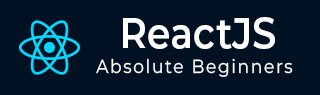
- ReactJS 教程
- ReactJS - 首页
- ReactJS - 简介
- ReactJS - 路线图
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优点与缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用新创建的组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性 (props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建一个事件感知组件
- ReactJS - 在 Expense Manager 应用中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React Hooks 进行组件生命周期管理
- ReactJS - 布局组件
- ReactJS - 分页
- ReactJS - Material UI
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 非受控组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - 键
- ReactJS - 路由
- ReactJS - Redux
- ReactJS - 动画
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- Hooks
- ReactJS - Hooks 简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义 Hooks
- ReactJS 高级
- ReactJS - 可访问性
- ReactJS - 代码分割
- ReactJS - Context
- ReactJS - 错误边界
- ReactJS - 转发 Refs
- ReactJS - 片段
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 优化性能
- ReactJS - Profiler API
- ReactJS - 传送门
- ReactJS - 无 ES6 ECMAScript 的 React
- ReactJS - 无 JSX 的 React
- ReactJS - 调和
- ReactJS - Refs 和 DOM
- ReactJS - 渲染 Props
- ReactJS - 静态类型检查
- ReactJS - 严格模式
- ReactJS - Web Components
- 附加概念
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - 走马灯
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
- ReactJS 有用资源
- ReactJS - 快速指南
- ReactJS - 有用资源
- ReactJS - 讨论
ReactJS - 键
列表和键
在之前的章节中,我们学习了如何使用 for 循环和 map 函数在 React 中使用集合。如果我们运行应用程序,它将按预期输出。如果我们在浏览器中打开开发者控制台,则会显示如下警告:
Warning: Each child in a list should have a unique "key" prop. Check the render method of `ExpenseListUsingForLoop`. See https://reactjs.ac.cn/link/warning-keys for more information. tr ExpenseListUsingForLoop@ div App
那么,这是什么意思,它如何影响我们的 React 应用程序?众所周知,React 尝试通过各种机制仅渲染 DOM 中已更新的值。当 React 渲染集合时,它会尝试通过仅更新列表中已更新的项来优化渲染。
但是,React 没有提示来查找哪些项是新的、已更新的或已删除的。为了获取信息,React 允许为所有组件设置 key 属性。唯一的要求是 key 的值在当前集合中必须唯一。
让我们重新创建我们之前的应用程序之一并应用 key 属性。
create-react-app myapp cd myapp npm start
接下来,在 components 文件夹下创建一个组件,**ExpenseListUsingForLoop** (src/components/ExpenseListUsingForLoop.js)。
import React from 'react' class ExpenseListUsingForLoop extends React.Component { render() { return <table> <thead> <tr> <th>Item</th> <th>Amount</th> </tr> </thead> <tbody> </tbody> <tfoot> <tr> <th>Sum</th> <th></th> </tr> </tfoot> </table> } } export default ExpenseListUsingForLoop
在这里,我们创建了一个带有表头和表尾的基本表格结构。然后,创建一个函数来查找总支出金额。我们稍后会在 render 方法中使用它。
getTotalExpenses() { var items = this.props['expenses']; var total = 0; for(let i = 0; i < items.length; i++) { total += parseInt(items[i]); } return total; }
在这里,getTotalExpenses 遍历 expense props 并汇总总支出。然后,在 render 方法中添加支出项和总金额。
render() { var items = this.props['expenses']; var expenses = [] expenses = items.map((item, idx) => <tr key={idx}><td>item {idx + 1}</td><td>{item}</td></tr>) var total = this.getTotalExpenses(); return <table> <thead> <tr> <th>Item</th> <th>Amount</th> </tr> </thead> <tbody> {expenses} </tbody> <tfoot> <tr> <th>Sum</th> <th>{total}</th> </tr> </tfoot> </table> }
这里:
使用 map 函数遍历 expense 数组中的每个项目,使用转换函数为每个条目创建表格行 (tr),最后将返回的数组设置到 expenses 变量中。
为每一行设置 key 属性,其值为项目的索引值。
在 JSX 表达式中使用 expenses 数组来包含生成的 rows。
使用 getTotalExpenses 方法查找总支出金额并将其添加到 render 方法中。
ExpenseListUsingForLoop 组件的完整源代码如下:
import React from 'react' class ExpenseListUsingForLoop extends React.Component { getTotalExpenses() { var items = this.props['expenses']; var total = 0; for(let i = 0; i < items.length; i++) { total += parseInt(items[i]); } return total; } render() { var items = this.props['expenses']; var expenses = [] expenses = items.map( (item, idx) => <tr key={idx}><td>item {idx + 1}</td><td>{item}</td></tr>) var total = this.getTotalExpenses(); return <table> <thead> <tr> <th>Item</th> <th>Amount</th> </tr> </thead> <tbody> {expenses} </tbody> <tfoot> <tr> <th>Sum</th> <th>{total}</th> </tr> </tfoot> </table> } } export default ExpenseListUsingForLoop
接下来,使用 ExpenseListUsingForLoop 组件更新 App 组件 (App.js)。
import ExpenseListUsingForLoop from './components/ExpenseListUsingForLoop'; import './App.css'; function App() { var expenses = [100, 200, 300] return ( <div> <ExpenseListUsingForLoop expenses={expenses} /> </div> ); } export default App;
接下来,在App.css中添加基本的样式。
/* Center tables for demo */ table { margin: 0 auto; } div { padding: 5px; } /* Default Table Style */ table { color: #333; background: white; border: 1px solid grey; font-size: 12pt; border-collapse: collapse; } table thead th, table tfoot th { color: #777; background: rgba(0,0,0,.1); text-align: left; } table caption { padding:.5em; } table th, table td { padding: .5em; border: 1px solid lightgrey; }
接下来,在浏览器中检查应用程序。它将显示如下支出:
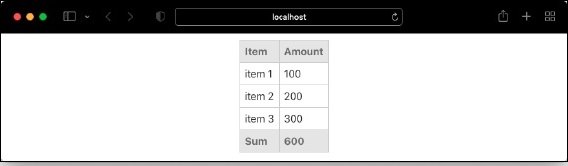
最后,打开开发者控制台,发现没有显示关于 key 的警告。
键和索引
我们了解到 key 应该唯一以优化组件的渲染。我们使用了索引值,错误消失了。这仍然是为列表提供值的正确方法吗?答案是肯定的和否定的。设置索引 key 在大多数情况下都能工作,但如果我们在应用程序中使用非受控组件,它会以意想不到的方式运行。
让我们更新我们的应用程序并添加两个如下所述的新功能:
在支出金额旁边添加一个输入元素到每一行。
添加一个按钮以删除列表中的第一个元素。
首先,添加一个构造函数并设置应用程序的初始状态。因为我们将在应用程序的运行时删除某些项目,所以我们应该使用状态而不是 props。
constructor(props) { super(props) this.state = { expenses: this.props['expenses'] } }
接下来,添加一个函数来删除列表的第一个元素。
remove() { var itemToRemove = this.state['expenses'][0] this.setState((previousState) => ({ expenses: previousState['expenses'].filter((item) => item != itemToRemove) })) }
接下来,在构造函数中绑定 remove 函数,如下所示:
constructor(props) { super(props) this.state = { expenses: this.props['expenses'] } this.remove = this.remove.bind(this) }
接下来,在表格下方添加一个按钮,并在其 onClick 操作中设置 remove 函数。
render() { var items = this.state['expenses']; var expenses = [] expenses = items.map( (item, idx) => <tr key={idx}><td>item {idx + 1}</td><td>{item}</td></tr>) var total = this.getTotalExpenses(); return ( <div> <table> <thead> <tr> <th>Item</th> <th>Amount</th> </tr> </thead> <tbody> {expenses} </tbody> <tfoot> <tr> <th>Sum</th> <th>{total}</th> </tr> </tfoot> </table> <div> <button onClick={this.remove}>Remove first item</button> </div> </div> ) }
接下来,在所有行中支出金额旁边添加一个输入元素,如下所示:
expenses = items.map((item, idx) => <tr key={idx}><td>item {idx + 1}</td><td>{item} <input /></td></tr>)
接下来,在浏览器中打开应用程序。应用程序将如下渲染:
接下来,在第一个输入框中输入一个金额(例如,100),然后单击“删除第一个项目”按钮。这将删除第一个元素,但输入的金额将填充到第二个元素(金额:200)旁边的输入框中,如下所示:
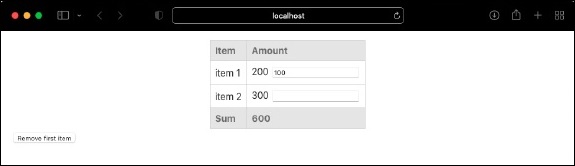
为了解决这个问题,我们应该删除索引作为 key 的用法。相反,我们可以使用表示项目的唯一 ID。让我们将项目从数字数组更改为对象数组,如下所示 (App.js):
import ExpenseListUsingForLoop from './components/ExpenseListUsingForLoop'; import './App.css'; function App() { var expenses = [ { id: 1, amount: 100 }, { id: 2, amount: 200 }, { id: 3, amount: 300 } ] return ( <div> <ExpenseListUsingForLoop expenses={expenses} /> </div> ); } export default App;
接下来,通过将 item.id 设置为 key 来更新渲染逻辑,如下所示:
expenses = items.map((item, idx) => <tr key={item.id}><td>{item.id}</td><td>{item.amount} <input /></td></tr>)
接下来,更新 getTotalExpenses 逻辑,如下所示:
getTotalExpenses() { var items = this.props['expenses']; var total = 0; for(let i = 0; i < items.length; i++) { total += parseInt(items[i].amount); } return total; }
在这里,我们更改了从对象获取金额的逻辑 (item[i].amount)。最后,在浏览器中打开应用程序并尝试重现之前的错误。在第一个输入框中添加一个数字(例如 100),然后单击“删除第一个项目”。现在,第一个元素被删除,并且输入的值不会保留在下一个输入框中,如下所示:
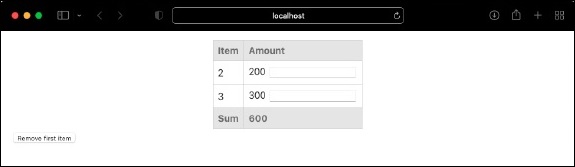