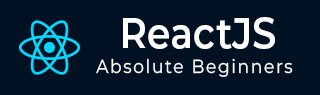
- ReactJS 教程
- ReactJS - 首页
- ReactJS - 简介
- ReactJS - 路线图
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优点和缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用新创建的组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性 (props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建一个事件感知组件
- ReactJS - 在 Expense Manager 应用中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React Hooks 进行组件生命周期管理
- ReactJS - 布局组件
- ReactJS - 分页
- ReactJS - Material UI
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 非受控组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - Keys
- ReactJS - 路由
- ReactJS - Redux
- ReactJS - 动画
- ReactJS - Bootstrap
- ReactJS - Map
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- Hooks
- ReactJS - Hooks 简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义 Hooks
- ReactJS 高级
- ReactJS - 可访问性
- ReactJS - 代码分割
- ReactJS - Context
- ReactJS - 错误边界
- ReactJS - 转发 Refs
- ReactJS - 片段
- ReactJS - 高阶组件
- ReactJS - 集成其他库
- ReactJS - 优化性能
- ReactJS - Profiler API
- ReactJS - Portals
- ReactJS - 无 ES6 ECMAScript 的 React
- ReactJS - 无 JSX 的 React
- ReactJS - 调和
- ReactJS - Refs 和 DOM
- ReactJS - 渲染 Props
- ReactJS - 静态类型检查
- ReactJS - 严格模式
- ReactJS - Web Components
- 其他概念
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - PropTypes
- ReactJS - BrowserRouter
- ReactJS - DOM
- ReactJS - 轮播图
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
- ReactJS 有用资源
- ReactJS - 快速指南
- ReactJS - 有用资源
- ReactJS - 讨论
ReactJS - testInstance.props 属性
在网页开发和创建用户界面时,有一个名为 testInstance.props 的概念。可以将其视为网站上某个特定元素的一组指令。
Props 是 Web 应用中父组件发送给子组件的消息。假设我们在网站上有一个按钮,并希望告诉它要小一些。在编程术语中,我们会说类似于 <Button size="small" />。在这种情况下,Size 是一个 prop,其值为 'small'。
当我们遇到 testInstance.props 时,就像我们在测试期间查看发送到网站某些部分的所有消息或指令。例如,如果我们有一个 <Button size="small" /> 组件,则其 testInstance.props 将为 {size:'small'}。它主要由这些消息或特征组成。
语法
testInstance.props
参数
testInstance.props 不接受任何参数。
返回值
testInstance.props 属性在测试期间返回与 Web 应用中特定元素相关联的一组指令。
示例
示例 - 按钮组件
这是一个名为 Button 的简单 React 组件。它旨在创建一个具有可自定义属性(如字体大小、背景颜色和标签)的按钮。该组件在测试期间记录其属性以进行调试。如果未通过 props 提供特定的样式或标签,则使用默认值以确保按钮在视觉上具有吸引力。因此,此应用程序的代码如下所示:
import React from 'react'; const Button = (props) => { const { fontSize, backgroundColor, label } = props; console.log('Button Props:', props); const buttonStyle = { fontSize: fontSize || '16px', backgroundColor: backgroundColor || 'blue', padding: '10px 15px', borderRadius: '5px', cursor: 'pointer', color: '#fff', // Text color }; const buttonLabel = label || 'Click me'; return ( <button style={buttonStyle}> {buttonLabel} </button> ); }; export default Button;
输出
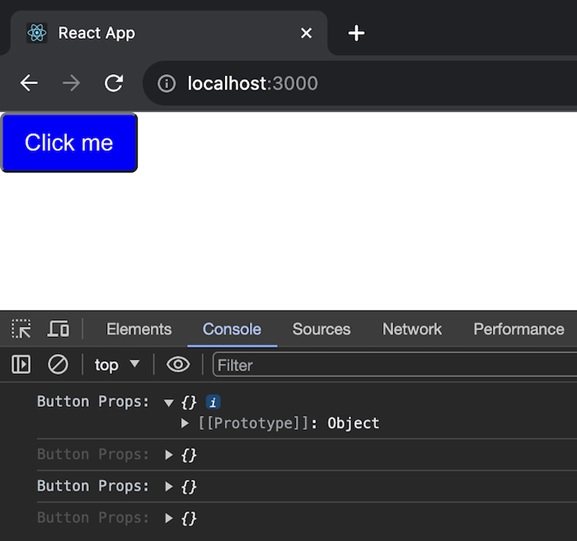
示例 - 图像组件
在此示例中,App 组件使用一组图像 props(如 src、alt 和 width)呈现 Image 组件。我们可以用所需图像的实际 URL、替代文本和宽度替换占位符值。Image 组件中的 console.log 语句将在测试期间记录这些 props。因此,代码如下:
Image.js
import React from 'react'; const Image = (props) => { const { src, alt, width } = props; console.log('Image Props:', props); // Log the props here return <img src={src} alt={alt} style={{ width }} />; }; export default Image;
App.js
import React from 'react'; import Image from './Image'; const App = () => { const imageProps = { src: 'https://www.example.com/picphoto.jpg', // Replace with the actual image URL alt: 'Sample Image', width: '300px', }; return ( <div> <h1>Image Component Example</h1> <Image {...imageProps} /> </div> ); }; export default App;
输出
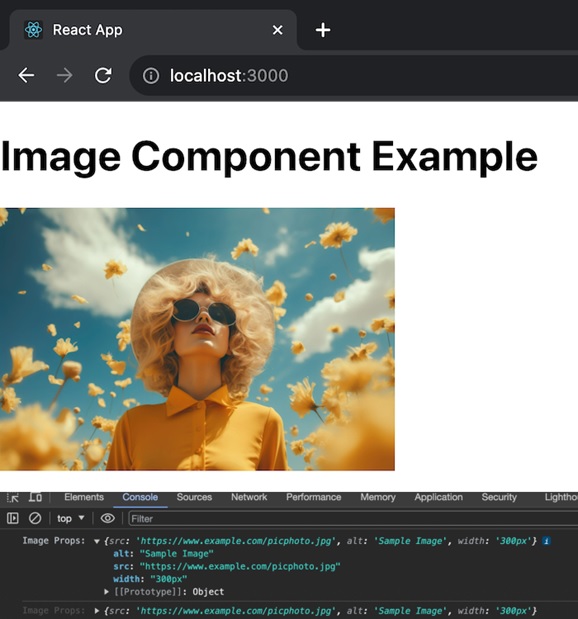
示例 - 输入组件
在此示例中,App 组件使用一组输入 props(如 placeholder 和 maxLength)呈现 Input 组件。Input 组件中的 console.log 语句将在测试期间记录这些 props。并且此组件的代码如下所示:
Input.js
import React from 'react'; const Input = (props) => { const { placeholder, maxLength } = props; console.log('Input Props:', props); // Log the props here return <input type="text" placeholder={placeholder} maxLength={maxLength} />; }; export default Input;
App.js
import React from 'react'; import Input from './Input'; const App = () => { const inputProps = { placeholder: 'Enter your text', maxLength: 50, }; return ( <div> <h1>Input Component Example</h1> <Input {...inputProps} /> </div> ); }; export default App;
输出
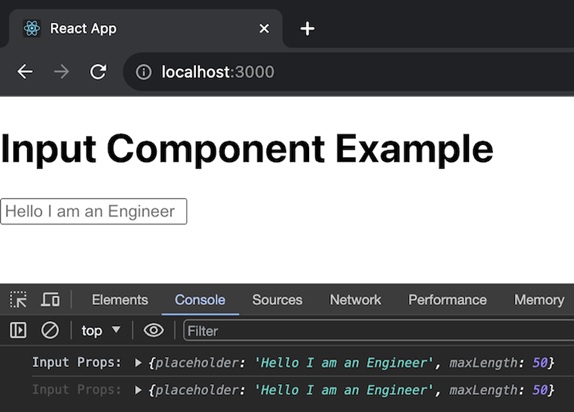
总结
testInstance.props 是一个属性,允许开发人员在测试期间查看和理解分配给网站上某些元素的属性或指令。这就像查看一张解释 Web 应用的每个组件应如何工作的备忘单。通过理解此原理,开发人员可以确保其网站正常运行并为用户提供出色的体验。