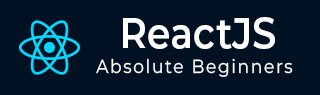
- ReactJS 教程
- ReactJS - 首页
- ReactJS - 简介
- ReactJS - 路线图
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优点与缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用新创建的组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性 (props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建一个事件感知组件
- ReactJS - 在 Expense Manager 应用中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React Hooks 进行组件生命周期管理
- ReactJS - 布局组件
- ReactJS - 分页
- ReactJS - Material UI
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 非受控组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - Keys
- ReactJS - 路由
- ReactJS - Redux
- ReactJS - 动画
- ReactJS - Bootstrap
- ReactJS - 地图
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- Hooks
- ReactJS - Hooks 简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义 Hooks
- ReactJS 高级
- ReactJS - 可访问性
- ReactJS - 代码分割
- ReactJS - 上下文
- ReactJS - 错误边界
- ReactJS - 转发 Refs
- ReactJS - 碎片
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 优化性能
- ReactJS - Profiler API
- ReactJS - 端口
- ReactJS - 无 ES6 ECMAScript 的 React
- ReactJS - 无 JSX 的 React
- ReactJS - 调和
- ReactJS - Refs 和 DOM
- ReactJS - 渲染 Props
- ReactJS - 静态类型检查
- ReactJS - 严格模式
- ReactJS - Web Components
- 其他概念
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - 走马灯
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
- ReactJS 有用资源
- ReactJS - 快速指南
- ReactJS - 有用资源
- ReactJS - 讨论
ReactJS - testInstance.findAllByType() 方法
在软件测试中,我们经常需要根据类型查找特定的测试实例。testInstance.findAllByType() 方法可以帮助我们做到这一点。此函数主要用于查找所有测试实例,而无需指定特定类型。
它搜索并返回所有后代测试实例,而不管它们的类型是什么。如果我们心中有一个特定的类型,我们可以使用此函数。它有助于查找并返回所有具有指定类型的测试实例。
这些方法使软件开发人员和测试人员能够更轻松地根据类型查找和处理测试实例。
语法
testInstance.findAllByType(type)
参数
type − 这是我们在调用 findAllByType 方法时提供的参数。它显示了我们想要查找的测试实例的特定类型。我们需要将 type 替换为我们正在查找的实际类型。
返回值
此方法返回与指定类型匹配的测试实例集合。该集合包含具有给定类型的所有后代测试实例。
示例
所以现在我们将创建不同的应用程序及其测试用例,以更好地理解 testInstance.findAllByType() 方法。
示例 - 基本组件搜索
假设我们有一个简单的 Button 组件需要测试。现在,我们可以在主 App 组件中使用 Button 组件。请确保根据项目结构调整 Button 组件的路径。此示例演示了如何以简单的方式在测试中使用 findAllByType 来测试 Button 组件。
Button.js
import React from 'react'; const Button = ({ label }) => { return ( <button>{label}</button> ); }; export default Button;
App.js
import React from 'react'; import { create } from 'react-test-renderer'; import Button from './Button'; const App = () => { // Create a test renderer instance for the component const testRenderer = create(<Button label="Click me" />); // Get the root test instance const testInstance = testRenderer.root; // Now we can use findAllByType const foundInstances = testInstance.findAllByType('button'); return ( <div> <h1>Basic Component Search</h1> <p>Found {foundInstances.length} instances of Button component.</p> </div> ); }; export default App;
输出
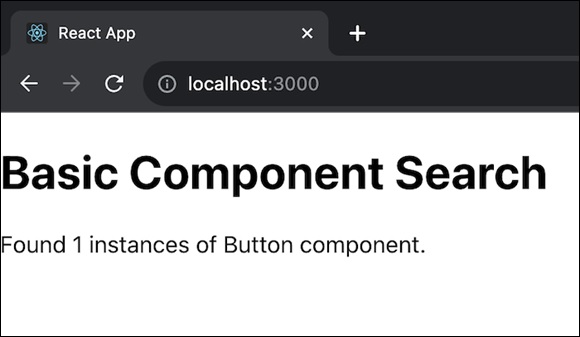
正如我们在上面的输出图像中看到的。有一条消息可见,其中写着我们在应用程序中找到一个按钮组件。这是一个基本的组件搜索操作,我们可以使用 findAllByType() 方法。
示例 - 自定义组件搜索
假设我们有一个名为 CustomComponent 的简单自定义组件。现在我们可以在我们的 App 组件中使用此 CustomComponent。请确保根据项目结构调整 CustomComponent 组件的路径。此示例演示了如何以简单的方式使用 findAllByType 来测试 CustomComponent。
CustomComponent.js
import React from 'react'; const CustomComponent = ({ text }) => { return ( <div> <p>{text}</p> </div> ); }; export default CustomComponent;
App.js
import React from 'react'; import { create } from 'react-test-renderer'; import CustomComponent from './CustomComponent'; const App = () => { // Create a test renderer instance for the component const testRenderer = create(<CustomComponent text="Hello, Custom Component!" />); // Get the root test instance const testInstance = testRenderer.root; // Now we can use findAllByType const customComponentType = CustomComponent; const foundInstances = testInstance.findAllByType((node) => node.type === customComponentType); return ( <div> <h1>Custom Component Search</h1> <p>Found {foundInstances.length} instances of CustomComponent component.</p> </div> ); }; export default App;
输出
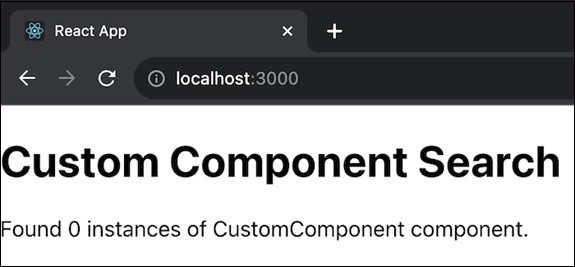
实际输出将根据测试配置而有所不同,这只是一个示例。这些测试的主要目标是确保组件按预期工作并且测试断言有效。
示例 - 动态组件搜索
下面是另一个使用 testInstance.findAllByType(type) 的 React 应用示例。此应用程序呈现项目列表,并允许我们动态搜索特定项目类型。ItemList 组件呈现项目列表,而 App 组件动态更改项目类型并根据所选项目类型记录找到的实例数量。
ItemList.js
import React from 'react'; const ItemList = ({ items }) => { return ( <div> <h2>Item List</h2> <ul> {items.map((item, index) => ( <li key={index}>{item}</li> ))} </ul> </div> ); }; export default ItemList;
App.js
import React, { useState, useEffect } from 'react'; import { create } from 'react-test-renderer'; import ItemList from './ItemList'; const App = () => { const [itemType, setItemType] = useState('Fruit'); useEffect(() => { // Creating a test renderer instance const testRenderer = create(<ItemList items={['Apple', 'Banana', 'Orange']} />); const testInstance = testRenderer.root; // Finding instances dynamically const foundInstances = testInstance.findAllByType('li'); console.log(`Found ${foundInstances.length} instances of ${itemType} in the list.`); }, [itemType]); return ( <div> <h1>Dynamic Item Search</h1> <p>Change item type dynamically:</p> <button onClick={() => setItemType('Fruit')}>Show Fruits</button> <button onClick={() => setItemType('Vegetable')}>Show Vegetables</button> </div> ); }; export default App;
输出
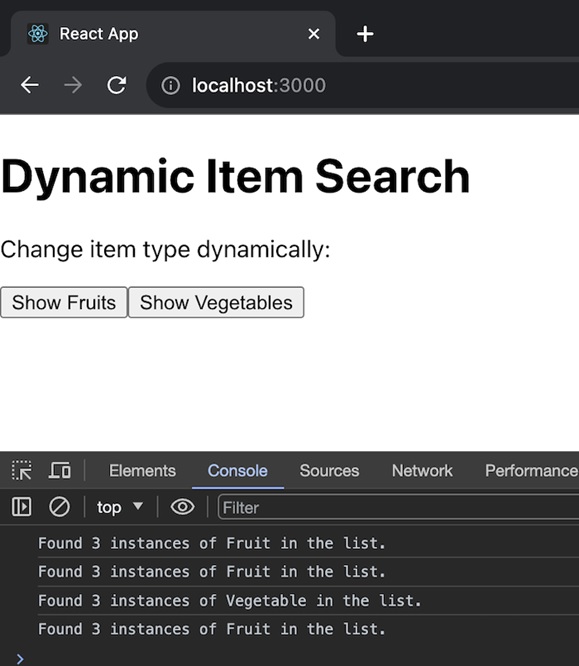
总结
总的来说,我们可以说当我们使用 findAllByType(type) 时,我们需要告诉它我们感兴趣的测试实例的类型(type 参数),作为回报,它会给我们一组这些特定的测试实例。并且我们根据不同的情况(如基本组件搜索、自定义组件搜索和动态组件搜索)创建了三个不同的应用程序。您可以根据您的具体需求使用此函数。