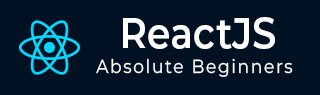
- ReactJS 教程
- ReactJS - 首页
- ReactJS - 简介
- ReactJS - 路线图
- ReactJS - 安装
- ReactJS - 功能特性
- ReactJS - 优点与缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用新创建的组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性 (props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建一个事件感知组件
- ReactJS - 在 Expense Manager 应用中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React Hooks 的组件生命周期
- ReactJS - 布局组件
- ReactJS - 分页
- ReactJS - Material UI
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 非受控组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - Keys
- ReactJS - 路由
- ReactJS - Redux
- ReactJS - 动画
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - 命令行工具命令
- ReactJS - 构建和部署
- ReactJS - 示例
- Hooks
- ReactJS - Hooks 简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义 Hooks
- ReactJS 高级
- ReactJS - 可访问性
- ReactJS - 代码分割
- ReactJS - Context
- ReactJS - 错误边界
- ReactJS - 转发 Refs
- ReactJS - Fragments
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 性能优化
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - 无 ES6 ECMAScript 的 React
- ReactJS - 无 JSX 的 React
- ReactJS - 调和
- ReactJS - Refs 和 DOM
- ReactJS - Render Props
- ReactJS - 静态类型检查
- ReactJS - Strict Mode
- ReactJS - Web Components
- 其他概念
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - 走马灯
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
- ReactJS 有用资源
- ReactJS - 快速指南
- ReactJS - 有用资源
- ReactJS - 讨论
ReactJS - isCompositeComponent()
React JS 的核心思想是将我们的应用程序分解成更小的组件,并且每个组件都有其自身的生命周期。React 提供了内置方法,我们可以在组件生命周期的不同阶段使用这些方法。
在 React JS 中,isCompositeComponent() 方法主要用于检查实例是否为用户自定义组件。它帮助我们确定特定元素是开发者创建的自定义组件,还是系统内置组件。因此,我们可以说它帮助我们找出应用程序的特定部分是我们创建的组件,还是系统自带的。
语法
isCompositeComponent(instance)
参数
instance − 这是我们要检查的元素。此方法用于检查元素或组件实例是否为用户自定义组件。因此,我们可以检查任何 React 元素或组件实例,以查看它是否是自定义组件还是内置组件。
返回值
它返回一个布尔值,如果提供的元素是用户自定义组件,则返回 true,否则返回 false。
示例
示例 1
现在,我们将创建一个简单的 React 应用,展示如何使用此方法。我们将创建一个名为 App 的基本组件,并在其中使用 isCompositeComponent()。
import React from 'react'; import { isCompositeComponent } from 'react-dom/test-utils'; // Define App Component const App = () => { // Function to show isCompositeComponent() function myFunction() { var a = isCompositeComponent(el); console.log("It is an element :", a); } const el = <div> <h1>element</h1> </div> // Return our JSX code return ( <div> <h1>React App to show isCompositeComponent method</h1> <button onClick={myFunction}>Click me !!</button> </div> ); } export default App;
输出
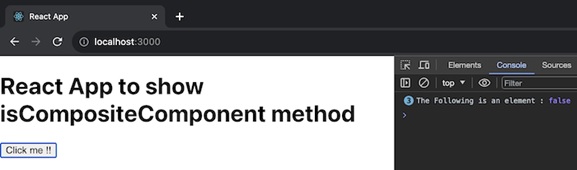
在代码中,我们看到一个名为“App”的简单组件。“App”组件内有一个名为“myFunction”的函数,它使用 isCompositeComponent() 来判断“el”元素是否为用户自定义组件。当我们点击“Click me!!”按钮时,结果将记录在控制台中。
示例 2
此应用程序包含一个名为 FunctionalComponent 的简单函数式组件。主 App 组件渲染一个按钮,当点击该按钮时,它将使用 isCompositeComponent() 方法检查 FunctionalComponent 是否为组合组件。
import React from 'react'; import { isCompositeComponent } from 'react-dom/test-utils'; const FunctionalComponent = () => { return <div>Hello from FunctionalComponent!</div>; }; const App = () => { function checkComponent() { const isComposite = isCompositeComponent(<FunctionalComponent />); console.log("Is FunctionalComponent a composite component?", isComposite); } return ( <div> <h1>React App to show isCompositeComponent method</h1> <button onClick={checkComponent}>Check FunctionalComponent</button> </div> ); }; export default App;
输出
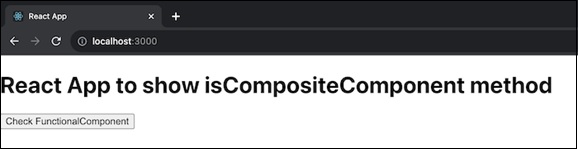
示例 3
此应用程序包含一个名为 ClassComponent 的简单类组件。主 App 组件渲染一个按钮,当点击该按钮时,它将使用 isCompositeComponent() 方法检查 ClassComponent 是否为组合组件。
import React, { Component } from 'react'; import { isCompositeComponent } from 'react-dom/test-utils'; class ClassComponent extends Component { render() { return <div>Hello from ClassComponent!</div>; } } const App = () => { function checkComponent() { const isComposite = isCompositeComponent(<ClassComponent />); console.log("Is ClassComponent a composite component?", isComposite); } return ( <div> <h1>React App to show isCompositeComponent method</h1> <button onClick={checkComponent}>Check ClassComponent</button> </div> ); }; export default App;
输出
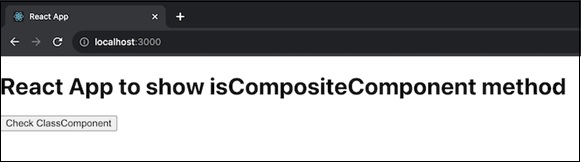
总结
isCompositeComponent() 方法可用于确定 React 应用的特定元素是否是用户创建的组件。对于用户自定义元素,它返回 true,否则返回 false。