角函数,但使用双曲线而不是圆来定义。math.h 头文件提供sinh()、cosh() 和tanh() 函数。
double sinh(double x)
此函数返回 x 的双曲正弦值。
double cosh(double x)
此函数返回 x 的双曲余弦值。
double tanh(double x)
此函数返回 x 的双曲正切值。
示例
以下示例演示了如何在C程序中使用双曲函数:
#include <stdio.h> #include <math.h> #define PI 3.14159265 int main() { double x,val, sh, ch, th; x = 45; val = PI/180.0 ; sh = sinh(x*val); ch = cosh(x*val); th = tanh(x*val); printf("The sinh(%f) = %lf\n", x, sh); printf("The cosh(%f) = %lf\n", x, ch); printf("The tanh(%f) = %lf\n", x, th); return(0); }
输出
运行代码并检查其输出:
The sinh(45.000000) = 0.868671 The cosh(45.000000) = 1.324609 The tanh(45.000000) = 0.655794
指数和对数函数
"math.h" 库包含以下与指数和对数相关的函数:
exp() 函数:它返回 e 的 x 次幂的值。(e 的值 - 欧拉数 - 约为 2.718)
double exp(double x)
log() 函数:它返回“x”的自然对数(以 e 为底的对数)。
double log(double x)
请注意,对数函数等价于指数函数的反函数。
log10() 函数:它返回“x”的常用对数(以 10 为底的对数)。
double log10(double x)
示例
以下示例演示了如何在C程序中使用指数和对数函数:
#include <stdio.h> #include <math.h> #define PI 3.14159265 int main () { double x = 2; double e, ln, ls; e = exp(2); ln = log(e); printf("exp(%f): %f log(%f): %f\n",x, e, e, ln); ln = log(x); printf("log(%f): %f\n",x,ln); ls = log10(x); printf("log10(%f): %f\n",x,ls); return(0); }
输出
运行此代码时,将产生以下输出:
exp(2.000000): 7.389056 log(7.389056): 2.000000 log(2.000000): 0.693147 log10(2.000000): 0.301030
浮点数函数
The frexp() and ldexp() functions are used for floating-point manipulation.
frexp() 函数
"math.h" 头文件还包含 frexp() 函数。它将浮点数分解为其有效数和指数。
double frexp(double x, int *exponent)
这里,“x”是要计算的浮点值,而“exponent”是指向 int 对象的指针,其中要存储指数的值。
此函数返回归一化分数。
示例
请查看以下示例:
#include <stdio.h> #include <math.h> int main () { double x = 1024, fraction; int e; fraction = frexp(x, &e); printf("x = %.2lf = %.2lf * 2^%d\n", x, fraction, e); return(0); }
输出
运行代码并检查其输出:
x = 1024.00 = 0.50 * 2^11
ldexp() 函数
ldexp() 函数将有效数和指数组合起来形成一个浮点数。其语法如下:
double ldexp(double x, int exponent)
这里,“x”是表示有效数的浮点值,“exponent”是指数的值。此函数返回 (x * 2 exp)
示例
以下示例演示了如何在C程序中使用此 ldexp() 函数:
#include <stdio.h> #include <math.h> int main () { double x, ret; int n; x = 0.65; n = 3; ret = ldexp(x ,n); printf("%f * 2 %d = %f\n", x, n, ret); return(0); }
输出
运行代码并检查其输出:
0.650000 * 2^3 = 5.200000
幂和平方根函数
The pow() and sqrt() functions are used to calculate the power and square root of the given number.
pow() 函数
此函数返回 x 的 y 次幂,即 xy。
double pow(double x, double y)
sqrt() 函数
返回 x 的平方根。
double sqrt(double x)
sqrt(x) 函数返回的值与 pow(x, 0.5) 相同
示例
以下示例演示了如何在C程序中使用 pow() 和 sqrt() 函数:
#include <stdio.h> #include <math.h> int main() { double x = 9, y=2; printf("Square root of %lf is %lf\n", x, sqrt(x)); printf("Square root of %lf is %lf\n", x, pow(x, 0.5) ); printf("%lf raised to power %lf\n", x, pow(x, y)); return(0); }
输出
运行此代码时,将产生以下输出:
Square root of 9.000000 is 3.000000 Square root of 9.000000 is 3.000000 9.000000 raised to power 81.000000
舍入函数
math.h 库包含ceil()、floor() 和 round() 函数,这些函数对给定的浮点数进行舍入。
ceil() 函数
它返回大于或等于 x 的最小整数。
double ceil(double x)
此函数返回不小于 x 的最小整数值。
floor() 函数
此函数返回小于或等于 x 的最大整数。
double floor(double x)
参数 x:这是浮点值。此函数返回不大于 x 的最大整数值。
round() 函数
此函数用于将作为参数传递给它的双精度、浮点或长双精度值舍入到最接近的整数值。
double round( double x )
返回值是以浮点数表示的最接近的整数。
示例
以下示例演示了如何在C程序中使用舍入函数:
#include <stdio.h> #include <math.h> int main() { float val1, val2, val3, val4; val1 = 1.2; val2 = 1.6; val3 = 2.8; val4 = -2.3; printf ("ceil(%lf) = %.1lf\n", val1, ceil(val1)); printf ("floor(%lf) = %.1lf\n", val2, floor(val2)); printf ("ceil(%lf) = %.1lf\n", val3, ceil(val3)); printf ("floor(%lf) = %.1lf\n", val4, floor(val4)); printf("round(%lf) = %.1lf\n", val1, round(val1)); printf("round(%lf) = %.1lf", val4, round(val4)); return(0); }
输出
运行此代码时,将产生以下输出:
ceil(1.200000) = 2.0 floor(1.600000) = 1.0 ceil(2.800000) = 3.0 floor(-2.300000) = -3.0 round(1.200000) = 1.0 round(-2.300000) = -2.0
模函数
此类别中"math.h" 库包含以下函数
modf() 函数
The modf() function returns the fraction component (part after the decimal), and sets integer to the integer component.
double modf(double x, double *integer)
这里,“x”是浮点值,“integer”是指向一个对象的指针,其中存储整数部分。
此函数返回“x”的小数部分,符号相同。
示例
请查看以下示例:
#include <stdio.h> #include <math.h> int main () { double x, fractpart, intpart; x = 8.123456; fractpart = modf(x, &intpart); printf("Integral part = %lf\n", intpart); printf("Fraction Part = %lf \n", fractpart); return(0); }
输出
运行此代码时,将产生以下输出:
Integral part = 8.000000 Fraction Part = 0.123456
fmod() 函数
The fmod() function returns the remainder of x divided by y.
double fmod(double x, double y)
这里,“x”是分子,“y”是分母。该函数返回“x / y”的余数。
示例
请查看以下示例:
#include <stdio.h> #include <math.h> int main () { float a, b; int c; a = 9.2; b = 3.7; c = 2; printf("Remainder of %f / %d is %lf\n", a, c, fmod(a,c)); printf("Remainder of %f / %f is %lf\n", a, b, fmod(a,b)); return(0); }
输出
运行此代码时,将产生以下输出:
Remainder of 9.200000 / 2 is 1.200000 Remainder of 9.200000 / 3.700000 is 1.800000
请注意,模运算符 (%) 仅适用于整数操作数。