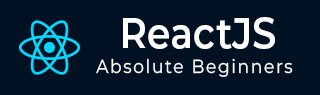
- ReactJS 教程
- ReactJS - 首页
- ReactJS - 简介
- ReactJS - 路线图
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优点与缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用新创建的组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性 (props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建一个事件感知组件
- ReactJS - 在 Expense Manager APP 中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React Hooks 进行组件生命周期管理
- ReactJS - 布局组件
- ReactJS - 分页
- ReactJS - Material UI
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 非受控组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - 键
- ReactJS - 路由
- ReactJS - Redux
- ReactJS - 动画
- ReactJS - Bootstrap
- ReactJS - 地图
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- Hooks
- ReactJS - Hooks 简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义 Hooks
- ReactJS 高级
- ReactJS - 可访问性
- ReactJS - 代码分割
- ReactJS - 上下文
- ReactJS - 错误边界
- ReactJS - 转发 Refs
- ReactJS - 片段
- ReactJS - 高阶组件
- ReactJS - 集成其他库
- ReactJS - 优化性能
- ReactJS - Profiler API
- ReactJS - 端口
- ReactJS - 无 ES6 ECMAScript 的 React
- ReactJS - 无 JSX 的 React
- ReactJS - 调和
- ReactJS - Refs 和 DOM
- ReactJS - 渲染 Props
- ReactJS - 静态类型检查
- ReactJS - 严格模式
- ReactJS - Web Components
- 其他概念
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - 走马灯
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
- ReactJS 有用资源
- ReactJS - 快速指南
- ReactJS - 有用资源
- ReactJS - 讨论
ReactJS - testInstance.findByProps() 方法
testInstance.findByProps(props) 是一个测试函数。testInstance 最有可能指的是软件测试环境中的测试实例或对象。findByProps(props) 是一个组件,这一事实表明我们正在测试实例中搜索特定的内容。“props”指的是属性,即我们正在查找的项目的属性。
此代码查找具有特定属性的单个测试对象。这就像在许多物品中寻找特定物品。如果只有一个匹配项,则返回该实例。否则,将显示错误消息。
语法
testInstance.findByProps(props)
参数
props - “props”指的是属性,即我们正在查找的项目的属性。
返回值
此代码查找具有特定特征的单个测试对象。这就像查找一个对象。如果只有一个匹配项,则返回该实例。如果不是这种情况,则会显示错误消息。
示例
示例 - 基本组件测试
使用 testInstance.findByProps(props) 方法创建 React 应用涉及编写测试用例,以在我们的 React 组件中查找具有某些属性的特定元素。以下是此应用的简单代码 -
MyComponent.js
import React from 'react'; const MyComponent = ({ title }) => ( <div> <h1>{title}</h1> <p>Hello, this is a simple component.</p> </div> ); export default MyComponent;
MyComponent.test.js
import React from 'react'; import { render } from '@testing-library/react'; import MyComponent from './MyComponent'; test('finds the title using findByProps', () => { const { findByProps } = render(<MyComponent title="My App" />); const titleElement = findByProps({ title: 'My App' }); expect(titleElement).toBeInTheDocument(); });
输出
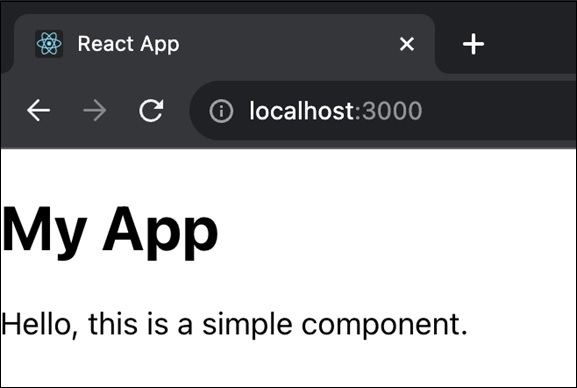
示例 - 列表组件测试
现在我们将创建一个名为 ListComponent 的 React 组件。它接收一个 prop item,它是一个数组。它渲染一个无序列表 (<ul>),其中列表项 (<li>) 由 items 数组的元素生成。
然后我们将为 ListComponent 组件创建 ListComponent.test.js。它使用来自 @testing-library/react 库的 render 函数来渲染具有 prop items 的 ListComponent。测试函数正在检查列表项是否包含文本“Item 2”,该项是否存在于使用 findByProps 渲染的组件中。
ListComponent.js
import React from 'react'; const ListComponent = ({ items }) => ( <ul> {items.map((item, index) => ( <li key={index}>{item}</li> ))} </ul> ); export default ListComponent;
ListComponent.test.js
import React from 'react'; import { render } from '@testing-library/react'; import ListComponent from './ListComponent'; test('finds the list item using findByProps', () => { const items = ['Item 1', 'Item 2', 'Item 3']; const { findByProps } = render(<ListComponent items={items} />); const listItem = findByProps({ children: 'Item 2' }); expect(listItem).toBeInTheDocument(); });
输出
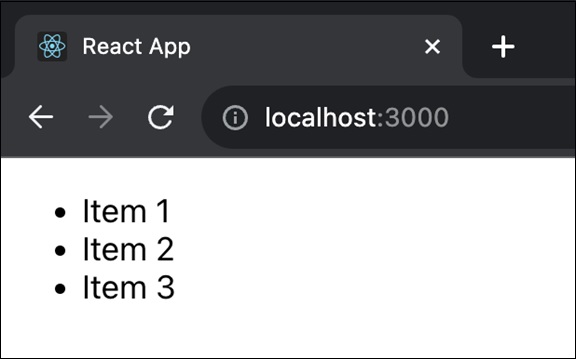
此代码测试了具有项目列表的 ListComponent 的渲染,并检查特定列表项(“Item 2”)是否存在于组件中,并借助 findByProps 方法。
示例 - 表单组件测试
这次我们将创建一个名为 FormComponent 的 React 组件。它包含一个带有输入字段的表单。输入字段使用 useState hook 进行控制以管理 inputValue。标签具有 htmlFor 属性,输入字段使用相同的 id。
然后我们将为 FormComponent 组件创建一个测试文件 (FormComponent.test.js)。测试检查输入字段是否以空值 (“”) 开始,并断言它最初存在于文档中。fireEvent.change 模拟用户在输入字段中键入“Hello, World!”。然后它使用 findByProps 搜索具有更新值的输入字段,并断言它存在于文档中。
FormComponent.js
import React, { useState } from 'react'; const FormComponent = () => { const [inputValue, setInputValue] = useState(''); return ( <form> <label htmlFor="inputField">Type something:</label> <input type="text" id="inputField" value={inputValue} onChange={(e) => setInputValue(e.target.value)} /> </form> ); }; export default FormComponent;
FormComponent.test.js
import React from 'react'; import { render, fireEvent } from '@testing-library/react'; import FormComponent from './FormComponent'; test('finds the input value using findByProps', async () => { const { findByProps } = render(<FormComponent />); const inputElement = findByProps({ value: '' }); expect(inputElement).toBeInTheDocument(); fireEvent.change(inputElement, { target: { value: 'Hello, World!' } }); const updatedInputElement = findByProps({ value: 'Hello, World!' }); expect(updatedInputElement).toBeInTheDocument(); });
此代码通过检查当用户在其中键入时输入字段是否正确更新来测试 FormComponent 的渲染和用户交互。
总结
因此,testInstance.findByProps(props) 帮助我们在测试期间查找具有特定属性的对象。如果它找到完全一个匹配项,则测试成功。否则,将发送错误。我们已经看到了可以使用此函数来测试 React 应用的不同应用和案例。