如何在 React 组件中使用 handleChange() 函数?
handleChange() 不是 React 的内置函数,但顾名思义,我们可以定义它来处理用户对输入所做的更改。在 React 中,我们需要在用户在输入字段中输入任何值时处理输入,以使其可编辑。
在这里,我们将学习如何将 handleChange() 函数与单个和多个输入一起使用。
在函数式组件中使用 handleChange() 函数
在 React 中,我们可以使用 function 关键字定义组件并将其称为函数式组件。在使用函数式组件时,我们可以使用 Hook 来管理输入值。
语法
用户可以按照以下语法在函数式组件中使用 handleChange() 函数。
function handleChange(event) { let value = event.target.value; } <input type = "text" onChange = {handleChange} value = {value} />
在上面的语法中,我们使用了 onChange 事件属性,每当输入值发生变化时,它都会调用 handleChange() 函数。在 handleChange() 函数中,我们可以使用“event.target.value”获取新的输入值。
示例
在下面的示例中,我们创建了一个包含文本输入字段的函数式组件。我们还在函数式组件中添加了 handleChange() 函数。
在 handleChange() 函数中,我们获取更新后的输入值,并使用 Hook 将其设置为 value 变量。我们还在输出中显示“value”变量的值。
import React from "react"; import { useState } from "react"; function App() { const [value, setValue] = useState("Initial"); function handleChange(event) { // gives the value of the targetted element let value = event.target.value; setValue(value); } return ( <div> <h3> Using the <i> handleChange() function </i> with inputs in React functional components. </h3> <input type = "text" onChange = {handleChange} value = {value} /> <br/> <br/> <div style = {{ color: "blue" }}> {" "} The text entered in the input field is {value}.{" "} </div> </div> ); } export default App;
输出
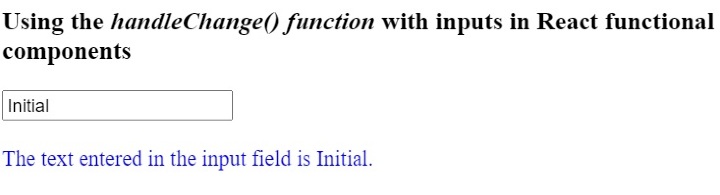
示例
在下面的示例中,我们在单个函数式组件中创建了多个输入。我们使用单个 handleChange() 函数来处理所有输入。在 handleChange() 函数中,我们可以使用“event.target.name”获取输入的名称,并根据名称使用该函数来设置输入值。
用户可以在输入字段中输入值,并观察单个 handleChange() 函数如何处理所有输入。
import React from "react"; import { useState } from "react"; function App() { const [id, setId] = useState("1"); const [firstName, setFirstName] = useState("Shubham"); const [lastName, setLastName] = useState("Vora"); const [age, setAge] = useState(22); function handleChange(event) { // gives the value of the targetted element let value = event.target.value; let inputName = event.target.name; if (inputName == "id") { setId(value); } else if (inputName == "fname") { setFirstName(value); } else if (inputName == "lname") { setLastName(value); } else { setAge(age); } } return ( <div> <h3> Using the <i> handleChange() function </i> with inputs in React functional components. </h3> <label for = "Id"> Id </label> <br /> <input type = "text" id = "Id" name = "id" onChange = {handleChange} value = {id} /> <br /> <br /> <label for = "fname"> First Name </label> <br /> <input type = "text" id = "fname" name = "fname" onChange = {handleChange} value = {firstName} /> <br /> <br /> <label for = "lname"> Last Name </label> <br /> <input type = "text" id = "lname" name = "lname" onChange = {handleChange} value = {lastName} /> <br /> <br /> <label for = "age"> Age </label> <br /> <input type = "text" id = "age" name = "age" onChange = {handleChange} value = {age} /> <br /> <br /> </div> ); } export default App;
输出

在类组件中使用 handleChange() 函数
我们可以在 ReactJS 中使用 class 关键字创建类组件。我们不能使用 Hook 来管理类组件的变量。因此,我们需要在类组件中使用 state。
此外,每当我们将 handleChange() 方法与类组件一起使用时,都需要在构造函数中绑定该方法。
语法
用户可以按照以下语法在类组件中使用 handleChange() 函数。
handleChange(event) { this.state.text = event.target.value; } <input onChange = {this.handleChange} name = "text" />
在上面的语法中,每当输入值发生变化时,我们都会更新 state 值。
示例
在下面的示例中,我们创建了一个包含输入字段的类组件。我们还在输入字段上添加了 handleChage() 方法。
每当用户更改输入值时,我们都会调用 handleChange() 方法,该方法会更新组件的状态。
import React from "react"; class App extends React.Component { constructor(props) { super(props); this.state = { text: "Sample", }; this.handleChange = this.handleChange.bind(this); } handleChange(event) { this.state.text = event.target.value; } render() { return ( <div> <h2> {" "} Using the <i> handleChange() function </i> in ReactJs with class components.{" "} </h2> <input onChange = {this.handleChange} name = "text" /> </div> ); } } export default App;
输出

用户学习了如何在 React 类组件和函数式组件中使用输入字段的 handleChange() 函数。我们还学习了如何使用单个 handleChange() 函数处理多个输入。