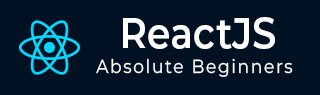
- ReactJS 教程
- ReactJS - 首页
- ReactJS - 简介
- ReactJS - 路线图
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优点与缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用新创建的组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性 (props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建一个事件感知组件
- ReactJS - 在 Expense Manager 应用中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React Hooks 进行组件生命周期管理
- ReactJS - 布局组件
- ReactJS - 分页
- ReactJS - Material UI
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 非受控组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - Keys
- ReactJS - 路由
- ReactJS - Redux
- ReactJS - 动画
- ReactJS - Bootstrap
- ReactJS - 地图
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- Hooks
- ReactJS - Hooks 简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义 Hooks
- ReactJS 高级
- ReactJS - 可访问性
- ReactJS - 代码分割
- ReactJS - 上下文
- ReactJS - 错误边界
- ReactJS - 转发 Refs
- ReactJS - 片段
- ReactJS - 高阶组件
- ReactJS - 集成其他库
- ReactJS - 优化性能
- ReactJS - Profiler API
- ReactJS - 门户
- ReactJS - 无 ES6/ECMAScript 的 React
- ReactJS - 无 JSX 的 React
- ReactJS - 调和
- ReactJS - Refs 和 DOM
- ReactJS - 渲染 Props
- ReactJS - 静态类型检查
- ReactJS - 严格模式
- ReactJS - Web Components
- 其他概念
- ReactJS - 日期选择器
- ReactJS - 头盔组件(Helmet)
- ReactJS - 内联样式
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - 走马灯
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
- ReactJS 有用资源
- ReactJS - 快速指南
- ReactJS - 有用资源
- ReactJS - 讨论
ReactJS - 头盔组件(Helmet)
Web 文档的元信息对于 SEO 非常重要。文档的元信息通常在 head 部分使用 meta 标签指定。title 标签在提供文档的元信息方面也发挥着重要作用。Head 部分还将包含 script 和 style 标签。Helmet 组件通过提供所有有效的 head 标签,提供了一种简单的方法来管理文档的 head 部分。Helmet 将收集其内部指定的所有信息,并更新文档的 head 部分。
让我们在本节学习如何在 React 中使用 Helmet 组件。
安装 Helmet
在学习 Helmet 的概念和用法之前,让我们先学习如何使用 npm 命令安装 Helmet 组件。
npm install --save react-helmet
上述命令将安装 Helmet 组件,并使其在我们的应用程序中可以使用。
Helmet 的概念和用法
Helmet 接受所有有效的 head 标签。它接受普通的 HTML 标签,并将标签输出到文档的 head 部分,如下所示:
import React from "react"; import {Helmet} from "react-helmet"; class Application extends React.Component { render () { return ( <div className="application"> <Helmet> <title>Helmet sample application</title> <meta charSet="utf-8" /> <meta name="description" content="Helmet sample program to understand the working of the helmet component" /> <meta name="keywords" content="React, Helmet, HTML, CSS, Javascript" /> <meta name="author" content="Peter" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> </Helmet> // ... </div> ); } };
这里:
title 用于指定文档的标题
description meta 标签用于指定文档的详细信息
keywords 用于指定文档的主要关键词。搜索引擎将使用它。
author 用于指定文档的作者
viewport 用于指定文档的默认视口
charSet 用于指定文档中使用的字符集。
head 部分的输出如下:
<head> <title>Helmet sample application</title> <meta charSet="utf-8" /> <meta name="description" content="Helmet sample program to understand the working of the helmet component" /> <meta name="keywords" content="React, Helmet, HTML, CSS, Javascript" /> <meta name="author" content="Peter" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> </head>
Helmet 组件可以在任何其他 React 组件内部使用以更改 header 部分。它也可以嵌套,以便在渲染子组件时更改 header 部分。
<Parent> <Helmet> <title>Helmet sample application</title> <meta charSet="utf-8" /> <meta name="description" content="Helmet sample program to understand the working of the helmet component" /> <meta name="keywords" content="React, Helmet, HTML, CSS, Javascript" /> <meta name="author" content="Peter" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> </Helmet> <Child> <Helmet> <title>Helmet sample application :: rendered by child component</title> <meta name="description" content="Helmet sample program to explain nested feature of the helmet component" /> </Helmet> </Child> </Parent>
这里:
子组件中的 Helmet 将更新 head 部分,如下所示:
<head> <title>Helmet sample application :: rendered by child component</title> <meta charSet="utf-8" /> <meta name="description" content="Helmet sample program to explain nested feature of the helmet component" /> <meta name="keywords" content="React, Helmet, HTML, CSS, Javascript"> <meta name="author" content="Peter"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> </head>
应用 Helmet
让我们在本节创建一个新的 React 应用程序,学习如何在其中应用 Helmet 组件。
首先,使用以下命令创建一个新的 React 应用程序并启动它。
create-react-app myapp cd myapp npm start
接下来,打开 App.css (src/App.css) 并移除所有 CSS 类。
// remove all css classes
接下来,创建一个简单的组件,SimpleHelmet (src/Components/SimpleHelmet.js) 并渲染:
import React from "react"; import {Helmet} from "react-helmet"; class SimpleHelmet extends React.Component { render() { return ( <div> <Helmet> <title>Helmet sample application</title> <meta charSet="utf-8" /> <meta name="description" content="Helmet sample program to understand the working of the helmet component" /> <meta name="keywords" content="React, Helmet, HTML, CSS, Javascript" /> <meta name="author" content="Peter" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> </Helmet> <p>A sample application to demonstrate helmet component.</p> </div> ) } } export default SimpleHelmet;
这里我们有:
从 react-helmet 包中导入了 Helmet
在组件中使用 Helmet 更新 head 部分。
接下来,打开 App 组件 (src/App.js),并使用 SimpleHelmet 组件,如下所示:
import './App.css' import React from 'react'; import SimpleHelmet from './Components/SimpleHelmet' function App() { return ( <div className="container"> <div style={{ padding: "10px" }}> <div> <SimpleHelmet /> </div> </div> </div> ); } export default App;
这里我们有:
从 react 包中导入了 SimpleHelmet 组件
使用了 SimpleHelmet 组件
接下来,打开 index.html (public/index.html) 文件并移除 meta 标签,如下所示:
<!DOCTYPE html> <html lang="en"> <head> <link rel="icon" href="%PUBLIC_URL%/favicon.ico" /> <link rel="apple-touch-icon" href="%PUBLIC_URL%/logo192.png" /> <link rel="manifest" href="%PUBLIC_URL%/manifest.json" /> </head> <body> <noscript>You need to enable JavaScript to run this app.</noscript> <div style="padding: 10px;"> <div id="root"></div> </div> </body> </html>
这里:
title 标签被移除
meta 标签(描述、主题颜色和视口)被移除
最后,在浏览器中打开应用程序并检查最终结果。
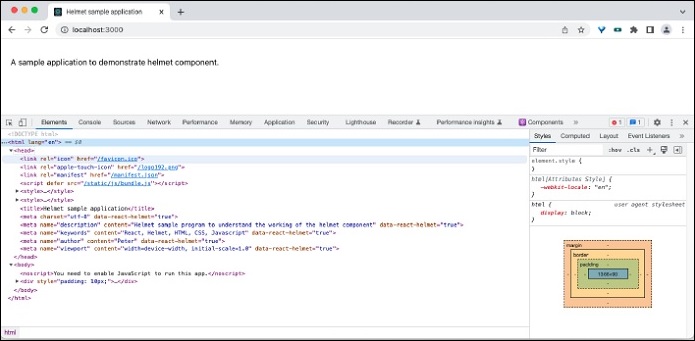
在开发者工具中打开源代码,您可以看到如下所示的 html 信息:
<title>Helmet sample application</title> <meta charset="utf-8" data-react-helmet="true"> <meta name="description" content="Helmet sample program to understand the working of the helmet component" data-react-helmet="true"> <meta name="keywords" content="React, Helmet, HTML, CSS, Javascript" data-react-helmet="true"> <meta name="author" content="Peter" data-react-helmet="true"> <meta name="viewport" content="width=device-width, initial-scale=1.0" data-react-helmet="true">
总结
Helmet 组件是一个易于使用的组件,用于管理文档的 head 内容,支持服务器端和客户端渲染。