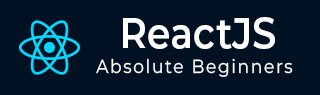
- ReactJS 教程
- ReactJS - 首页
- ReactJS - 简介
- ReactJS - 路线图
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优点与缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用程序
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用新创建的组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性 (props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建一个事件感知组件
- ReactJS - 在 Expense Manager APP 中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React Hooks 进行组件生命周期管理
- ReactJS - 布局组件
- ReactJS - 分页
- ReactJS - Material UI
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 非受控组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - Keys
- ReactJS - 路由
- ReactJS - Redux
- ReactJS - 动画
- ReactJS - Bootstrap
- ReactJS - 地图
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- Hooks
- ReactJS - Hooks 简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义 Hooks
- ReactJS 高级
- ReactJS - 可访问性
- ReactJS - 代码分割
- ReactJS - 上下文
- ReactJS - 错误边界
- ReactJS - 转发 Refs
- ReactJS - 片段
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 优化性能
- ReactJS - Profiler API
- ReactJS - 端口
- ReactJS - 无 ES6 ECMAScript 的 React
- ReactJS - 无 JSX 的 React
- ReactJS - 调和
- ReactJS - Refs 和 DOM
- ReactJS - 渲染 Props
- ReactJS - 静态类型检查
- ReactJS - 严格模式
- ReactJS - Web Components
- 其他概念
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - 走马灯
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
- ReactJS 有用资源
- ReactJS - 快速指南
- ReactJS - 有用资源
- ReactJS - 讨论
ReactJS - 静态 propTypes 属性
我们可以在 React 中使用“propTypes”来定义我们的组件应该接收的属性 (props) 的类型。这确保了只向我们的组件提供正确的信息,使我们的代码更安全。
PropTypes 可以被认为是表达我们的组件期望接收什么类型的信息的一种方式。这相当于告诉我们的组件:“嘿,我期望某种类型的数据,所以确保我们得到它!”
换句话说,我们可以说 PropTypes 是 React 中用于对传递给 React 组件的 props 进行类型检查的一种方法。它通过为每个参数定义预期类型来帮助开发人员检测常见问题。propTypes 属性作为静态属性添加到 React 组件类中,这意味着它与类相关联,而不是与类的实例相关联。
导入 propTypes
import PropTypes from 'prop-types';
示例
示例 1
因此,我们正在创建一个小型 React 应用程序,使用静态 propTypes 来定义我们的组件将需要的 props 类型。让我们创建一个基本的应用程序,它接受我们的姓名并进行问候。
import React from 'react'; import PropTypes from 'prop-types'; class Greeting extends React.Component { // Define propTypes static propTypes = { name: PropTypes.string.isRequired, // 'isRequired' means this prop is mandatory }; render() { return ( <div> <h1>Hello, {this.props.name}!</h1> </div> ); } } class App extends React.Component { constructor(props) { super(props); this.state = { userName: '', }; } handleNameChange = (event) => { this.setState({ userName: event.target.value }); }; render() { return ( <div> <h1>Simple Greeting App</h1> <label> Enter your name: <input type="text" value={this.state.userName} onChange={this.handleNameChange} /> </label> {/* Render the Greeting component and pass the name as a prop */} <Greeting name={this.state.userName} /> </div> ); } } export default App;
输出
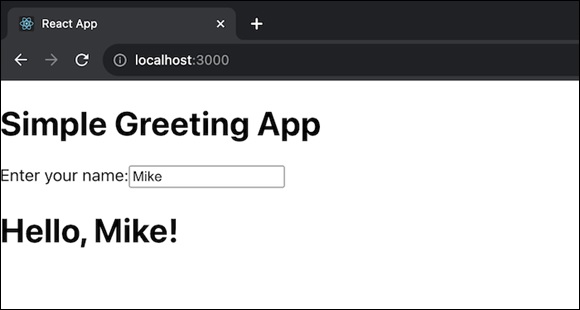
在这个示例中,我们有两个组件:Greeting 和 App。Greeting 组件需要一个名为 name 的字符串类型 prop,它被标记为 isRequired,表示它必须被提供。
App 组件是一个简单的应用程序,我们可以在其中将我们的姓名输入到输入框中。然后,给定的姓名作为 prop 发送到 Greeting 组件,Greeting 组件显示一个问候消息。
在启动此应用程序之前,请记住设置我们的 React 环境并安装必要的包,包括 prop-types。
示例 2
让我们再创建一个小型 React 应用程序,它使用静态 propTypes。这次让我们创建一个 Counter 应用程序。Counter 组件将显示一个数字作为 prop。如果未指定数字,则将其设置为 0。
import React from 'react'; import PropTypes from 'prop-types'; class Counter extends React.Component { // Define propTypes for the Counter component static propTypes = { number: PropTypes.number.isRequired, }; render() { return ( <div> <h2>Counter: {this.props.number}</h2> </div> ); } } class CounterApp extends React.Component { constructor(props) { super(props); this.state = { count: 0, }; } handleIncrement = () => { this.setState((prevState) => ({ count: prevState.count + 1 })); }; handleDecrement = () => { this.setState((prevState) => ({ count: prevState.count - 1 })); }; render() { return ( <div> <h1>Simple Counter App</h1> {/* Render the Counter component and pass the count as a prop */} <Counter number={this.state.count} /> <button onClick={this.handleIncrement}>Increment</button> <button onClick={this.handleDecrement}>Decrement</button> </div> ); } } export default CounterApp;
输出
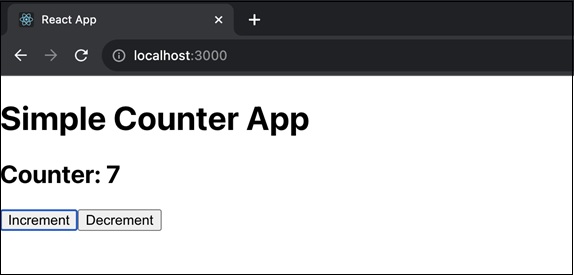
在这个应用程序中,我们创建了一个 Counter 组件,其中包含 number prop 的 propTypes。CounterApp 组件在其状态中维护一个计数以及用于增加和减少计数的按钮。Counter 组件显示当前计数。
示例 3
让我们再创建一个小型 React 应用程序,它使用静态 propTypes。这次,我们将创建一个 ColorBox 组件,它将颜色作为 prop 并显示一个彩色框。
import React from 'react'; import PropTypes from 'prop-types'; class ColorBox extends React.Component { // Define propTypes for the ColorBox component static propTypes = { color: PropTypes.string.isRequired, }; render() { const boxStyle = { width: '100px', height: '100px', backgroundColor: this.props.color, }; return ( <div> <h2>Color Box</h2> <div style={boxStyle}></div> </div> ); } } class ColorBoxApp extends React.Component { constructor(props) { super(props); this.state = { selectedColor: 'blue', }; } handleColorChange = (event) => { this.setState({ selectedColor: event.target.value }); }; render() { return ( <div> <h1>Color Box App</h1> <label> Select a color: <select value={this.state.selectedColor} onChange={this.handleColorChange}> <option value="red">Red</option> <option value="green">Green</option> <option value="blue">Blue</option> </select> </label> <ColorBox color={this.state.selectedColor} /> </div> ); } } export default ColorBoxApp;
输出
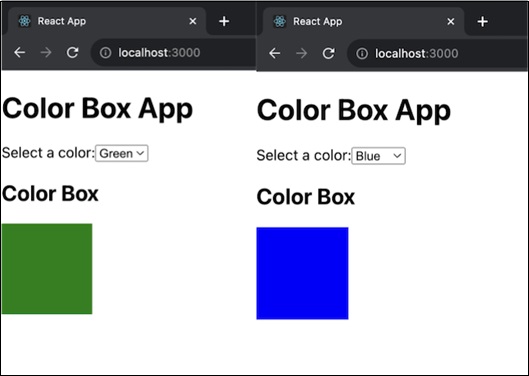
在这个应用程序中,ColorBox 组件对 color prop 有 propTypes 要求。ColorBoxApp 组件在其状态中维护一个选定的颜色并提供颜色选择下拉菜单。然后使用 ColorBox 组件根据已选颜色显示彩色框。
总结
PropTypes 是 React 的一项功能,它允许我们定义组件将接收的属性 (props) 的预期类型。它通过允许开发人员在开发之前指定 props 的数据类型来帮助检测潜在的问题。