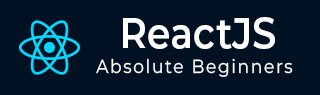
- ReactJS 教程
- ReactJS - 首页
- ReactJS - 简介
- ReactJS - 路线图
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优点与缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用新创建的组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性 (props)
- ReactJS - 使用属性创建组件
- ReactJS - props 校验
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建一个事件感知组件
- ReactJS - 在 Expense Manager 应用中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React Hooks 进行组件生命周期管理
- ReactJS - 布局组件
- ReactJS - 分页
- ReactJS - Material UI
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 非受控组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - Keys
- ReactJS - 路由
- ReactJS - Redux
- ReactJS - 动画
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- Hooks
- ReactJS - Hooks 简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义 Hooks
- ReactJS 高级
- ReactJS - 可访问性
- ReactJS - 代码分割
- ReactJS - Context
- ReactJS - 错误边界
- ReactJS - 转发 Refs
- ReactJS - Fragments
- ReactJS - 高阶组件
- ReactJS - 集成其他库
- ReactJS - 性能优化
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - 无 ES6 ECMAScript 的 React
- ReactJS - 无 JSX 的 React
- ReactJS - 调和
- ReactJS - Refs 和 DOM
- ReactJS - Render Props
- ReactJS - 静态类型检查
- ReactJS - Strict Mode
- ReactJS - Web Components
- 其他概念
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - 轮播图
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
- ReactJS 有用资源
- ReactJS - 快速指南
- ReactJS - 有用资源
- ReactJS - 讨论
ReactJS - renderToString() 方法
将 React 组件渲染到 HTML 是一个常见的操作,尤其是在处理服务器端渲染 (SSR) 时。这可以通过使用 renderToString 方法来实现。
什么是 renderToString?
React 的 renderToString 函数将 React 组件渲染成 HTML 字符串。在服务器上,它主要用于在将我们的 React 应用提供给客户端作为初始服务器响应的一部分之前预渲染我们的 React 应用。这意味着我们的网站加载速度更快,并且更易于搜索引擎优化。
语法
renderToString(reactNode)
参数
reactNode − 要渲染到 HTML 的 React 节点。例如 <App />。
返回值
它返回一个 HTML 字符串。
示例
示例 - 问候应用
在这个代码中,我们将创建一个名为 Greetings 的 React 组件来显示简单的问候消息。它将使用服务器端渲染将组件转换为 HTML 字符串并将该字符串记录到控制台。执行时,我们将看到 Greetings 组件的初始 HTML 表示,其中包含欢迎标题和鼓励使用 React 进行构建的消息。
// Import necessary modules const React = require('react'); const ReactDOMServer = require('react-dom/server'); // Define a component with greetings const Greetings = () => ( <div> <h1>Welcome to React!</h1> <p>Hope you enjoy building with it.</p> </div> ); export default Greetings; // Render the component to a string const htmlString2 = ReactDOMServer.renderToString(<Greetings />); // Log the result console.log(htmlString2);
输出
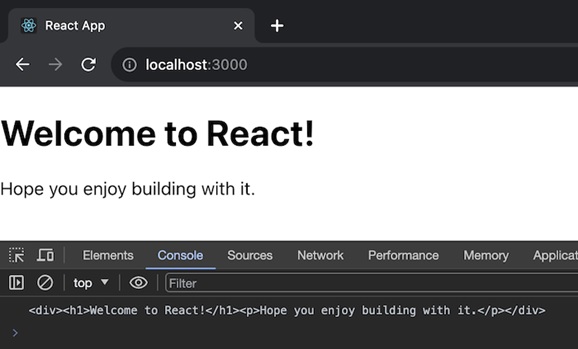
示例 - 计数器应用
在这个应用中,我们将创建一个 React 组件 (Counter),它表示一个带有递增按钮的简单计数器。它将使用服务器端渲染将组件转换为 HTML 字符串并将该字符串记录到控制台。执行时,我们将看到 Counter 组件的初始 HTML 表示,计数为 0。
// Import necessary modules const React = require('react'); const ReactDOMServer = require('react-dom/server'); // Define a component with a simple counter class Counter extends React.Component { constructor() { super(); this.state = { count: 0 }; } render() { return ( <div> <h1>Counter: {this.state.count}</h1> <button onClick={() => this.setState({ count: this.state.count + 1 })}> Increment </button> </div> ); } } export default Counter; // Render the component to a string const htmlString3 = ReactDOMServer.renderToString(<Counter />); // Log the result console.log(htmlString3);
输出
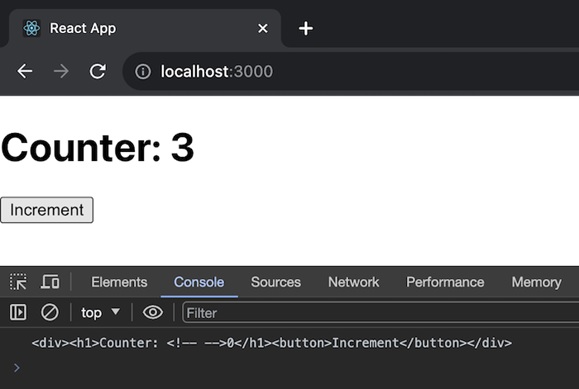
示例 - 服务器和客户端
我们可以通过两种不同的方式使用 renderToString:第一种是在服务器上,第二种是在客户端。因此,我们将逐一讨论这两种方式。
在服务器上
首先,我们将从 'react-dom/server' 中导入 renderToString 方法。并使用它将我们的 React 应用渲染到 HTML。
import { renderToString } from 'react-dom/server'; const html = renderToString(<App />);
在客户端
我们需要一个单独的函数,例如 hydrateRoot,才能使服务器生成的 HTML 具有交互性。
首先,验证我们的响应是否包含服务器渲染的 HTML,并且我们是否包含了在客户端加载我们的 React 组件和 hydrateRoot 所需的脚本。
服务器端渲染 (Node.js/Express)
import express from 'express'; import React from 'react'; import { renderToString } from 'react-dom/server'; import App from './App'; // Import the main React component const app = express(); app.get('/', (req, res) => { // Server-side rendering const html = renderToString(<App />); res.send(` <!DOCTYPE html> <html> <head> <title>Server-Side Rendering</title> </head> <body> <div id="root">${html}</div> <script src="/client.js"></script> </body> </html> `); }); app.listen(3000, () => { console.log('Server is running on https://127.0.0.1:3000'); });
客户端 (client.js)
import React from 'react'; import { hydrateRoot } from 'react-dom'; // Import hydrateRoot import App from './App'; // Import the main React component // Find the root element const rootElement = document.getElementById('root'); // Hydrate the server-rendered HTML hydrateRoot(rootElement, <App />);
使用此方法,我们可以实现服务器端渲染,同时使内容在客户端具有交互性,结合两种方式的优点。
局限性
React Suspense 仅部分支持 renderToString。如果组件等待数据,renderToString 会立即发送其回退内容作为 HTML。
虽然它可以在浏览器中使用,但不建议将其用于客户端应用程序。客户端渲染有更好的选择。
总结
renderToString 是一个有用的 React 方法,用于在服务器端将我们的组件更改为 HTML,从而提高网站性能和搜索引擎优化。请记住,它可能不是客户端代码的最佳选择。