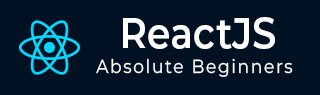
- ReactJS 教程
- ReactJS - 首页
- ReactJS - 简介
- ReactJS - 路线图
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优点与缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用新创建的组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性 (props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建一个事件感知组件
- ReactJS - 在 Expense Manager APP 中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React Hooks 进行组件生命周期管理
- ReactJS - 布局组件
- ReactJS - 分页
- ReactJS - Material UI
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 非受控组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - Keys
- ReactJS - 路由
- ReactJS - Redux
- ReactJS - 动画
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- Hooks
- ReactJS - Hooks 简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义 Hooks
- ReactJS 高级
- ReactJS - 可访问性
- ReactJS - 代码分割
- ReactJS - Context
- ReactJS - 错误边界
- ReactJS - 转发 Refs
- ReactJS - Fragments
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 性能优化
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - 无 ES6 ECMAScript 的 React
- ReactJS - 无 JSX 的 React
- ReactJS - Reconciliation (协调)
- ReactJS - Refs 和 DOM
- ReactJS - Render Props
- ReactJS - 静态类型检查
- ReactJS - Strict Mode (严格模式)
- ReactJS - Web Components
- 其他概念
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - 轮播图
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
- ReactJS 有用资源
- ReactJS - 快速指南
- ReactJS - 有用资源
- ReactJS - 讨论
ReactJS - testInstance.findByType() 方法
测试就像编程世界中的英雄。它用于检查我们的代码是否按预期运行。findByType() 函数在此过程中是一个有用的工具。让我们来研究一下这个函数以及它如何帮助我们。
findByType() 是一个命令,它帮助我们在测试组中找到特定的测试实例。它就像有一个指导者,指导我们进行我们想要进行的准确测试。
findByType() 是一个用于测试的有用工具。此函数可以帮助我们浏览复杂的测试,确保我们的测试过程既高效又实用。
一个更集中的方法是 testInstance.findByType(type)。它将搜索范围限制为单一类型的测试。将“type”定义为一个类别或标签,这有助于我们确定测试的性质。
语法
testInstance.findByType(type)
参数
type − ‘type’ 根据测试的目的或用途定义测试。例如,我们可能有验证数字的测试,也有检查字符串的测试,等等。通过指定一个 type,findByType() 可以专注于包含在给定类别中的测试。
返回值
当我们调用 testInstance.findByType(type) 时,该函数将返回一个与我们提供的 type 匹配的单个后代测试实例。
示例
现在我们将使用 testInstance.findByType(type) 方法创建不同的 React 应用,以展示此函数如何在各种场景中使用。
示例 - 基本组件搜索
假设我们有一个包含各种组件的 React 应用,并且我们想使用 testInstance.findByType(type) 查找特定组件。
App.js
import React from 'react'; import ComponentA from './ComponentA'; function App() { return ( <div> <h1>React App</h1> <ComponentA /> </div> ); } export default App;
ComponentA.js
import React from 'react'; const ComponentA = () => { return ( <div> <p>This is Component A</p> </div> ); } export default ComponentA;
App.test.js
import React from 'react'; import { render } from '@testing-library/react'; import App from './App'; test('Find ComponentA using findByType', () => { const { findByType } = render(<App />); const componentA = findByType('ComponentA'); // perform further testing expect(componentA).toBeInTheDocument(); });
输出
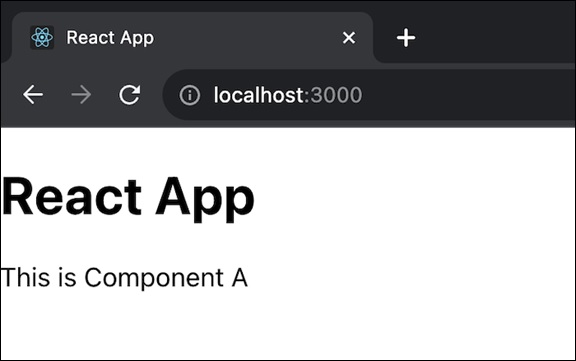
此示例展示了一个基本组件搜索场景,其中 testInstance.findByType(type) 可用于测试 React 应用程序。
示例 - 动态组件渲染
在这种情况下,让我们想象一个 React 应用,其中组件根据用户交互动态渲染。因此,此应用及其相应测试用例的代码如下:
App.js
import React, { useState } from 'react'; import DynamicComponent from './components/DynamicComponent'; function App() { const [showComponent, setShowComponent] = useState(true); return ( <div> <h1>React App Example 2</h1> {showComponent && <DynamicComponent />} </div> ); } export default App;
DynamicComponent.js
import React from 'react'; const DynamicComponent = () => { return ( <div> <p>This is a Dynamic Component</p> </div> ); } export default DynamicComponent;
App.test.js
import React from 'react'; import { render } from '@testing-library/react'; import App from './App'; test('Find DynamicComponent using findByType', () => { const { findByType } = render(<App />); const dynamicComponent = findByType('DynamicComponent'); // perform further testing expect(dynamicComponent).toBeInTheDocument(); });
输出
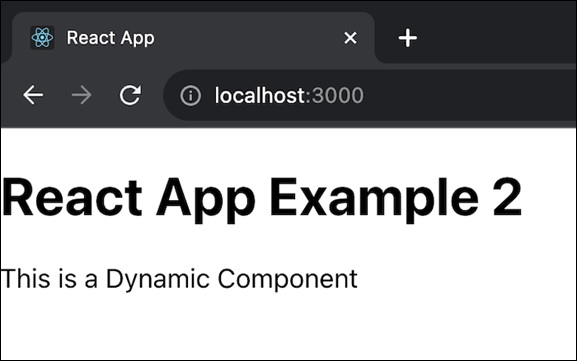
此示例展示了一个动态组件渲染场景,其中 testInstance.findByType(type) 可用于测试 React 应用程序。
示例 - 带有错误处理的条件渲染
在此示例中,我们将探索一个使用 testInstance.findByType(type) 进行条件渲染和错误处理的 React 应用。因此,此应用及其测试文件的代码如下:
App.js
import React, { useState } from 'react'; import ErrorBoundary from './components/ErrorBoundary'; function App() { const [hasError, setHasError] = useState(false); const triggerError = () => { setHasError(true); }; return ( <div> <h1>React App Example 3</h1> <ErrorBoundary hasError={hasError} /> <button onClick={triggerError}>Trigger Error</button> </div> ); } export default App;
ErrorBoundary.js
import React from 'react'; class ErrorBoundary extends React.Component { componentDidCatch(error, info) { // Handle the error console.error('Error caught:', error, info); } render() { if (this.props.hasError) { // Render fallback UI in case of an error return <p>Something went wrong!</p>; } return this.props.children; } } export default ErrorBoundary;
App.test.js
import React from 'react'; import { render } from '@testing-library/react'; import App from './App'; test('Find ErrorBoundary using findByType', () => { const { findByType } = render(<App />); const errorBoundary = findByType('ErrorBoundary'); // perform further testing expect(errorBoundary).toBeInTheDocument(); });
输出
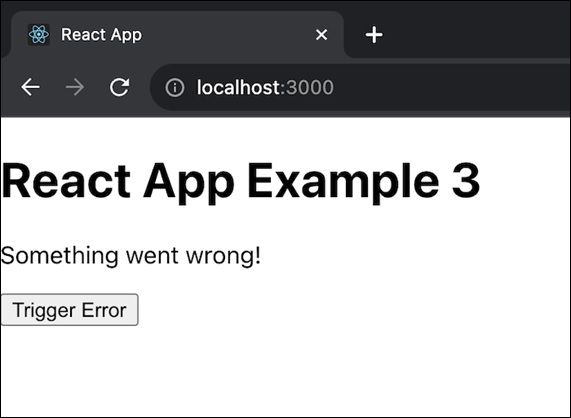
此示例展示了一个带有错误处理的条件渲染场景,其中 testInstance.findByType(type) 可用于测试 React 应用程序。
总结
testInstance.findByType(type) 将搜索范围限制为特定类型的测试。此方法的结果返回提供的类型的特定测试实例(假设存在唯一匹配)。如果出现任何错误,它将向我们显示错误消息,提示我们检查并修改我们的请求或验证测试的组织方式。
请记住,该函数力求准确,其返回值取决于获得给定类型的清晰、唯一的匹配。