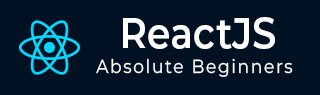
- ReactJS 教程
- ReactJS - 首页
- ReactJS - 简介
- ReactJS - 路线图
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优点与缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用新创建的组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性 (props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建一个事件感知组件
- ReactJS - 在 Expense Manager 应用中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React Hooks 的组件生命周期
- ReactJS - 布局组件
- ReactJS - 分页
- ReactJS - Material UI
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 非受控组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - Keys
- ReactJS - 路由
- ReactJS - Redux
- ReactJS - 动画
- ReactJS - Bootstrap
- ReactJS - 地图
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- Hooks
- ReactJS - Hooks 简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义 Hooks
- ReactJS 高级
- ReactJS - 可访问性
- ReactJS - 代码分割
- ReactJS - Context
- ReactJS - 错误边界
- ReactJS - 转发 Refs
- ReactJS - 碎片
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 优化性能
- ReactJS - Profiler API
- ReactJS - 端口
- ReactJS - 无 ES6 ECMAScript 的 React
- ReactJS - 无 JSX 的 React
- ReactJS - 调和
- ReactJS - Refs 和 DOM
- ReactJS - 渲染 Props
- ReactJS - 静态类型检查
- ReactJS - 严格模式
- ReactJS - Web Components
- 其他概念
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - 轮播图
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
- ReactJS 有用资源
- ReactJS - 快速指南
- ReactJS - 有用资源
- ReactJS - 讨论
ReactJS - componentDidCatch() 方法
React 是一个流行的 JavaScript 库,用于创建用户界面。在创建 React 应用时,我们可能会遇到代码错误。当这些错误发生在 React 组件中时,正确处理它们可能是一项艰巨的任务。这就是 componentDidCatch 函数可以发挥作用的地方。因此,componentDidCatch 捕获我们组件中的错误并防止我们的整个应用崩溃。所以我们将通过一个例子来了解它是如何工作的。
什么是 componentDidCatch?
componentDidCatch 是一个可以在 React 组件中定义的函数。其主要功能是检测任何组件子元素中的错误,无论该子元素在组件树中位于何处。
当任何子组件中发生错误时,React 将调用 componentDidCatch。它给了我们一个响应错误的机会。
componentDidCatch 的一个常见用法是记录错误。在生产环境中,这可以发送到错误报告服务,使我们能够跟踪和修复问题。
语法
componentDidCatch(error, info)
参数
componentDidCatch 函数使用两个参数:error 和 info。
error - 这是抛出的错误。它通常是 Error 对象的一个实例,但也可能是字符串或 null。
info - 这是有关错误的数据。它提供了一个堆栈跟踪,指示问题发生的位置以及涉及哪些组件。
返回值
componentDidCatch 函数不返回任何内容。它主要用于指示错误不产生结果。
如何使用它?
我们将创建一个应用并使用 componentDidCatch 来展示其用法 -
在这个应用中,我们有一个 MyComponent,它在其 componentDidMount 函数中故意抛出一个错误以获取错误。我们将在 ErrorBoundary 中使用 MyComponent,它将捕获并处理 MyComponent 中发生的任何错误。如果 MyComponent 中发生错误,ErrorBoundary 将显示一个自定义的回退 UI,其中包含错误消息。
示例
示例
import React from 'react'; class MyComponent extends React.Component { constructor(props) { super(props); this.state = { data: null }; } componentDidMount() { // Try to parse invalid JSON to evoke an error. try { JSON.parse("This is not valid JSON"); } catch (error) { throw new Error("Something went wrong in MyComponent!"); } } render() { // Render the component as usual return <div>{this.state.data}</div>; } } class ErrorBoundary extends React.Component { constructor(props) { super(props); this.state = { hasError: false, error: null }; } static getDerivedStateFromError(error) { // Update state to show a fallback UI and store the error return { hasError: true, error }; } componentDidCatch(error, info) { console.error("Error caught by ErrorBoundary:", error); console.error("Component Stack:", info.componentStack); } render() { if (this.state.hasError) { // Render a custom fallback UI with the error message return ( <div> <h2>Something went wrong</h2> <p>{this.state.error.message}</p> </div> ); } // Render the child components if no error found return this.props.children; } } function App() { return ( <div> <h1>My App</h1> <ErrorBoundary> <MyComponent /> </ErrorBoundary> </div> ); } export default App;
输出
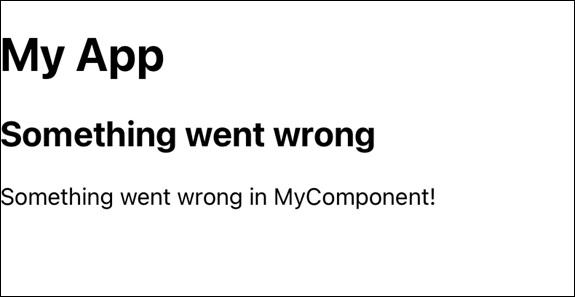
示例 - 带有错误处理的动态表单
此应用显示了一个动态表单,用户可以在其中将数据输入字段。如果在表单数据处理期间发生错误,componentDidCatch 方法将记录错误并告知用户该问题。
import React, { Component } from 'react'; class DynamicForm extends Component { constructor(props) { super(props); this.state = { formData: {}, hasError: false, }; } handleInputChange = (field, value) => { try { // Logic to handle dynamic form data this.setState(prevState => ({ formData: { ...prevState.formData, [field]: value, }, })); } catch (error) { // Handle errors this.setState({ hasError: true }); } }; handleButtonClick = () => { // Sending form data to a server try { // Logic to send data to the server console.log('Sending form data:', this.state.formData); } catch (error) { // Handle errors in sending data console.error('Error sending form data:', error); this.setState({ hasError: true }); } }; componentDidCatch(error, info) { console.error('Form Error:', error, info); this.setState({ hasError: true }); } render() { if (this.state.hasError) { return <div>Sorry, there was an issue with the form.</div>; } return ( <div> <h1>Dynamic Form</h1> <input type="text" placeholder="Field 1" onChange={e => this.handleInputChange('field1', e.target.value)} /> <input type="text" placeholder="Field 2" onChange={e => this.handleInputChange('field2', e.target.value)} /> <button onClick={this.handleButtonClick}>Send Data</button> </div> ); } } export default DynamicForm;
输出
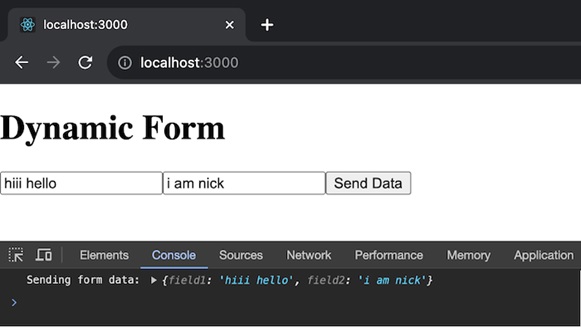
当用户点击“发送数据”按钮时,表单数据将记录到控制台。我们可以用我们发送数据到服务器的实际逻辑替换 handleButtonClick 方法中的 console.log 语句,使用 fetch。
注释
过去,开发人员在 componentDidCatch 内部使用 setState 来更改用户界面并显示错误警告。这种方法现在被认为已过时。为了处理错误,最好使用 static getDerivedStateFromError。
在开发和生产环境中,React 的行为有所不同。在开发环境中,componentDidCatch 将捕获错误并将它们发送到浏览器的全局错误处理程序。它们不会在生产环境中冒泡,因此我们必须使用 componentDidCatch 显式捕获它们。
总结
componentDidCatch 是一个有用的 React 功能,它使我们能够正确地管理组件中的错误。它就像一个安全网,记录错误并允许我们记录它们或显示错误消息。请记住,它应该与 getDerivedStateFromError 结合使用,并且开发和生产设置之间存在差异。