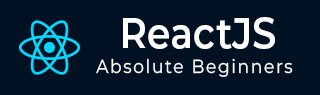
- ReactJS 教程
- ReactJS - 首页
- ReactJS - 简介
- ReactJS - 路线图
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优势与劣势
- ReactJS - 架构
- ReactJS - 创建 React 应用
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用新创建的组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性 (props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建一个事件感知组件
- ReactJS - 在 Expense Manager 应用中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React Hooks 的组件生命周期
- ReactJS - 布局组件
- ReactJS - 分页
- ReactJS - Material UI
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 非受控组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - Keys
- ReactJS - 路由
- ReactJS - Redux
- ReactJS - 动画
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- Hooks
- ReactJS - Hooks 简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义 Hooks
- ReactJS 高级
- ReactJS - 可访问性
- ReactJS - 代码分割
- ReactJS - Context
- ReactJS - 错误边界
- ReactJS - 转发 Refs
- ReactJS - Fragments
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 性能优化
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - 无 ES6 ECMAScript 的 React
- ReactJS - 无 JSX 的 React
- ReactJS - 调和
- ReactJS - Refs 和 DOM
- ReactJS - Render Props
- ReactJS - 静态类型检查
- ReactJS - Strict Mode
- ReactJS - Web Components
- 其他概念
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - 走马灯
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
- ReactJS 有用资源
- ReactJS - 快速指南
- ReactJS - 有用资源
- ReactJS - 讨论
React 中有一个名为 findAllInRenderedTree 的实用工具。此工具帮助我们定位应用程序中特定部分(称为组件)。
可以将我们的 React 应用视为一棵大树,每个组件充当一个分支。findAllInRenderedTree 方法充当友好的浏览器,遍历每个分支并确定它是否匹配测试。
它的工作原理如下
输入 - 浏览器接收两项内容:树(我们的 React 应用)和测试(帮助我们找到所需内容的规则)。
遍历 - 浏览器逐一遍历树的每个组件。
测试 - 浏览器对每个组件进行测试。如果组件通过测试(测试返回 true),浏览器会将其添加到列表中。
结果 - 最终,我们将得到一个通过测试的所有组件的列表。
语法
findAllInRenderedTree( tree, test )
参数
tree (React.Component) - 要遍历的树的根组件。
test (function) - 一个测试函数,它接受输入并返回布尔值。此函数确定是否应将组件包含在结果中。
返回值
此函数返回通过测试的组件数组。
示例
示例 1
要查找待办事项列表中的所有已完成项目,请使用 findAllInRenderedTree。
创建一个包含待办事项列表的 React 应用。要将任务标记为已完成,请在其旁边添加一个复选框。我们将使用 findAllInRenderedTree 来识别并分别显示所有已完成的任务。
import React, { useState } from 'react'; import './App.css'; const TodoApp = () => { const [tasks, setTasks] = useState([ { id: 1, text: 'Complete task 1', completed: false }, { id: 2, text: 'Finish task 2', completed: true }, // Add more tasks if needed ]); const findCompletedTasks = () => { // Using findAllInRenderedTree to find completed tasks const completedTasks = findAllInRenderedTree(tasks, (task) => task.completed); console.log('Completed Tasks:', completedTasks); }; return ( <div className='App'> <h2>Todo List</h2> {tasks.map((task) => ( <div key={task.id}> <input type="checkbox" checked={task.completed} readOnly /> <span>{task.text}</span> </div> ))} <button onClick={findCompletedTasks}>Find Completed Tasks</button> </div> ); }; export default TodoApp;
输出
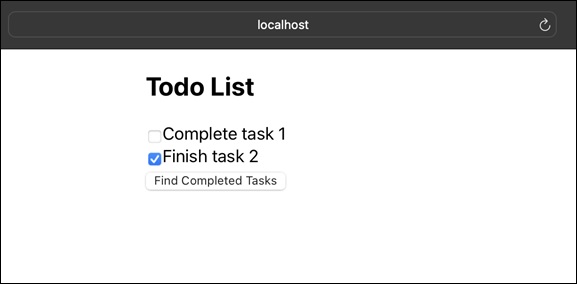
示例 2
在这个应用中,我们必须识别并显示调色板中与颜色相关的所有组件,因此我们将使用 findAllInRenderedTree。
使用调色板创建一个 React 应用,其中每种颜色都作为一个组件。要查找并显示所有颜色组件,请使用 findAllInRenderedTree。
import React from 'react'; import './App.css'; const ColorPaletteApp = () => { const colors = ['red', 'blue', 'green', 'yellow', 'purple']; const findColorComponents = () => { // Using findAllInRenderedTree to find color components const colorComponents = findAllInRenderedTree(colors, (color) => color); console.log('Color Components:', colorComponents); }; return ( <div className='App'> <h2>Color Palette</h2> {colors.map((color) => ( <div key={color} style={{ backgroundColor: color, height: '50px', width: '50px' }}></div> ))} <button onClick={findColorComponents}>Find Color Components</button> </div> ); }; export default ColorPaletteApp;
输出
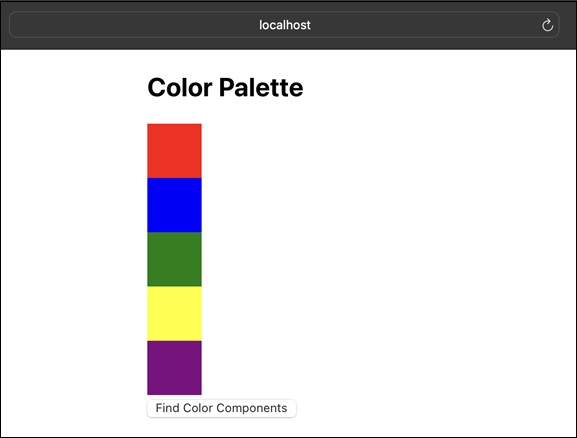
示例 3
在这个应用中,我们将找到并评估交互式测验中的所有响应组件。因此,创建一个包含交互式测验(带有问题和多项选择响应)的 React 应用。使用 findAllInRenderedTree 查找和评估所选答案,并根据正确性提供反馈。
import React, { useState } from 'react'; import './App.css'; const QuizApp = () => { const [questions, setQuestions] = useState([ { id: 1, text: 'What is the capital of France?', options: ['Berlin', 'London', 'Paris'], correctAnswer: 'Paris' }, { id: 2, text: 'Which planet is known as the Red Planet?', options: ['Earth', 'Mars', 'Venus'], correctAnswer: 'Mars' }, ]); const checkAnswers = () => { // Using findAllInRenderedTree to find selected answers const selectedAnswers = findAllInRenderedTree(questions, (question) => question.selectedAnswer); console.log('Selected Answers:', selectedAnswers); }; const handleOptionClick = (questionId, selectedOption) => { setQuestions((prevQuestions) => prevQuestions.map((question) => question.id === questionId ? { ...question, selectedAnswer: selectedOption } : question ) ); }; return ( <div className='App'> <h2>Interactive Quiz</h2> {questions.map((question) => ( <div key={question.id}> <p>{question.text}</p> {question.options.map((option) => ( <label key={option}> <input type="radio" name={`question-${question.id}`} value={option} onChange={() => handleOptionClick(question.id, option)} /> {option} </label> ))} </div> ))} <button onClick={checkAnswers}>Check Answers</button> </div> ); }; export default QuizApp;
输出
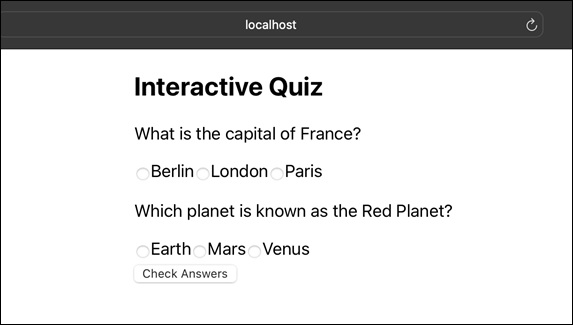
总结
这些示例显示了如何在各种情况下使用 findAllInRenderedTree 方法,从任务管理到浏览调色板和生成交互式测验。每个应用都使用此方法来查找和交互 React 树中的特定组件。