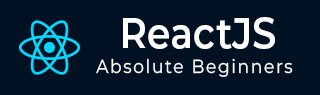
- ReactJS 教程
- ReactJS - 首页
- ReactJS - 简介
- ReactJS - 路线图
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优点与缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用新创建的组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性 (props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建一个事件感知组件
- ReactJS - 在 Expense Manager APP 中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React Hooks 进行组件生命周期管理
- ReactJS - 布局组件
- ReactJS - 分页
- ReactJS - Material UI
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 非受控组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - Keys
- ReactJS - 路由
- ReactJS - Redux
- ReactJS - 动画
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- Hooks
- ReactJS - Hooks 简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义 Hooks
- ReactJS 高级
- ReactJS - 可访问性
- ReactJS - 代码分割
- ReactJS - Context
- ReactJS - 错误边界
- ReactJS - 转发 Refs
- ReactJS - Fragments
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 性能优化
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - 无 ES6 ECMAScript 的 React
- ReactJS - 无 JSX 的 React
- ReactJS - 协调
- ReactJS - Refs 和 DOM
- ReactJS - Render Props
- ReactJS - 静态类型检查
- ReactJS - Strict Mode
- ReactJS - Web Components
- 其他概念
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - 走马灯
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
- ReactJS 有用资源
- ReactJS - 快速指南
- ReactJS - 有用资源
- ReactJS - 讨论
ReactJS - 静态 defaultProps 属性
React 是一个流行的用户界面开发库。有时在创建 React 应用时,我们可能希望为某些属性设置默认设置。“defaultProps”的概念在这种情况下就派上用场了。
什么是 defaultProps?
DefaultProps 是 React 的一个特性,允许我们为组件的属性 (或 props) 提供默认值。当未提供 prop、prop 未定义或缺少 prop 时,将使用这些默认值。需要注意的是,如果 prop 显式设置为 null,则不会应用 defaultProps。
defaultProps 的用法
class Button extends Component { static defaultProps = { color: 'blue' }; render() { return <button className={this.props.color}>Click me</button>; } }
在此代码中,我们有一个 Button 组件,它接受一个 'color' prop。如果未提供 'color' prop 或其未定义,defaultProps 会介入并默认将 'color' prop 设置为 'blue'。
defaultProps 实践示例
如果没有提供 'color' prop,Button 组件将默认设置为 'blue'。
<Button /> {/* The color is "blue" */}
即使我们将 'color' prop 直接设置为 undefined,defaultProps 仍然生效,将 'color' 的默认值设置为 'blue'。
<Button color={undefined} /> {/* The color is "blue" */}
如果我们将 'color' prop 更改为 null,defaultProps 不会覆盖它,'color' 将为 null。
<Button color={null} /> {/* The color is null */}
如果我们为 'color' prop 提供一个值,则将使用该值而不是默认值。
<Button color="red" /> {/* The color is "red" */}
示例
示例 - Button 组件应用
我们将创建一个 React 应用,其中我们将显示一个具有蓝色颜色的按钮组件,以展示 defaultProps 的用法。
现在打开项目,并使用代码编辑器导航到项目目录中的 src 文件夹。替换 App.js 文件中的以下代码:
import React, { Component } from 'react'; import './App.css'; // Import the CSS file class Button extends Component { static defaultProps = { color: 'blue' }; render() { return <button className={`button ${this.props.color}`}>Click me</button>; } } class App extends Component { render() { return ( <div className="App"> <Button /> </div> ); } } export default App;
App.css
.button { background-color: rgb(119, 163, 208); color: white; padding: 5px 20px; border: 2px; margin-top: 25px; border-radius: 5px; cursor: pointer; } .blue { background-color: blue; }
输出
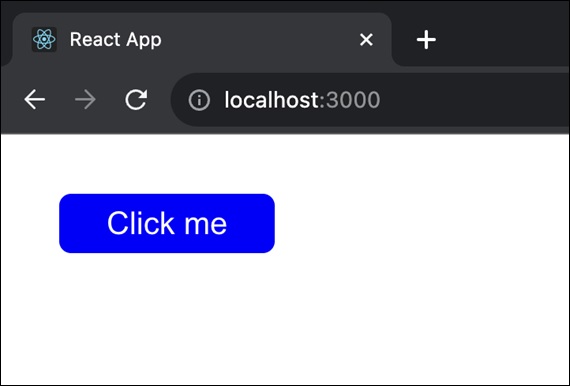
示例 - 简单计数器应用
在这个应用中,我们将显示一个从指定初始值(默认为 0)开始的计数器。用户可以点击一个按钮来增加计数器。此应用将向我们展示如何使用静态 defaultProps 为某些属性提供默认值。此应用的代码如下:
import React, { Component } from 'react'; class Counter extends Component { static defaultProps = { initialValue: 0, }; constructor(props) { super(props); this.state = { count: this.props.initialValue, }; } increment = () => { this.setState((prevState) => ({ count: prevState.count + 1 })); }; render() { return ( <div> <h2>Counter App</h2> <p>Count: {this.state.count}</p> <button onClick={this.increment}>Increment</button> </div> ); } } export default Counter;
输出
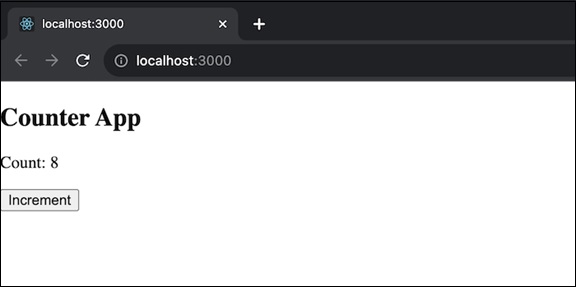
示例 - 切换开关应用
在这个应用中,我们将提供一个可以打开或关闭的切换开关。我们将初始状态设置为打开或关闭(默认为关闭)。用户可以点击一个按钮来切换开关。此应用的代码如下:
import React, { Component } from 'react'; class ToggleSwitch extends Component { static defaultProps = { defaultOn: false, }; constructor(props) { super(props); this.state = { isOn: this.props.defaultOn, }; } toggle = () => { this.setState((prevState) => ({ isOn: !prevState.isOn })); }; render() { return ( <div> <h2>Toggle Switch App</h2> <p>Switch is {this.state.isOn ? 'ON' : 'OFF'}</p> <button onClick={this.toggle}>Toggle</button> </div> ); } } export default ToggleSwitch;
输出
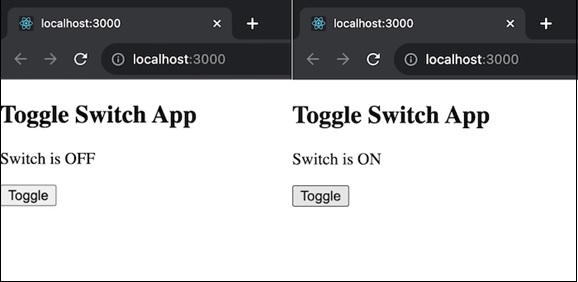
在上面的输出中,我们可以看到有一个名为“Toggle”的按钮,当我们点击它时,消息将根据条件更改。如果消息是“Switch is OFF”,那么点击按钮后,消息将更改为“Switch is ON”。
总结
defaultProps 是 React 中一个有用的特性,它改进了对组件属性默认值的处理。它确保即使缺少或未定义某些 props,我们的组件也能有效地工作。通过定义默认值,我们可以使代码更简洁、更易于使用。