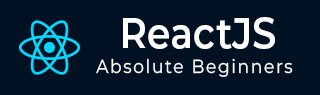
- ReactJS 教程
- ReactJS - 首页
- ReactJS - 简介
- ReactJS - 路线图
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优点与缺点
- ReactJS - 架构
- ReactJS - 创建React应用
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用新创建的组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性 (props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建一个事件感知组件
- ReactJS - 在Expense Manager APP中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理API
- ReactJS - 无状态组件
- ReactJS - 使用React Hooks进行状态管理
- ReactJS - 使用React Hooks进行组件生命周期管理
- ReactJS - 布局组件
- ReactJS - 分页
- ReactJS - Material UI
- ReactJS - Http客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 非受控组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - Keys
- ReactJS - 路由
- ReactJS - Redux
- ReactJS - 动画
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - 表格
- ReactJS - 使用Flux管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- Hooks
- ReactJS - Hooks 简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义 Hooks
- ReactJS 高级
- ReactJS - 可访问性
- ReactJS - 代码分割
- ReactJS - Context
- ReactJS - 错误边界
- ReactJS - 转发 Refs
- ReactJS - Fragments
- ReactJS - 高阶组件
- ReactJS - 集成其他库
- ReactJS - 性能优化
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - 无ES6 ECMAScript的React
- ReactJS - 无JSX的React
- ReactJS - 调和
- ReactJS - Refs和DOM
- ReactJS - Render Props
- ReactJS - 静态类型检查
- ReactJS - Strict Mode
- ReactJS - Web Components
- 其他概念
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - 走马灯
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考API
- ReactJS 有用资源
- ReactJS - 快速指南
- ReactJS - 有用资源
- ReactJS - 讨论
ReactJS - findRenderedDOMComponentWithTag()
在React中,有一个很有用的函数叫做findRenderedDOMComponentWithTag()。这个函数就像一个特殊的侦探,在我们应用的虚拟世界中搜索名为“标签”的特定组件。
当我们使用findRenderedDOMComponentWithTag()时,我们是在告诉我们的计算机程序在应用程序的虚拟表示(称为DOM(文档对象模型))中找到一个特定的标签。
该函数检查该确切的标签。最有趣的部分是它期望找到完全匹配项。如果有多个或没有匹配项,它将抛出异常。
语法
findRenderedDOMComponentWithTag( tree, tagName )
参数
tree − 它充当我们虚拟环境的地图,显示所有元素以及它们之间的关联方式。
tagName − 这是我们在虚拟环境中查找的标签的名称。
返回值
findRenderedDOMComponentWithTag()函数给出一个单一的结果。当我们使用此函数时,我们是在告诉我们的计算机程序在我们的应用程序的DOM中找到一个特定的HTML标签(如<div>,<span>等)。该方法期望只识别一个与提供的标签匹配的项。如果它检测到多个或没有匹配项,它将抛出异常,表明某些内容不符合预期。
示例
示例 - 查找标题
假设我们有一本虚拟书,我们想找到标题,它是一个标题(h1)标签。为此,我们将使用findRenderedDOMComponentWithTag(tree, 'h1')。以下是此应用程序的代码:
// Import required libraries import React from 'react'; import { render } from '@testing-library/react'; import { findRenderedDOMComponentWithTag } from 'testing-library'; // App component const App = () => { return ( <div> <h1>Title of the Book</h1> </div> ); }; // Test case const { container } = render(<App1 />); const headingElement = findRenderedDOMComponentWithTag(container, 'h1'); console.log(headingElement.textContent);
输出
Title of the Book
该程序的目标是使用findRenderedDOMComponentWithTag()函数查找并显示该标题。当我们要求应用程序找到标题(h1标签)时,它应该返回短语“书名”。
示例 - 定位按钮
让我们假设在这个应用程序中我们有一堆按钮。要查找并与特定按钮进行交互,请使用findRenderedDOMComponentWithTag(tree, 'button')。
// Import necessary libraries import React from 'react'; import { render } from '@testing-library/react'; import { findRenderedDOMComponentWithTag } from 'testing-library'; // App component const App = () => { return ( <div> <button onClick={() => console.log('Button 1 is clicked')}>Button 1</button> <button onClick={() => console.log('Button 2 is clicked')}>Button 2</button> </div> ); }; // Test case const { container } = render(<App />); const buttonElement = findRenderedDOMComponentWithTag(container, 'button'); buttonElement.click();
输出
Button 1 is clicked
程序中有两个按钮,一个标记为“按钮1”,另一个标记为“按钮2”。这里的目标是使用findRenderedDOMComponentWithTag()函数查找并与这些按钮进行交互。当我们按下按钮时,它应该显示一条消息,例如“已点击按钮1”。
示例3
让我们创建一个应用程序,其中我们有一些图像。要找到特定图像,我们将使用findRenderedDOMComponentWithTag(tree, 'img')。
// Import required libraries import React from 'react'; import { render } from '@testing-library/react'; import { findRenderedDOMComponentWithTag } from 'testing-library'; // App component const App = () => { return ( <div> <img src="image1.jpg" alt="This is Image 1" /> <img src="image2.jpg" alt="This is Image 2" /> </div> ); }; // Test case const { container } = render(<App />); const imageElement = findRenderedDOMComponentWithTag(container, 'img'); console.log(imageElement.getAttribute('alt'));
输出
This is Image 1
在这个应用程序中,有两张照片,每张照片显示不同的内容。目标是使用findRenderedDOMComponentWithTag()函数识别并显示有关这些图像的信息。例如,当我们找到一张图片时,它应该告诉我们它是什么。如果它是第一张图片,它可以说类似于“这是图片1”。
总结
findRenderedDOMComponentWithTag()方法充当数字浏览器,帮助我们在应用程序的虚拟环境中找到特定项目。它确保我们得到我们想要的东西。因此,此功能使我们的编码体验更加轻松。记住要小心使用它,并期望它只找到一件东西。如果有多于或少于一件,它会通知我们。