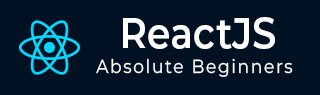
- ReactJS 教程
- ReactJS - 首页
- ReactJS - 简介
- ReactJS - 路线图
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优点与缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用新创建的组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性 (props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建一个事件感知组件
- ReactJS - 在 Expense Manager 应用中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React Hooks 进行组件生命周期管理
- ReactJS - 布局组件
- ReactJS - 分页
- ReactJS - Material UI
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 非受控组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - Keys
- ReactJS - 路由
- ReactJS - Redux
- ReactJS - 动画
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- Hooks
- ReactJS - Hooks 简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义 Hooks
- ReactJS 高级
- ReactJS - 可访问性
- ReactJS - 代码分割
- ReactJS - Context
- ReactJS - 错误边界
- ReactJS - 转发 Refs
- ReactJS - Fragments
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 性能优化
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - 无 ES6 ECMAScript 的 React
- ReactJS - 无 JSX 的 React
- ReactJS - Reconciliation
- ReactJS - Refs 和 DOM
- ReactJS - Render Props
- ReactJS - 静态类型检查
- ReactJS - Strict Mode
- ReactJS - Web Components
- 其他概念
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 行内样式
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - 走马灯
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
- ReactJS 有用资源
- ReactJS - 快速指南
- ReactJS - 有用资源
- ReactJS - 讨论
ReactJS - testInstance.findAllByProps() 方法
testInstance.findAllByProps() 方法用于查找所有具有某些属性的后代测试实例。简单来说,它可以帮助我们定位并获取测试中与特定特征匹配的所有元素。
如果我们想更具体地查找具有特定属性的实例,可以使用 testInstance.findAllByProps(props)。这意味着我们正在搜索具有指定属性(属性或特征)的测试实例。此方法是根据其属性查找和收集测试中元素的工具。它就像一个用于测试组件的搜索函数。
语法
testInstance.findAllByProps(props)
参数
props − 这是我们提供给函数的输入。它显示了我们想要在测试实例中查找的属性或特征。这些可以是我们正在查找的特定属性或特征。
返回值
该函数将返回与特定属性匹配的测试实例集合。它为我们提供了一个具有我们正在搜索的特征的测试元素列表。
示例
示例 - 带有 Props 的基本组件
我们将创建一个简单的 React 组件及其对应的测试文件。此文件定义了一个名为 SimpleComponent 的基本 React 组件。它接受一个 prop text 并将其渲染在 div 元素内。在测试文件中,编写了一个测试用例以确保 SimpleComponent 正确渲染提供的文本。它使用 findAllByProps 查找具有特定 props 的元素。因此,此应用程序的代码如下所示:
SimpleComponent.js
import React from 'react'; const SimpleComponent = ({ text }) => { return <div>{text}</div>; }; export default SimpleComponent;
SimpleComponent.test.js
import React from 'react'; import { create } from 'react-test-renderer'; import SimpleComponent from './SimpleComponent'; test('renders text correctly', () => { const component = create(<SimpleComponent text="Hello, World!" />); const instance = component.root; const elements = instance.findAllByProps({ text: 'Hello, World!' }); expect(elements.length).toBe(1); });
输出
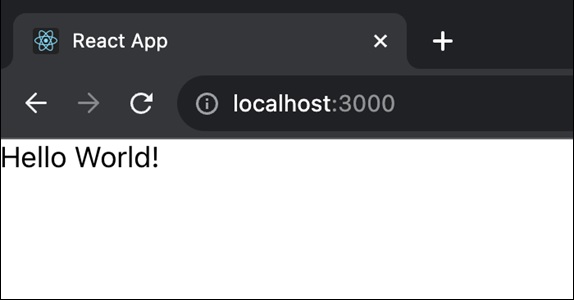
示例 - 带有 props 的列表组件
现在让我们创建一个 ListComponent 及其对应的测试文件。在此文件中,将定义一个名为 ListComponent 的 React 组件。它将接受一个 prop items 并渲染一个无序列表,其中包含数组中每个项目的列表项。此测试文件包含一个测试用例,用于检查 ListComponent 是否正确渲染列表项。此示例的代码如下所示:
ListComponent.js
import React from 'react'; const ListComponent = ({ items }) => { return ( <ul> {items.map((item, index) => ( <li key={index}>{item}</li> ))} </ul> ); }; export default ListComponent;
ListComponent.test.js
import React from 'react'; import { create } from 'react-test-renderer'; import ListComponent from './ListComponent'; test('renders list items correctly', () => { const items = ['Item 1', 'Item 2', 'Item 3']; const component = create(<ListComponent items={items} />); const instance = component.root; const elements = instance.findAllByProps({ children: items }); expect(elements.length).toBe(items.length); });
输出
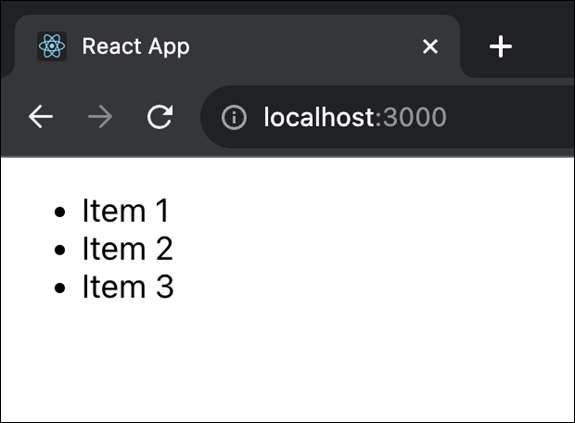
示例 - 条件渲染组件
在这个 React 应用中,我们将创建一个简单的 ToggleComponent,它将有一个按钮来切换段落的可见性。该组件还将有一个对应的测试文件 ToggleComponent.test.js,它使用 react-test-renderer 库来测试条件渲染行为。
ToggleComponent.js
import React, { useState } from 'react'; const ToggleComponent = () => { const [isToggled, setToggle] = useState(false); return ( <div> <button onClick={() => setToggle(!isToggled)}>Toggle</button> {isToggled && <p>This is visible when toggled!</p>} </div> ); }; export default ToggleComponent;
ToggleComponent.test.js
import React from 'react'; import { create } from 'react-test-renderer'; import ToggleComponent from './ToggleComponent'; test('renders paragraph conditionally', () => { // Create a test instance const component = create(<ToggleComponent />); const instance = component.root; // Find elements in the component with props const elements = instance.findAllByProps({ children: 'This is visible when toggled!' }); expect(elements.length).toBe(0); instance.findByType('button').props.onClick(); const updatedElements = instance.findAllByProps({ children: 'This is visible when toggled!' }); expect(updatedElements.length).toBe(1); });
输出
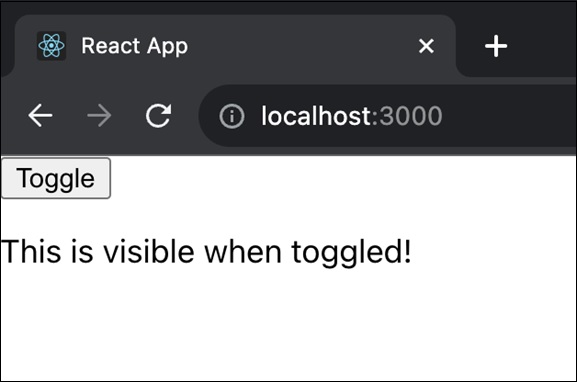
此示例显示了一种测试具有条件渲染的 React 组件的简单方法。测试用例确保段落最初不可见,并在单击按钮时可见。
总结
testInstance.findAllByProps() 函数通常用于 React 的测试库中,根据其属性查找和与组件交互。此函数使测试和验证应用程序中的特定行为更加容易。此函数用于查找所有具有特定属性的后代测试实例。因此,我们已经看到了可以使用此方法测试我们的应用程序的不同场景。