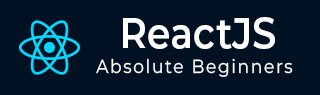
- ReactJS 教程
- ReactJS - 首页
- ReactJS - 简介
- ReactJS - 路线图
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用新创建的组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性 (props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建一个事件感知组件
- ReactJS - 在 Expense Manager 应用中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React Hooks 进行组件生命周期管理
- ReactJS - 布局组件
- ReactJS - 分页
- ReactJS - Material UI
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 非受控组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - Keys
- ReactJS - 路由
- ReactJS - Redux
- ReactJS - 动画
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- Hooks
- ReactJS - Hooks 简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义 Hooks
- ReactJS 高级
- ReactJS - 可访问性
- ReactJS - 代码分割
- ReactJS - Context
- ReactJS - 错误边界
- ReactJS - 转发 Refs
- ReactJS - 片段
- ReactJS - 高阶组件
- ReactJS - 集成其他库
- ReactJS - 优化性能
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - 无 ES6 ECMAScript 的 React
- ReactJS - 无 JSX 的 React
- ReactJS - 调和
- ReactJS - Refs 和 DOM
- ReactJS - 渲染 Props
- ReactJS - 静态类型检查
- ReactJS - 严格模式
- ReactJS - Web Components
- 其他概念
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - 轮播图
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
- ReactJS 有用资源
- ReactJS - 快速指南
- ReactJS - 有用资源
- ReactJS - 讨论
ReactJS - componentWillUnmount() 方法
组件是我们 React 应用的构建块。它们类似于乐高积木,我们将其组装成更大的建筑物。componentWillUnmount() 是每个组件都可用的生命周期方法之一。
componentWillUnmount 方法是 React 类组件的一部分。React 在组件从屏幕上移除或“卸载”之前调用它。这是一个用于清理任务的常用位置,例如取消数据获取或停用订阅。
语法
componentWillUnmount() { // Cleanup and resource release logic here }
参数
componentWillUnmount 不接受任何参数。它是一个生命周期函数,当组件即将被卸载时,React 会调用它。
返回值
componentWillUnmount 函数不应返回任何内容。它用于执行清理操作并释放资源,而不是返回值。
示例
示例
让我们创建一个基本的示例应用来展示如何在 React 类组件中使用 componentWillUnmount 函数。在这个示例中,我们将创建一个计数器应用,它在组件挂载时启动计时器,并在组件卸载时停止计时器。
import React, { Component } from 'react'; class App extends Component { state = { count: 0, }; timerID = null; componentDidMount() { // Start the timer when the component mounts this.timerID = setInterval(() => { this.setState((prevState) => ({ count: prevState.count + 1 })); }, 1000); } componentWillUnmount() { // Stop the timer when the component unmounts clearInterval(this.timerID); } render() { return ( <div> <h1>Counter: {this.state.count}</h1> </div> ); } } export default App;
输出
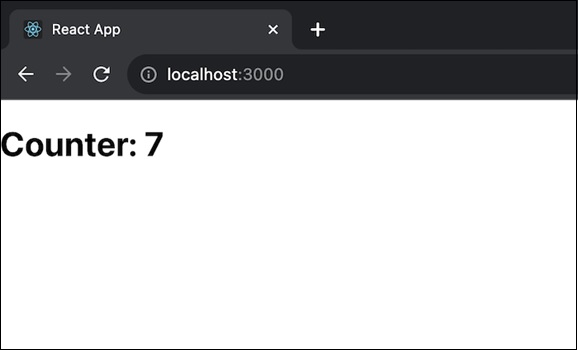
我们创建了一个 CounterApp 组件,它扩展了 Component。在 componentDidMount 方法中,我们使用 setInterval 启动了一个计时器,每秒更新一次 count 状态。这是一个简单的计数器,每秒递增一次。在 componentWillUnmount 方法中,我们使用 clearInterval 停止了计时器,以防止组件卸载时出现内存泄漏。render 方法显示当前的计数器值。
此应用展示了如何使用 componentWillUnmount 函数来清理资源。在我们的例子中,它是在组件卸载时停止计时器。
示例 - 用户资料应用
在此应用中,我们将有一个用户资料显示,并且 componentWillUnmount() 函数用于在组件卸载时可能需要的任何清理操作。
UserProfileApp.js
import React, { Component } from 'react'; import './App.css'; class UserProfileApp extends Component { state = { userProfile: { username: 'Akshay_Sharma', email: '[email protected]', }, }; componentDidMount() { // Fetch user profile data when the component mounts } componentWillUnmount() { // Any cleanup needed for user profile app can be done here console.log('UserProfileApp will unmount'); } render() { const { username, email } = this.state.userProfile; return ( <div className='App user-profile-container'> <h1>User Profile</h1> <p>Username: {username}</p> <p>Email: {email}</p> </div> ); } } export default UserProfileApp;
App.css
.user-profile-container { max-width: 400px; margin: auto; padding: 20px; border: 1px solid #ccc; border-radius: 8px; box-shadow: 0 2px 5px rgba(0, 0, 0, 0.1); background-color:rgb(224, 204, 178); } h1 { color: red; } p { margin: 8px 0; color: green; }
输出
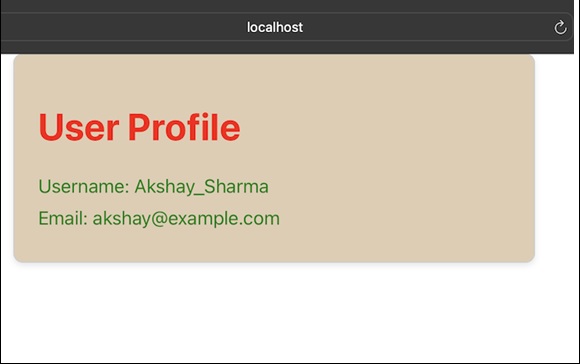
示例 - 倒计时应用
在此应用中,我们将有一个倒计时器,它在组件挂载时启动,并在组件即将卸载时停止。所以此应用的代码如下:
CountdownTimerApp.js
import React, { Component } from 'react'; import './App.css' class CountdownTimerApp extends Component { state = { time: 10, }; timerID = null; componentDidMount() { // Start the countdown timer when the component mounts this.timerID = setInterval(() => { this.setState((prevState) => ({ time: prevState.time - 1 })); }, 1000); } componentWillUnmount() { // Stop the countdown timer when the component unmounts clearInterval(this.timerID); } render() { return ( <div className='App'> <h1>Countdown Timer: {this.state.time}s</h1> </div> ); } } export default CountdownTimerApp;
输出
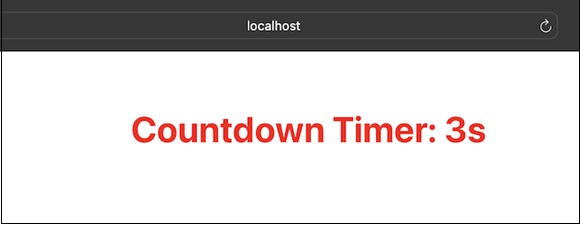
componentWillUnmount() 方法用于在组件即将卸载时清除间隔或执行清理操作。
局限性
在 React 的严格模式下进行开发时,React 可以使用 componentDidMount,立即调用 componentWillUnmount,然后再次调用 componentDidMount。这是一个用于识别 componentWillUnmount 问题并确保其正确复制 componentDidMount 行为的有用工具。
总结
componentWillUnmount 是 React 类组件中的一个方法,用于在从屏幕上清除组件之前清理资源。对于停止数据获取和停用订阅等操作,它是必需的。我们通过复制 componentDidMount 中的行为来确保我们的组件在其整个生命周期中都能顺利运行。