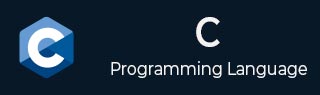
- C编程教程
- C语言 - 首页
- C语言基础
- C语言 - 概述
- C语言 - 特性
- C语言 - 历史
- C语言 - 环境搭建
- C语言 - 程序结构
- C语言 - Hello World
- C语言 - 编译过程
- C语言 - 注释
- C语言 - 词法单元
- C语言 - 关键字
- C语言 - 标识符
- C语言 - 用户输入
- C语言 - 基本语法
- C语言 - 数据类型
- C语言 - 变量
- C语言 - 整数提升
- C语言 - 类型转换
- C语言 - 类型强制转换
- C语言 - 布尔值
- C语言中的常量和字面量
- C语言 - 常量
- C语言 - 字面量
- C语言 - 转义序列
- C语言 - 格式说明符
- C语言中的运算符
- C语言 - 运算符
- C语言 - 算术运算符
- C语言 - 关系运算符
- C语言 - 逻辑运算符
- C语言 - 位运算符
- C语言 - 赋值运算符
- C语言 - 一元运算符
- C语言 - 自增和自减运算符
- C语言 - 三元运算符
- C语言 - sizeof 运算符
- C语言 - 运算符优先级
- C语言 - 其他运算符
- C语言中的决策机制
- C语言 - 决策机制
- C语言 - if 语句
- C语言 - if...else 语句
- C语言 - 嵌套 if 语句
- C语言 - switch 语句
- C语言 - 嵌套 switch 语句
- C语言中的循环
- C语言 - 循环
- C语言 - while 循环
- C语言 - for 循环
- C语言 - do...while 循环
- C语言 - 嵌套循环
- C语言 - 无限循环
- C语言 - break 语句
- C语言 - continue 语句
- C语言 - goto 语句
- C语言中的函数
- C语言 - 函数
- C语言 - 主函数
- C语言 - 按值调用函数
- C语言 - 按引用调用函数
- C语言 - 嵌套函数
- C语言 - 变参函数
- C语言 - 用户自定义函数
- C语言 - 回调函数
- C语言 - 返回语句
- C语言 - 递归
- C语言中的作用域规则
- C语言 - 作用域规则
- C语言 - 静态变量
- C语言 - 全局变量
- C语言中的数组
- C语言 - 数组
- C语言 - 数组的特性
- C语言 - 多维数组
- C语言 - 向函数传递数组
- C语言 - 从函数返回数组
- C语言 - 变长数组
- C语言中的指针
- C语言 - 指针
- C语言 - 指针和数组
- C语言 - 指针的应用
- C语言 - 指针运算
- C语言 - 指针数组
- C语言 - 指向指针的指针
- C语言 - 向函数传递指针
- C语言 - 从函数返回指针
- C语言 - 函数指针
- C语言 - 指向数组的指针
- C语言 - 指向结构体的指针
- C语言 - 指针链
- C语言 - 指针与数组的比较
- C语言 - 字符指针和函数
- C语言 - 空指针
- C语言 - void 指针
- C语言 - 悬空指针
- C语言 - 解引用指针
- C语言 - 近指针、远指针和巨指针
- C语言 - 指针数组的初始化
- C语言 - 指针与多维数组的比较
- C语言中的字符串
- C语言 - 字符串
- C语言 - 字符串数组
- C语言 - 特殊字符
- C语言中的结构体和联合体
- C语言 - 结构体
- C语言 - 结构体和函数
- C语言 - 结构体数组
- C语言 - 自引用结构体
- C语言 - 查找表
- C语言 - 点(.)运算符
- C语言 - 枚举(enum)
- C语言 - 结构体填充和打包
- C语言 - 嵌套结构体
- C语言 - 匿名结构体和联合体
- C语言 - 联合体
- C语言 - 位域
- C语言 - typedef
- C语言中的文件处理
- C语言 - 输入输出
- C语言 - 文件I/O (文件处理)
- C语言预处理器
- C语言 - 预处理器
- C语言 - 编译指示
- C语言 - 预处理器运算符
- C语言 - 宏
- C语言 - 头文件
- C语言中的内存管理
- C语言 - 内存管理
- C语言 - 内存地址
- C语言 - 存储类别
- 其他主题
- C语言 - 错误处理
- C语言 - 变参
- C语言 - 命令执行
- C语言 - 数学函数
- C语言 - static关键字
- C语言 - 随机数生成
- C语言 - 命令行参数
- C编程资源
- C语言 - 问答
- C语言 - 快速指南
- C语言 - 速查表
- C语言 - 有用资源
- C语言 - 讨论
C语言中的static关键字
什么是C语言中的static关键字?
C语言中的**static关键字**是存储类别说明符之一,其含义取决于其使用环境。
“**static**”关键字用于声明静态变量以及定义静态函数。当声明为“static”时,变量表示静态存储类别。
**静态函数**仅在其定义所在的程序文件(扩展名为“.c”)中可用。不能使用“extern”关键字将静态函数导入到另一个文件中。
static关键字的用途
以下是static关键字的不同用途:
- **静态局部变量**: 当用static关键字声明局部变量时,其生命周期将持续到程序结束,并且它在函数调用之间保留值。
- **静态全局变量**: 当用static关键字声明全局变量时,它只能在同一个文件中访问。当您想将全局变量作为其声明文件私有的全局变量时,这很有用。
- **静态函数**: 当函数被声明为静态函数时,其作用域将限制在其声明所在的 文件中。您不能在其他文件中访问该函数。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
静态变量(使用关键字static声明变量)
**当变量声明为static时,它只初始化一次**。编译器将该变量保留到程序结束。一个静态变量也用于存储应在多个函数之间共享的数据。
以下是关于静态变量的一些重要注意事项:
- 编译器在计算机主内存中为静态变量分配空间。
- 与**auto**不同,静态变量初始化为零,而不是垃圾值。
- 如果静态变量在函数内部声明,则不会在每次函数调用时重新初始化。
- 静态变量具有局部作用域。
static关键字与变量的示例
在下面的示例中,counter()函数中的变量“x”声明为static。第一次调用counter()函数时,它被初始化为“0”。在每次后续调用中,它不会重新初始化;而是保留之前的值。
#include <stdio.h> int counter(); int main() { counter(); counter(); counter(); return 0; } int counter() { static int x; printf("Value of x as it enters the function: %d\n", x); x++; printf("Incremented value of x: %d\n", x); }
输出
运行此代码时,将产生以下输出:
Value of x as it enters the function: 0 Incremented value of x: 1 Value of x as it enters the function: 1 Incremented value of x: 2 Value of x as it enters the function: 2 Incremented value of x: 3
静态变量类似于全局变量,因为它们都初始化为0(对于数值类型)或空指针(对于指针),除非显式赋值。但是,静态变量的作用域仅限于其声明的函数或块。
静态函数(使用关键字static声明函数)
默认情况下,每个函数都被编译器视为全局函数。它们可以在程序的任何地方访问。
在定义中使用关键字“static”作为前缀时,我们将得到一个静态函数,其作用域仅限于其目标文件(保存为“.c”扩展名的程序的编译版本)。这意味着静态函数仅在其目标文件中可见。
静态函数可以通过在函数名前放置关键字“static”来声明。
static关键字与函数的示例(在多个文件中)
使用CodeBlocks IDE打开一个控制台应用程序。添加两个文件“file1.c”和“main.c”。这些文件的内容如下:
“**file1.c**”的内容:
static void staticFunc(void) { printf("Inside the static function staticFunc() "); }
“**main.c**”的内容:
#include <stdio.h> #include <stdlib.h> int main() { staticFunc(); return 0; }
现在,如果构建上述控制台应用程序项目,我们将得到一个错误,即“undefined reference to staticFunc()”。发生这种情况是因为函数staticFunc()是静态函数,它只在其目标文件中可见。
static关键字与函数的示例(在同一个文件中)
下面的程序演示了静态函数在C程序中的工作方式:
#include <stdio.h> static void staticFunc(void){ printf("Inside the static function staticFunc() "); } int main(){ staticFunc(); return 0; }
输出
上述程序的输出如下:
Inside the static function staticFunc()
在上述程序中,函数staticFunc()是一个静态函数,它打印“Inside the static function staticFunc()”。main()函数调用staticFunc()。此程序正常工作,因为静态函数仅从其自身的目标文件调用。
static关键字与多个函数的示例
您可以在同一个目标文件中包含多个静态函数,如下例所示:
#include <stdio.h> #include <stdlib.h> #include <math.h> // define the static function static int square( int num){ return num * num; } static void voidfn(){ printf ("From inside the static function.\n"); } static int intfn(int num2){ return sqrt(num2); } int main(){ int n1, val; n1 = 16; val = square(n1); // Call voidfn static function printf("The square of the %d : %d\n", n1, val); voidfn(); // Call intfn static function val = intfn(n1); printf("The square root of the %d : %d\n", n1, val); return 0; }
输出
运行此代码时,将产生以下输出:
The square of the 16: 256 From inside the static function. The square root of the 16: 4