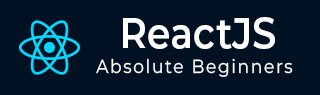
- ReactJS 教程
- ReactJS - 首页
- ReactJS - 简介
- ReactJS - 路线图
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优点与缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用新创建的组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性 (props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建一个事件感知组件
- ReactJS - 在 Expense Manager 应用中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React Hooks 的组件生命周期
- ReactJS - 布局组件
- ReactJS - 分页
- ReactJS - Material UI
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 非受控组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - Keys
- ReactJS - 路由
- ReactJS - Redux
- ReactJS - 动画
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- Hooks
- ReactJS - Hooks 简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义 Hooks
- ReactJS 高级
- ReactJS - 可访问性
- ReactJS - 代码分割
- ReactJS - Context
- ReactJS - 错误边界
- ReactJS - 转发 Refs
- ReactJS - Fragments
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 性能优化
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - 无需 ES6 ECMAScript 的 React
- ReactJS - 无需 JSX 的 React
- ReactJS - Reconciliation (协调)
- ReactJS - Refs 和 DOM
- ReactJS - Render Props
- ReactJS - 静态类型检查
- ReactJS - Strict Mode (严格模式)
- ReactJS - Web Components
- 其他概念
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - 轮播图
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
- ReactJS 有用资源
- ReactJS - 快速指南
- ReactJS - 有用资源
- ReactJS - 讨论
ReactJS - forceUpdate() 方法
React 中的组件充当网站或应用程序的构建块。它们可以显示多种内容并执行不同的操作。通常,组件只有在其自身状态或从外部获取的信息(称为 props)发生变化时才会更改或重新渲染。
React 组件只有在其状态或传递给它的 props 发生变化时才会重新渲染,但是如果我们需要在任何数据变化时重新渲染组件,则可以使用 React 的 forceUpdate() 方法。调用 forceUpdate() 将强制重新渲染组件,忽略 shouldComponentUpdate() 方法,而是调用组件的 render() 方法。
因此,forceUpdate() 是一种使 React 组件刷新自身的方法,即使它认为不需要刷新也是如此。
语法
forceUpdate(callbackFunc)
参数
callbackFunc − 这是一个可选的回调函数。如果我们定义一个回调函数,React 将在更新提交后执行它。
返回值
此函数不返回任何值。
示例
示例 1
让我们创建一个基本的 React 应用,展示如何使用 forceUpdate 函数。在这个示例中,我们将有一个组件从外部 API 获取一个随机数,并在单击按钮时更新 UI。
NumberDisplay.js
import React, { Component } from 'react'; class NumberDisplay extends Component { constructor() { super(); this.state = { randomNumber: null, }; } fetchData = async () => { // An API call to get a random number const response = await fetch('https://www.random.org/integers/?num=1&min=1&max=100&format=plain'); const data = await response.text(); this.setState({ randomNumber: parseInt(data) }); }; forceUpdateHandler = () => { // Use forceUpdate to manually update the component this.forceUpdate(); }; render() { return ( <div> <h1>Random Number: {this.state.randomNumber}</h1> <button onClick={this.fetchData}>Fetch New Number</button> <button onClick={this.forceUpdateHandler}>Force Update</button> </div> ); } } export default NumberDisplay;
App.js
import React from 'react'; import NumberDisplay from './NumberDisplay'; function App() { return ( <div> <h1>ForceUpdate Example App</h1> <NumberDisplay /> </div> ); } export default App;
输出
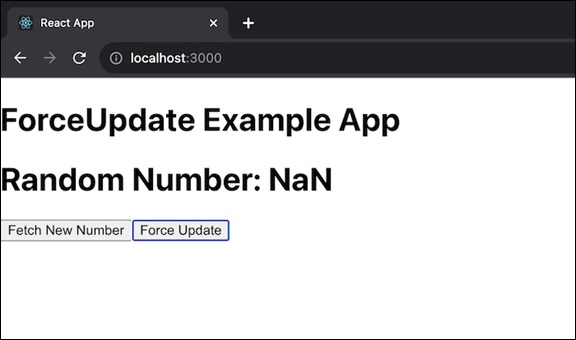
示例 2
假设我们有一个简单的计数应用程序。这个计数器应用程序在屏幕上显示一个数字,从 0 开始。我们可以通过单击“增加计数”按钮来增加计数。但是,还有一个标记为“强制更新”的按钮。当我们单击它时,即使我们没有增加计数,应用程序也会刷新并再次显示当前计数。这里使用 forceUpdate() 函数来确保显示始终是最新的。
import React from 'react'; class CounterApp extends React.Component { constructor() { super(); this.state = { count: 0 }; } increaseCount() { this.setState({ count: this.state.count + 1 }); } forceUpdateCounter() { this.forceUpdate(); } render() { return ( <div> <p>Count: {this.state.count}</p> <button onClick={() => this.increaseCount()}>Increase Count</button> <button onClick={() => this.forceUpdateCounter()}>Force Update</button> </div> ); } } export default CounterApp;
输出
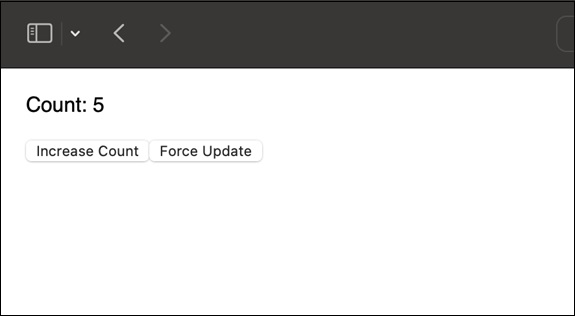
示例 3
现在让我们创建一个消息应用程序。此程序在屏幕上显示一条消息,最初设置为“Hello, world!”。通过单击“更改消息”按钮,我们可以更改消息。但是有一个“强制更新”按钮。当我们单击此按钮时,应用程序将刷新并显示当前消息。为了实现这种刷新行为,需要 forceUpdate() 函数。
import React from "react"; class MessageApp extends React.Component { constructor() { super(); this.state = { message: "Hello, world!" }; } changeMessage(newMessage) { this.setState({ message: newMessage }); } forceUpdateMessage() { this.forceUpdate(); } render() { return ( <div> <p>{this.state.message}</p> <button onClick={() => this.changeMessage("New Message")}> Change Message </button> <button onClick={() => this.forceUpdateMessage()}>Force Update</button> </div> ); } } export default MessageApp;
输出
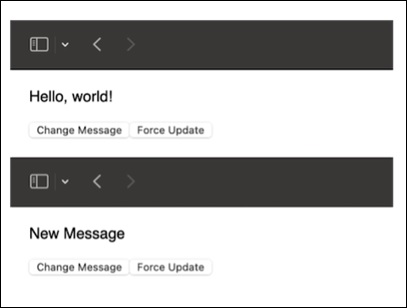
限制
如果我们使用 forceUpdate,React 将在不检查 shouldComponentUpdate 的情况下刷新。
总结
对于 React 组件,forceUpdate 的作用类似于刷新按钮。虽然我们不会经常使用它,但在组件从外部来源接收数据时它会很有用。记住,通常最好让 React 自动处理更新,但是如果我们需要更多控制,forceUpdate 是可用的。