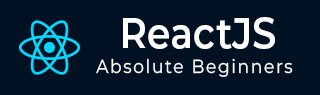
- ReactJS 教程
- ReactJS - 首页
- ReactJS - 简介
- ReactJS - 路线图
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优点与缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用新创建的组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性 (props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建一个事件感知组件
- ReactJS - 在 Expense Manager 应用中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React Hooks 进行组件生命周期管理
- ReactJS - 布局组件
- ReactJS - 分页
- ReactJS - Material UI
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 非受控组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - Keys
- ReactJS - 路由
- ReactJS - Redux
- ReactJS - 动画
- ReactJS - Bootstrap
- ReactJS - 地图
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- Hooks
- ReactJS - Hooks 简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义 Hooks
- ReactJS 高级
- ReactJS - 可访问性
- ReactJS - 代码分割
- ReactJS - 上下文
- ReactJS - 错误边界
- ReactJS - 转发 Refs
- ReactJS - 片段
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 优化性能
- ReactJS - Profiler API
- ReactJS - 端口
- ReactJS - 无 ES6 ECMAScript 的 React
- ReactJS - 无 JSX 的 React
- ReactJS - 调和
- ReactJS - Refs 和 DOM
- ReactJS - 渲染 Props
- ReactJS - 静态类型检查
- ReactJS - 严格模式
- ReactJS - Web Components
- 其他概念
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - 轮播图
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
- ReactJS 有用资源
- ReactJS - 快速指南
- ReactJS - 有用资源
- ReactJS - 讨论
ReactJS - isCompositeComponentWithType() 方法
众所周知,在 React 中,每个组件都有其自己的生命周期,这意味着它们在项目中运行时会经历不同的阶段。React 提供了内置方法来控制这些过程。
现在让我们看看 isCompositeComponentWithType() 方法。此方法告诉我们程序的给定元素是否为 React 组件。以下是我们可以使用它的方法:
语法
isCompositeComponentWithType( instance, componentClass )
参数
在 React 中,isCompositeComponentWithType 方法需要两个参数:
instance - 此参数提供我们要测试的组件实例。
componentClass - 此参数表示 React 组件类。
返回值
该函数将确定实例是否为此组件类的实例。该函数产生一个布尔结果:
如果实例是类型与 componentClass 匹配的组件,则返回 true。
如果实例不是提供的 componentClass 的组件,则返回 false。
示例
示例 - 简单应用
让我们创建一个简单的 React 应用,其中包含一个组件,然后在测试中使用 isCompositeComponentWithType()。我们将有一个简单的 React 组件 (MyComponent) 和一个测试代码。该测试使用 isCompositeComponentWithType() 检查渲染的组件是否为类型为“div”的复合组件。此应用的代码如下所示:
MyComponent.js
import React from 'react'; import { render } from '@testing-library/react'; import { isCompositeComponentWithType } from 'react-dom/test-utils'; const MyComponent = () => { return ( <div> <h1>Hello, I'm a simple component!</h1> </div> ); }; export default MyComponent; test('MyComponent is a composite component of type "div"', () => { const { container } = render(<MyComponent />); const isComposite = isCompositeComponentWithType(container.firstChild, 'div'); expect(isComposite).toBe(true); });
输出
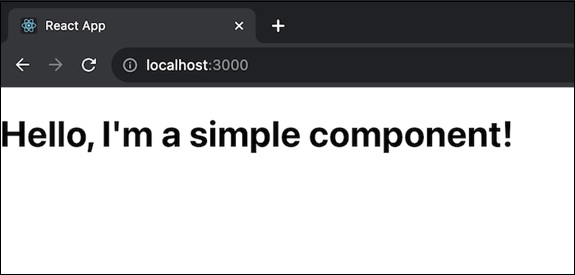
示例 - 测试按钮应用
这是包含 isCompositeComponentWithType() 方法的 App.js 文件的完整代码,以显示该方法的用法。
import React from 'react'; import { isCompositeComponentWithType } from 'react-dom/test-utils'; // Define App Component const App = () => { // Function to show isCompositeComponentWithType() function myFunction() { var a = isCompositeComponentWithType(el); console.log("Is the following element a composite component? " + a); } // The element for testing const el = <div> <h1>element</h1> </div> // Return the user interface return ( <div id='el'> <h1>React isCompositeComponentWithType() method usage</h1> <button onClick={myFunction}> Click Me !! </button> </div> ); } export default App;
输出
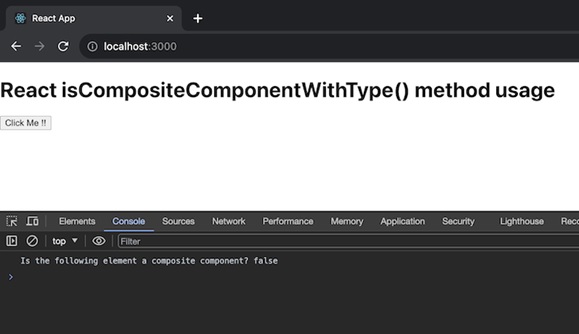
此代码显示了一个 App 组件,该组件具有一个 myFunction 函数来显示 isCompositeComponentWithType() 方法。当我们点击应用中的按钮时,它将检查 el 元素是否为复合组件并记录结果。
假设我们正在创建一个数字店面,并且想知道我们网站的特定部分是否属于产品列表类型。我们可以通过调用 isCompositeComponentWithType() 函数来实现这一点。首先,我们导入所需的工具,构建一个验证函数,创建一个元素(产品列表),然后在我们的网站上显示它并带有一个测试按钮。
示例 - 从 API 获取数据
现在我们将有一个应用从 API 获取和显示数据。还有一个测试文件,它使用 isCompositeComponentWithType() 测试 FetchData 组件是否渲染从 API 获取的数据。它使用 @testing-library/react 中的 render 函数来渲染组件,并使用 waitFor 来等待异步 fetch 调用完成。此应用的代码如下所示:
// FetchData.js import React, { useState, useEffect } from 'react'; const FetchData = () => { const [data, setData] = useState([]); useEffect(() => { const fetchData = async () => { try { const response = await fetch('https://jsonplaceholder.typicode.com/todos'); const result = await response.json(); setData(result); } catch (error) { console.error('Error fetching data:', error); } }; fetchData(); }, []); return ( <div> <h1>Fetching Data from an API</h1> <ul> {data.map(item => ( <li key={item.id}>{item.title}</li> ))} </ul> </div> ); }; export default FetchData;
FetchData.test.js
import React from 'react'; import { render, waitFor } from '@testing-library/react'; import FetchData from './FetchData'; // fetch function global.fetch = jest.fn(() => Promise.resolve({ json: () => Promise.resolve([{ id: 1, title: 'Sample Todo' }]), }) ); test('FetchData renders data from API', async () => { const { getByText } = render(<FetchData />); // Wait for the fetch call await waitFor(() => expect(fetch).toHaveBeenCalledTimes(1)); const todoItem = getByText('Sample Todo'); expect(todoItem).toBeInTheDocument(); });
输出
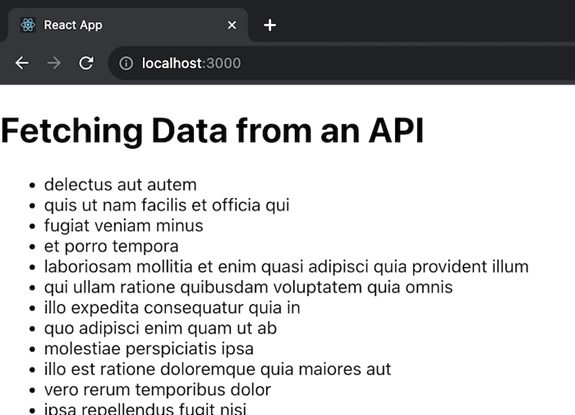
总结
isCompositeComponentWithType() 是一个有用的工具,可以识别应用中的 React 组件类型并在测试或调试情况下检查其有效性。我们创建了三个不同的应用来展示此函数的用法。