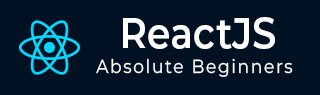
- ReactJS 教程
- ReactJS - 首页
- ReactJS - 简介
- ReactJS - 路线图
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优势与劣势
- ReactJS - 架构
- ReactJS - 创建 React 应用
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用新创建的组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性 (props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建一个事件感知组件
- ReactJS - 在 Expense Manager 应用中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React Hooks 进行组件生命周期管理
- ReactJS - 布局组件
- ReactJS - 分页
- ReactJS - Material UI
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 非受控组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - Keys
- ReactJS - 路由
- ReactJS - Redux
- ReactJS - 动画
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- Hooks
- ReactJS - Hooks 简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义 Hooks
- ReactJS 高级
- ReactJS - 可访问性
- ReactJS - 代码分割
- ReactJS - Context
- ReactJS - 错误边界
- ReactJS - 转发 Refs
- ReactJS - Fragments
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 性能优化
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - 无 ES6 ECMAScript 的 React
- ReactJS - 无 JSX 的 React
- ReactJS - 调和
- ReactJS - Refs 和 DOM
- ReactJS - Render Props
- ReactJS - 静态类型检查
- ReactJS - Strict Mode
- ReactJS - Web Components
- 其他概念
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - 轮播图
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
- ReactJS 有用资源
- ReactJS - 快速指南
- ReactJS - 有用资源
- ReactJS - 讨论
ReactJS - 事件对象
在 React 中,当我们为按钮点击等事件构建事件处理程序时,我们会得到一个称为 React 事件对象(或合成事件)的特殊对象。此对象充当我们的代码和浏览器事件之间的桥梁。它减少了 Web 浏览器之间的差异,并提供了一些重要信息。
如何使用它?
让我们使用一个简单的示例来分解它 -
假设我们在我们的 React 应用中有一个按钮,我们希望在单击它时执行某些操作。因此,我们将定义一个 onClick 处理程序函数,如下所示 -
示例
<button onClick={e => { console.log(e); // The event object }} />
在此代码中,“e”是我们的 React 事件对象,它提供有关按钮点击的信息。
发现事件对象可以做什么!
让我们浏览一下 React 事件对象实现的一些标准事件属性 -
序号 | 属性和描述 |
---|---|
1 | e.bubbles 这告诉我们事件是否在网页上的元素之间“冒泡”。例如,如果我们有一个在 div 内的按钮,则点击事件可能会从按钮冒泡到 div。它是一个布尔值(true 或 false)。 |
2 | e.cancelable 它表明我们可以停止事件的默认行为。例如,我们可以阻止在单击按钮时提交表单。它也是布尔类型。 |
3 | e.currentTarget 它指向在我们的 React 组件中附加事件处理程序的元素。在本例中,它是对按钮的引用。 |
4 | e.defaultPrevented 告诉我们是否有人使用 preventDefault() 来停止事件的默认操作。一个布尔值。 |
5 | e.eventPhase 它是一个数字,告诉我们事件处于哪个阶段(如捕获、目标或冒泡)。 |
6 | e.isTrusted 显示事件是否由用户启动。如果为 true,则为真正的用户操作;如果为 false,则可能是由代码触发的。 |
7 | e.target 这指向实际发生事件的元素。它可能是按钮。 |
8 | e.timeStamp 它提供事件发生的时间。 |
9 | e.nativeEvent 我们可以使用它来访问底层的浏览器事件。 |
示例 - 使用事件对象
让我们创建一个简单的应用程序来显示事件对象属性的工作原理。因此,在这个应用程序中,我们创建一个名为 EventHandlingExample.js 的组件,然后我们将在 App.js 组件中使用此组件来查看其工作原理。
EventHandlingExample.js
import React from 'react'; class EventHandlingExample extends React.Component { handleClick = (e) => { // Logging event properties console.log('Bubbles:', e.bubbles); console.log('Cancelable:', e.cancelable); console.log('Current Target:', e.currentTarget); console.log('Default Prevented:', e.defaultPrevented); console.log('Event Phase:', e.eventPhase); console.log('Is Trusted:', e.isTrusted); console.log('Target:', e.target); console.log('Time Stamp:', e.timeStamp); // Prevent the default behavior e.preventDefault(); } render() { return ( <div> <button onClick={this.handleClick}>Click Me</button> </div> ); } } export default EventHandlingExample;
App.js
import React from 'react'; import './App.css'; import EventHandlingExample from './EventHandlingExample'; function App() { return ( <div className="App"> <h1>React Event Object Example</h1> <EventHandlingExample /> </div> ); } export default App;
输出
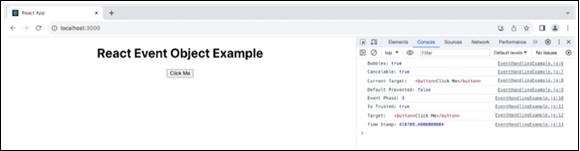
在上面的示例代码中,当我们单击“单击我”按钮时,事件属性将记录在控制台中。e.preventDefault() 行显示了如何阻止按钮执行其默认操作。
标准事件方法
让我们浏览一下 React 事件对象实现的一些标准事件方法 -
序号 | 方法和描述 |
---|---|
1 | e.preventDefault() 此方法可以阻止事件的默认行为。例如,您可以使用它来阻止表单提交。 |
2 | e.stopPropagation() 这会阻止事件进一步向上遍历 React 组件树。这有助于防止其他事件处理程序运行。 |
3 | e.isDefaultPrevented() 查看是否调用了 preventDefault()。返回布尔值。 |
4 | e.isPropagationStopped() 查看是否调用了 stopPropagation()。返回布尔值。 |
示例 - 使用事件方法
让我们创建一个基本的 React 应用,该应用使用事件对象方法来阻止默认行为并在单击按钮时停止事件传播。确保项目包含 React 和 ReactDOM。创建一个名为 EventMethodsExample 的新 React 组件,该组件显示事件对象方法的使用 -
EventMethodsExample.js
import React from 'react'; class EventMethodsExample extends React.Component { handleClick = (e) => { // Prevent the default behavior of the button e.preventDefault(); // Stop the event from propagating e.stopPropagation(); } render() { return ( <div> <button onClick={this.handleClick}>Click Me</button> <p>Clicking the button will prevent the default behavior and stop event propagation.</p> </div> ); } } export default EventMethodsExample;
App.js
import React from 'react'; import './App.css'; import EventMethodsExample from './EventMethodsExample'; function App() { return ( <div className="App"> <h1>React Event Object Methods Example</h1> <EventMethodsExample /> </div> ); } export default App;
输出
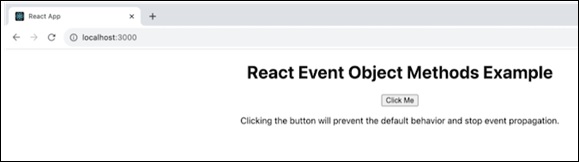
当我们运行 React 应用程序后单击“单击我”按钮时,它会阻止默认行为(如表单提交)并阻止事件进一步向上传播到 React 组件树。
此示例演示如何使用事件对象的 preventDefault() 和 stopPropagation() 方法来管理事件行为。
示例 - 使用事件对象和方法
让我们再创建一个示例应用程序来演示 React 事件对象的使用。在此示例中,我们将创建一个简单的 React 应用程序,该应用程序响应按键事件。
KeyPressExample.js
import React from 'react'; class KeyPressExample extends React.Component { handleKeyPress = (e) => { // Log the key that was pressed console.log('Pressed Key:', e.key); } render() { return ( <div> <h2>React Key Press Event Example</h2> <input type="text" placeholder="Press a key" onKeyPress={this.handleKeyPress} /> </div> ); } } export default KeyPressExample;
App.js
import React from 'react'; import './App.css'; import EventHandlingExample from './EventHandlingExample'; import EventMethodsExample from './EventMethodsExample'; import KeyPressExample from './KeyPressExample'; // Import the new component function App() { return ( <div> <h1>React Event Examples</h1> <EventHandlingExample /> <EventMethodsExample /> <KeyPressExample /> {/* Include the new component */} </div> ); } export default App;
输出
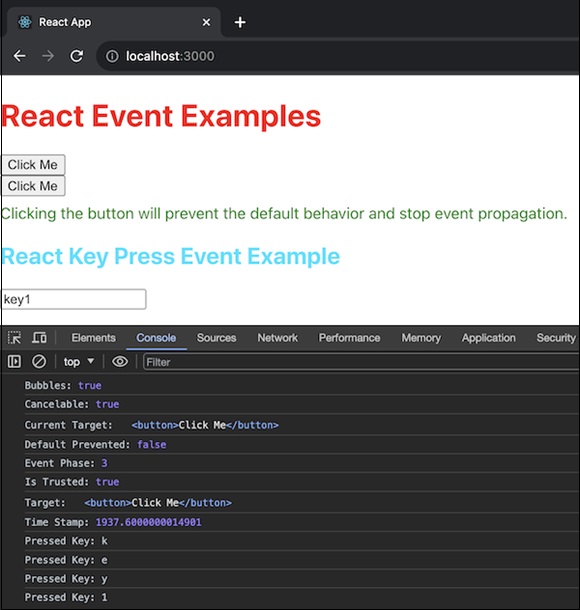
结论
简单来说,React 事件对象帮助我们以可靠且有效的方式处理用户交互,确保我们的应用程序在多个 Web 浏览器和设置中都能正常运行。