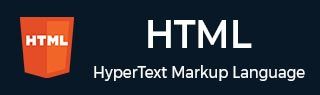
- HTML 教程
- HTML - 首页
- HTML - 路线图
- HTML - 简介
- HTML - 历史与演变
- HTML - 编辑器
- HTML - 基本标签
- HTML - 元素
- HTML - 属性
- HTML - 标题
- HTML - 段落
- HTML - 字体
- HTML - 块
- HTML - 样式表
- HTML - 格式化
- HTML - 引用
- HTML - 注释
- HTML - 颜色
- HTML - 图片
- HTML - 图片地图
- HTML - Iframes
- HTML - 短语元素
- HTML - 元标签
- HTML - 类
- HTML - ID
- HTML - 背景
- HTML 表格
- HTML - 表格
- HTML - 表格标题与说明
- HTML - 表格样式
- HTML - 表格 Colgroup
- HTML - 嵌套表格
- HTML 列表
- HTML - 列表
- HTML - 无序列表
- HTML - 有序列表
- HTML - 定义列表
- HTML 链接
- HTML - 文本链接
- HTML - 图片链接
- HTML - 邮件链接
- HTML 颜色名称与值
- HTML - 颜色名称
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML 表单
- HTML - 表单
- HTML - 表单属性
- HTML - 表单控件
- HTML - 输入属性
- HTML 媒体
- HTML - 视频元素
- HTML - 音频元素
- HTML - 嵌入多媒体
- HTML 头部
- HTML - Head 元素
- HTML - 添加 Favicon
- HTML - Javascript
- HTML 布局
- HTML - 布局
- HTML - 布局元素
- HTML - 使用 CSS 进行布局
- HTML - 响应式设计
- HTML - 符号
- HTML - 表情符号
- HTML - 样式指南
- HTML 图形
- HTML - SVG
- HTML - Canvas
- HTML API
- HTML - Geolocation API
- HTML - 拖放 API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web 存储
- HTML - 服务器发送事件
- HTML 杂项
- HTML - 文档对象模型 (DOM)
- HTML - MathML
- HTML - 微数据
- HTML - IndexedDB
- HTML - Web 消息传递
- HTML - Web CORS
- HTML - Web RTC
- HTML 演示
- HTML - 音频播放器
- HTML - 视频播放器
- HTML - 网页幻灯片
- HTML 工具
- HTML - Velocity Draw
- HTML - 二维码
- HTML - Modernizer
- HTML - 验证
- HTML - 颜色拾取器
- HTML 参考
- HTML - 速查表
- HTML - 标签参考
- HTML - 属性参考
- HTML - 事件参考
- HTML - 字体参考
- HTML - ASCII 码
- ASCII 表格查找
- HTML - 颜色名称
- HTML - 实体
- MIME 媒体类型
- HTML - URL 编码
- 语言 ISO 代码
- HTML - 字符编码
- HTML - 已弃用标签
- HTML 资源
- HTML - 快速指南
- HTML - 有用资源
- HTML - 颜色代码生成器
- HTML - 在线编辑器
HTML - DOM classList 属性
HTML DOM 的classList 属性是一个只读属性,它提供了一种访问和操作 HTML 元素类列表的方法。
此属性返回一个包含指定元素类的DOMTokenList 对象,使您可以轻松地添加、删除、切换和检查类的存在。
语法
以下是 HTML DOM 的classList 属性的语法:
element.classList;
参数
由于它是一个属性,因此不接受任何参数。
返回值
此属性返回一个 DOMTokenList 对象,该对象表示元素的 class 属性中的一组类名。
属性和方法
以下列出了您可以用来向列表中添加、切换或删除 CSS 类的属性和方法:
方法/属性 | 描述 |
---|---|
add() | 通过添加一个或多个类来修改元素的 classList。 |
remove() | 通过删除一个或多个类来修改元素的 classList。 |
toggle() | 通过切换类打开或关闭来修改元素的 classList。 |
contains() | 检查元素的 classList 是否包含特定类。 |
item() | 访问元素的 classList 中特定索引处的类。 |
replace() | 替换元素的 classList 中的一个类为另一个类。 |
entries() | 返回一个对象,该对象以键值对的形式迭代类列表。 |
keys() | 返回一个对象,该对象迭代类列表的每个索引。 |
values() | 返回一个对象,该对象允许迭代类列表的值(类名)。 |
forEach() | 对 classList 中的每个类执行给定的函数一次。 |
length | 返回元素的 classList 中的类数。 |
示例 1:向“div”元素添加新类
以下程序演示了 HTML DOM 的classList 属性的用法。它向一个<div> 元素添加一个类:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM classList</title> <style> .highlight { background-color: green; color: white; padding: 10px; } button{ padding: 8px 12px; margin: 10px 0px; } </style> </head> <body> <p>Click the below to add a class ("highlight") to the "div" element.</p> <div id="myDiv">Tutorialspoint ("TP")</div> <button onclick="addClasses()">Add Class</button> <script> function addClasses() { let divElement = document.getElementById('myDiv'); // Adding classes to the element by using add() method divElement.classList.add('highlight'); } </script> </body> </html>
上述程序向“div”元素添加了“highlight”类。
示例 2:从段落(“p”)中删除指定的类
以下是 HTML DOM 的classList 属性的另一个示例。我们使用此属性及其“remove()”方法从段落(<p>)中删除指定的类:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM classList</title> <style> .highlight { background-color: green; color: white; padding: 10px; } button{ padding: 8px 12px; } </style> </head> <body> <p>Click the below button to remove highlight from the paragraph.</p> <p id="paragraph" class="highlight">This paragraph has a highlighted background.</p> <button onclick="removeClass()">Remove Highlight Class</button> <script> function removeClass() { var paragraph = document.getElementById("paragraph"); paragraph.classList.remove("highlight"); } </script> </body> </html>
上述程序从段落中删除了指定的类。
示例 3:在突出显示段落之间切换
以下示例演示了classList 属性的“toggle()”方法的用法。此方法允许我们切换段落 (<p>) 内文本的背景颜色:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM classList</title> <style> .highlight { background-color: yellow; padding: 10px; } </style> </head> <body> <p>Click the button to add or remove the highlight...</p> <p id="paragraph">Click to toggle highlight:</p> <button onclick="document.getElementById('paragraph').classList.toggle('highlight')">Toggle Highlight </button> </body> </html>
上面的程序通过点击按钮向段落添加和删除“highlight”类。
示例 4:检查元素上的类
以下示例使用 classList 属性的“contains()”方法来检查元素是否应用了“highlight”类:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM classList</title> <style> .highlight { background-color: yellow; padding: 10px; } </style> </head> <body> <p>Click the below button to check whether the paragraph contains the "highlight" class.</p> <p id="pg" class="highlight">This paragraph has a highlighted background.</p> <button onclick="checkHighlight()">Check Highlight Class</button> <script> function checkHighlight() { var paragraph = document.getElementById("pg"); if (paragraph.classList.contains("highlight")) { alert("Yes, paragraph contains 'highlight' class"); } else { alert("No, paragraph contains 'highlight' class"); } } </script> </body> </html>
示例 5:访问特定类
此示例显示了classList 属性的“item()”方法的用法,该方法允许我们访问应用于元素的各个类名:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM classList</title> <style> .highlight { background-color: green; padding: 10px; color: white; } .italic { font-style: italic; } </style> </head> <body> <h2>Accessing Specific Classes</h2> <p id="paragraph" class="highlight italic">This paragraph has multiple classes.</p> <button onclick="getClassNames()">Get Classes</button> <p id="classOutput"></p> <script> function getClassNames() { var paragraph = document.getElementById("paragraph"); var classList = paragraph.classList; // Accessing specific classes by index var firstClass = classList.item(0); var secondClass = classList.item(1); var thirdClass = classList.item(2); // Displaying the classes document.getElementById("classOutput").textContent="First class: " + firstClass + ", Second class: " + secondClass; } </script> </body> </html>
示例 6:动态替换类
此示例显示了如何使用classList 属性的“replace()”方法动态地从元素中替换类:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM classList</title> <style> .highlight { background-color: yellow; padding: 10px; } .italic { background-color: green; padding: 10px; color: white; } button{ padding: 8px 10px; } </style> </head> <body> <p id="paragraph" class="highlight">This paragraph has a highlighted background.</p> <button onclick="replaceClass()">Replace Highlight with Italic</button> <script> function replaceClass() { var paragraph = document.getElementById("paragraph"); paragraph.classList.replace("highlight","italic"); } </script> </body> </html>
示例 7:遍历类
此示例演示了如何使用classList 属性的“forEach()”方法遍历动态应用于元素的每个类:
<!DOCTYPE html> <html> <head> <title>HTML DOM classList</title> <style> .red { color: red; padding: 10px; } .bold { font-weight: bold; } .underline { text-decoration: underline; } </style> </head> <body> <p>It will iterate over the class values..</p> <div id="ex" class="red bold underline">Dynamic Classes</div> <button onclick="applyForEach()">Apply forEach</button> <div id="output"></div> <script> function applyForEach() { const element = document.getElementById('ex'); let res="<p><strong>ClassList Classes:</strong></p>"; element.classList.forEach(className => { res += `<p>${className}</p>`; }); document.getElementById('output').innerHTML=res; } </script> </body> </html>
示例 8:检索类数
此示例显示了如何使用 HTML DOM 的classList.length 属性检索应用于元素(即<div>)的类数:
<!DOCTYPE html> <html> <head> <title>HTML DOM classList</title> <style> .red { color: red; padding: 10px; } .b { font-weight: bold; } .udl { text-decoration: underline; } button{ padding: 8px 12px; } </style> </head> <body> <p>Click the below button to displays number of classes applied...</p> <div id="ex" class="red b udl">Dynamic Classes</div> <button onclick="displayClassListLength()">Display ClassList Length</button> <div id="output"></div> <script> function displayClassListLength() { const element = document.getElementById('ex'); const classLength = element.classList.length; const res = `<p>Number of Classes: ${classLength}</p>`; document.getElementById('output').innerHTML = res; } </script> </body> </html>
支持的浏览器
属性 | ![]() |
![]() |
![]() |
![]() |
![]() |
---|---|---|---|---|---|
classList | 是 | 是 | 是 | 是 | 是 |