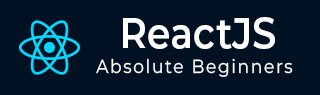
- ReactJS 教程
- ReactJS - 首页
- ReactJS - 简介
- ReactJS - 路线图
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优点和缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用程序
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用新创建的组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性 (props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建一个事件感知组件
- ReactJS - 在 Expense Manager 应用程序中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React Hooks 进行组件生命周期管理
- ReactJS - 布局组件
- ReactJS - 分页
- ReactJS - Material UI
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 非受控组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - Keys
- ReactJS - 路由
- ReactJS - Redux
- ReactJS - 动画
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- Hooks
- ReactJS - Hooks 简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义 Hooks
- ReactJS 高级
- ReactJS - 可访问性
- ReactJS - 代码分割
- ReactJS - 上下文
- ReactJS - 错误边界
- ReactJS - 转发 Refs
- ReactJS - 片段
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 优化性能
- ReactJS - Profiler API
- ReactJS - 端口
- ReactJS - 无 ES6 ECMAScript 的 React
- ReactJS - 无 JSX 的 React
- ReactJS - 调和
- ReactJS - Refs 和 DOM
- ReactJS - 渲染 Props
- ReactJS - 静态类型检查
- ReactJS - 严格模式
- ReactJS - Web Components
- 其他概念
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - 走马灯
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
- ReactJS 有用资源
- ReactJS - 快速指南
- ReactJS - 有用资源
- ReactJS - 讨论
ReactJS - static getDerivedStateFromProps() 方法
React 是一个著名的 JavaScript 库,用于创建用户界面。React 中有一个名为 getDerivedStateFromProps 的方法,看起来很复杂,但让我们将其分解成简单的词语。
在 React 中,getDerivedStateFromProps 方法在组件渲染之前调用,无论是在首次出现时还是在更新时。其目标是确定组件的状态是否需要响应其 props 的更改而更改。
语法
static getDerivedStateFromProps(props, state)
参数
props - 组件将渲染的下一组 props。
state - 组件即将渲染的状态。
返回值
如果需要更新状态,则应返回一个对象;否则,应返回 null。
示例
示例 1
让我们使用 getDerivedStateFromProps 函数创建一个简单的 React 应用程序。在此示例中,我们将创建一个表单,该表单在用户 ID 更改时自动刷新电子邮件地址。
import React, { Component } from 'react'; class Form extends Component { state = { email: this.props.defaultEmail, prevUserID: this.props.userID }; static getDerivedStateFromProps(props, state) { if (props.userID !== state.prevUserID) { return { prevUserID: props.userID, email: props.defaultEmail }; } return null; } handleUserIDChange = (e) => { // Simulate user ID change this.props.onUserIDChange(e.target.value); }; handleEmailChange = (e) => { this.setState({ email: e.target.value }); }; render() { return ( <div> <label> User ID: <input type="text" value={this.props.userID} onChange={this.handleUserIDChange} /> </label> <br /> <label> Email: <input type="text" value={this.state.email} onChange={this.handleEmailChange} /> </label> </div> ); } } class App extends Component { state = { userID: '123', defaultEmail: '[email protected]' }; handleUserIDChange = (newUserID) => { this.setState({ userID: newUserID }); }; render() { return ( <div> <h1>Form App</h1> <Form userID={this.state.userID} defaultEmail={this.state.defaultEmail} onUserIDChange={this.handleUserIDChange} /> </div> ); } } export default App;
输出
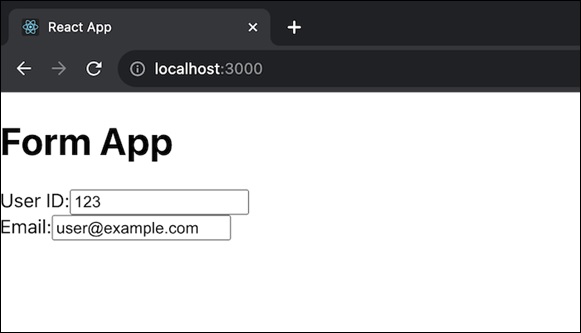
在上面的示例中,Form 组件具有用户 ID 和电子邮件输入。当用户 ID 更改时,getDerivedStateFromProps 函数重置电子邮件。handleUserIDChange 函数模拟用户 ID 更改。App 组件设置 Form 并管理用户 ID 的更改。这是一个基本示例,我们可以对其进行更改以满足我们的特定需求。
示例 2
现在,我们将创建一个简单的计数器应用程序,其中初始计数作为参数给出,并且可以递增计数器。当 prop 更改时,将调用 getDerivedStateFromProps 函数来更新状态。
import React, { Component } from 'react'; import './App.css'; class Counter extends Component { state = { count: this.props.initialCount, }; static getDerivedStateFromProps(props, state) { // Check if the initial count prop has changed if (props.initialCount !== state.count) { // Update the state with the new initial count return { count: props.initialCount, }; } return null; } handleIncrement = () => { this.setState((prevState) => ({ count: prevState.count + 1, })); }; render() { return ( <div> <p>Count: {this.state.count}</p> <button onClick={this.handleIncrement}>Increment</button> </div> ); } } class App extends Component { state = { initialCount: 0, }; handleInitialCountChange = (e) => { const newInitialCount = parseInt(e.target.value, 10); this.setState({ initialCount: newInitialCount }); }; render() { return ( <div className='App'> <h1>Counter App</h1> <label> Initial Count: <input type="number" value={this.state.initialCount} onChange={this.handleInitialCountChange} /> </label> <Counter initialCount={this.state.initialCount} /> </div> ); } } export default App;
输出
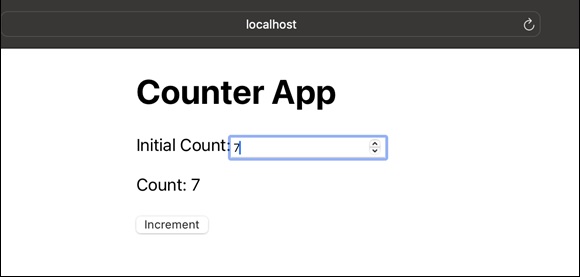
现在,我们有一个简单的 React 应用程序,其中计数器根据初始 count prop 更新,并且 getDerivedStateFromProps 方法用于处理 prop 更改。
示例 3
让我们创建一个简单的 React 应用程序,该应用程序使用生命周期方法 getDerivedStateFromProps 根据用户是否登录来显示消息。因此,在运行应用程序后,我们将能够看到用户已登录或已注销的消息。
import React, { Component } from 'react'; import './App.css'; class LoginStatus extends Component { static getDerivedStateFromProps(props, state) { // Check if the user is logged in const isLoggedIn = props.isLoggedIn; // Display different messages as per the login status return { statusMessage: isLoggedIn ? 'Welcome back! You are logged in.' : 'Please log in.' }; } render() { return ( <div> <p>{this.state.statusMessage}</p> </div> ); } } class App extends Component { state = { isLoggedIn: false }; handleLoginToggle = () => { this.setState((prevState) => ({ isLoggedIn: !prevState.isLoggedIn })); }; render() { return ( <div className='App'> <h1>Login App</h1> <button onClick={this.handleLoginToggle}> {this.state.isLoggedIn ? 'Logout' : 'Login'} </button> <LoginStatus isLoggedIn={this.state.isLoggedIn} /> </div> ); } } export default App;
输出
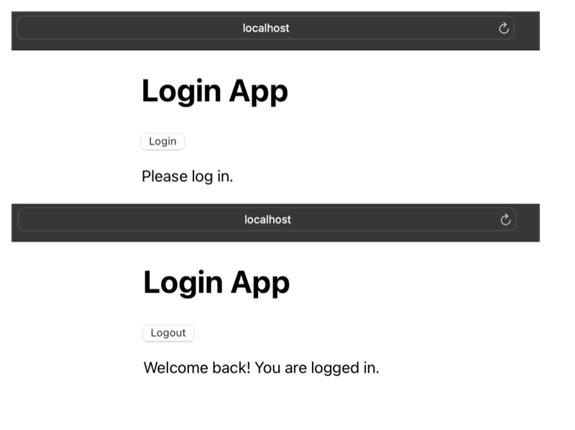
在上面的应用程序中,我们使用了 getDerivedStateFromProps() 方法来检查用户的登录和注销状态。正如我们在上面的输出中看到的,当我们单击该按钮时,屏幕上会显示一个按钮,它将显示消息“欢迎回来!您已登录。”并且按钮更改为注销。
局限性
与以前的 UNSAFE_componentWillReceiveProps 不同,它在每次渲染时都会被调用。
它无法访问组件实例,因此我们不能直接在其中使用它。
总结
getDerivedStateFromProps 函数乍一看可能很难,但它是特定条件下的工具。简单来说,它允许我们根据其 props 的更改来管理组件的状态。